Block Rogue Code: How to Prevent System.exit() Misuse
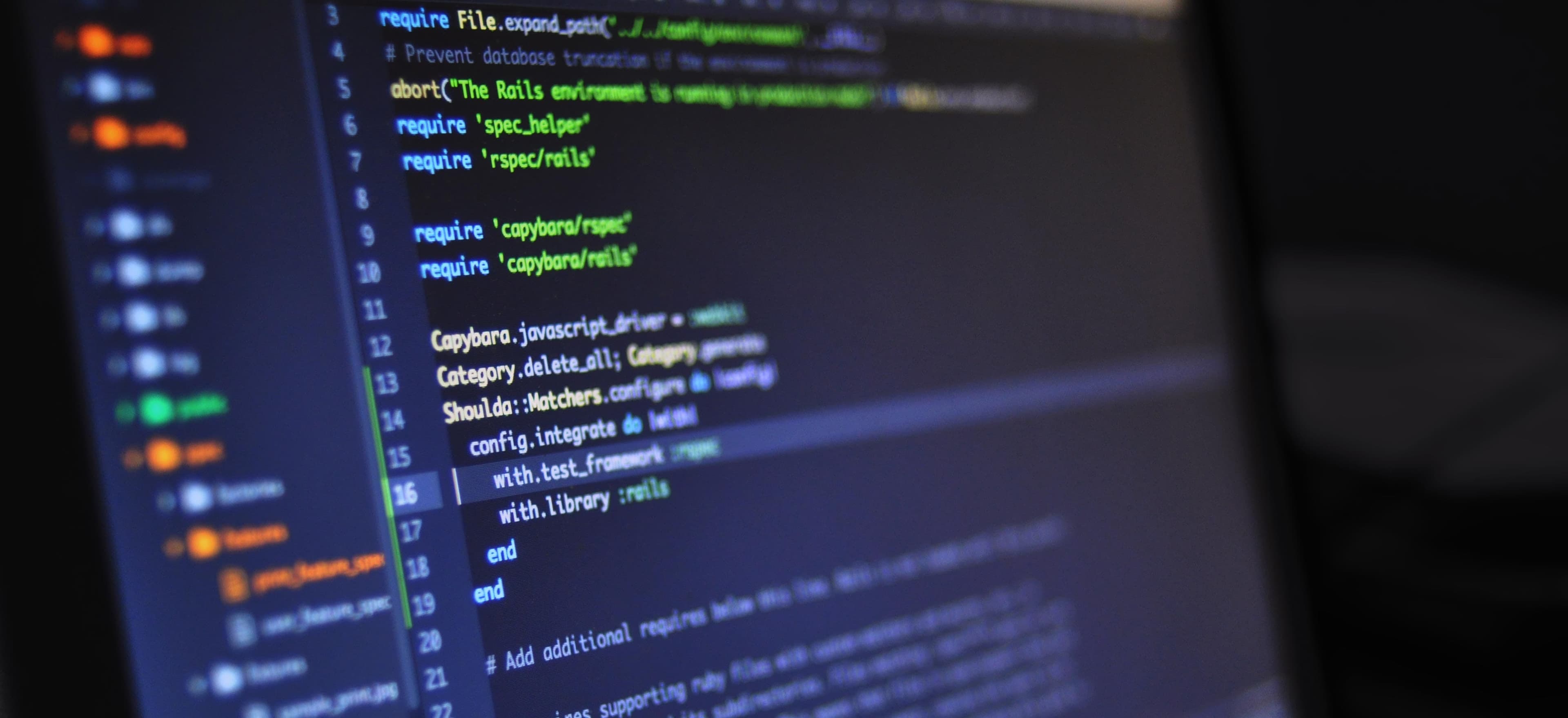
- Published on
Block Rogue Code: How to Prevent System.exit() Misuse
When developing a Java application, it's essential to maintain control over the behavior of the code and prevent potential security risks. One of the methods that can be misused in Java is System.exit()
, which allows the abrupt termination of the Java Virtual Machine (JVM). This can be a significant security vulnerability if not handled properly. In this article, we'll discuss the potential dangers of misusing System.exit()
and explore several strategies for preventing its misuse in your Java applications.
Understanding System.exit() and Its Risks
The System.exit()
method is a part of the java.lang.System
class and allows a Java application to terminate execution and shut down the JVM. While there are certainly legitimate use cases for using System.exit()
, such as indicating a successful or unsuccessful completion of the application, there are also significant risks associated with its misuse.
Potential Risks of Misusing System.exit()
One of the primary risks of misuse is that it can lead to unexpected termination of the application, resulting in potential data corruption or loss of unsaved information. Additionally, in a multi-threaded application, misuse of System.exit()
can cause inconsistent behavior and potential deadlock situations. From a security standpoint, an attacker could potentially exploit improper use of System.exit()
to force the premature termination of the application, leading to a denial-of-service attack.
Strategies for Preventing System.exit() Misuse
To mitigate the risks associated with the misuse of System.exit()
, developers can employ various strategies to control its usage and prevent unauthorized termination of the application. Below are some effective methods for achieving this goal.
Security Manager
Utilizing a SecurityManager
is a powerful way to control the usage of System.exit()
. By implementing a custom SecurityManager
, you can selectively grant or deny exitVM
permission to specific parts of the code. This approach allows you to restrict the usage of System.exit()
to authorized sections of the application while preventing its misuse in other areas.
public class CustomSecurityManager extends SecurityManager {
@Override
public void checkPermission(Permission perm) {
if (perm.getName().contains("exitVM")) {
// Check for authorized usage of exitVM
if (!isAuthorized()) {
throw new SecurityException("Unauthorized System.exit() attempt");
}
}
}
private boolean isAuthorized() {
// Implement custom logic to determine authorized usage
// Return true if authorized, false otherwise
}
}
In the code snippet above, the CustomSecurityManager
overrides the checkPermission
method to intercept and enforce permission checks for System.exit()
. It is crucial to implement the isAuthorized
method to define the logic for determining authorized usage.
Aspect-Oriented Programming (AOP)
Another approach to prevent misuse of System.exit()
is to leverage Aspect-Oriented Programming (AOP). AOP allows you to define cross-cutting concerns, such as permission checks, separately from the core application logic. By applying AOP, you can intercept calls to System.exit()
and enforce additional authorization checks before allowing the termination of the JVM.
@Aspect
public class ExitAuthorizationAspect {
@Before("execution(* java.lang.System.exit(..))")
public void checkExitAuthorization() {
if (!isAuthorized()) {
throw new SecurityException("Unauthorized System.exit() attempt");
}
}
private boolean isAuthorized() {
// Implement custom logic to determine authorized usage
// Return true if authorized, false otherwise
}
}
In the AOP-based approach depicted above, the ExitAuthorizationAspect
intercepts calls to System.exit()
and enforces authorization checks before the method is allowed to execute. The isAuthorized
method should contain the logic for determining authorized usage.
Custom Security Wrapper
Developers can create a custom wrapper around the System.exit()
method to encapsulate additional security checks. By centralizing the permission checks within the wrapper, it becomes easier to manage and audit the usage of System.exit()
throughout the application.
public class SecureSystemExit {
public static void exit(int status) {
if (!isAuthorized()) {
throw new SecurityException("Unauthorized System.exit() attempt");
}
System.exit(status);
}
private static boolean isAuthorized() {
// Implement custom logic to determine authorized usage
// Return true if authorized, false otherwise
}
}
The SecureSystemExit
class above provides a secure wrapper around System.exit()
, ensuring that authorization checks are performed before allowing the termination of the JVM. Developers can then utilize SecureSystemExit
in place of direct calls to System.exit()
.
Code Reviews and Best Practices
In addition to technical countermeasures, promoting best practices and conducting code reviews can help prevent the misuse of System.exit()
. By establishing coding guidelines and educating developers on the proper usage of System.exit()
, organizations can significantly reduce the likelihood of inadvertent or malicious misuse.
A Final Look
In conclusion, the System.exit()
method in Java can pose significant risks if misused, leading to unexpected application termination and potential security vulnerabilities. By employing strategies such as implementing a SecurityManager
, leveraging Aspect-Oriented Programming, creating custom security wrappers, and promoting best practices, developers can effectively mitigate the risks associated with the misuse of System.exit()
.
Preventing unauthorized termination of the application is crucial for ensuring the stability, integrity, and security of Java applications. By implementing the aforementioned strategies, developers can maintain control over the usage of System.exit()
and minimize the potential impact of its misuse.
Incorporating these preventive measures contributes to the overall security posture of Java applications and demonstrates a proactive approach to mitigating potential vulnerabilities.
Remember to always thoroughly test your security measures and stay updated on best practices to keep your Java applications secure and reliable.
For further information on Java security, consider exploring the official Java security documentation and the OWASP Java Security cheat sheet.
Stay secure and keep coding responsibly!