Overcoming Scala's Steep Learning Curve: A Guide
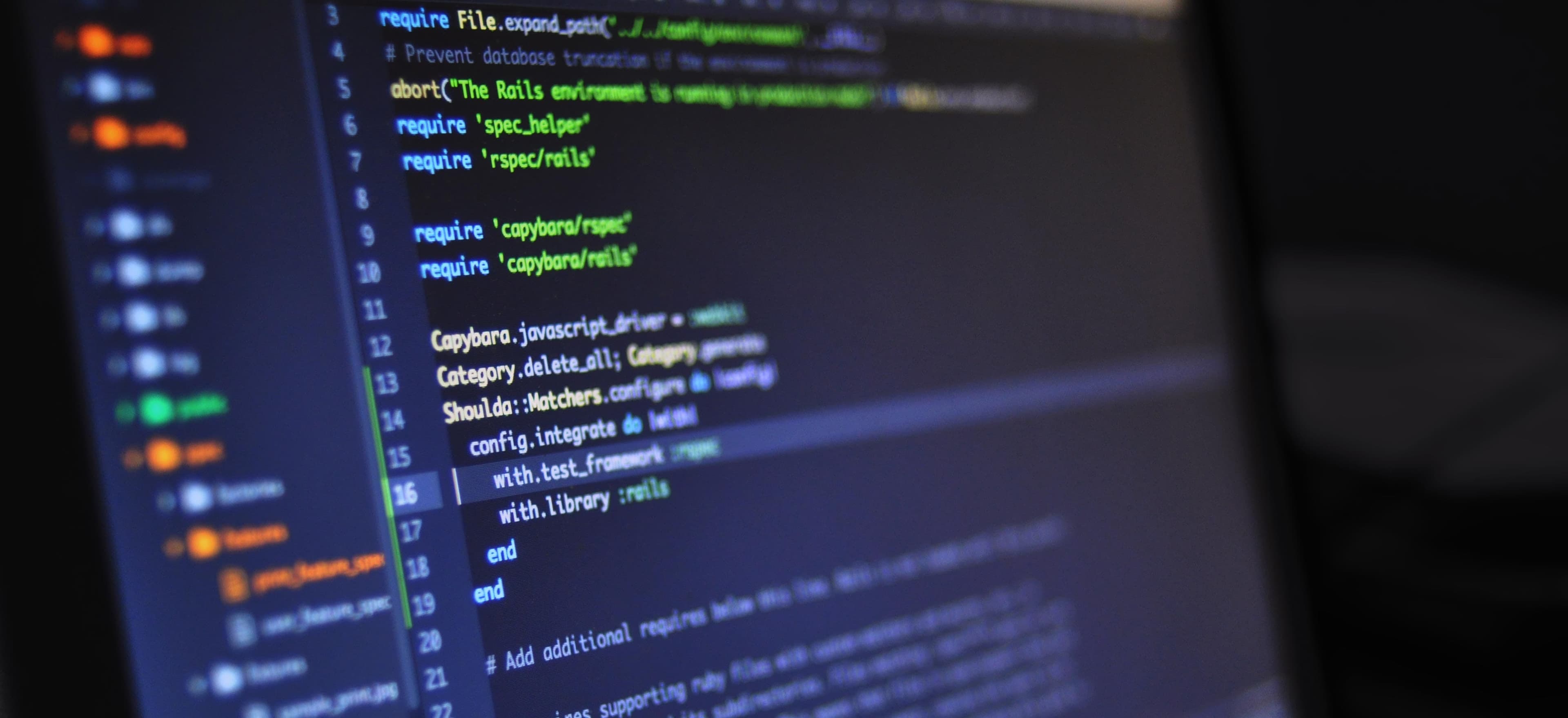
- Published on
Overcoming Scala's Steep Learning Curve: A Guide
If you've dabbled in the world of programming, you might have stumbled upon Scala - a powerful language known for its conciseness and versatility. However, it's no secret that Scala has a reputation for having a steep learning curve, often intimidating beginners and even seasoned developers. But fear not, for in this guide, we'll explore some strategies and tips to overcome Scala's complexities and embrace its potential.
Understanding the Challenge
Scala, a fusion of object-oriented and functional programming, introduces a range of paradigms and concepts that can be daunting for newcomers. Features like pattern matching, implicit conversions, and advanced type system may initially seem overwhelming. However, the key to mastering Scala lies in breaking down these complexities and understanding the underlying principles.
Embracing Functional Programming
One of the core strengths of Scala is its support for functional programming. Embracing immutability, higher-order functions, and pattern matching can enhance code clarity and maintainability. Let's dive into an example:
Immutability
// Immutable List
val numbers = List(1, 2, 3, 4, 5)
val updatedNumbers = numbers :+ 6 // Creates a new list with an additional element
In the above code snippet, we utilize immutability by creating a new list updatedNumbers
instead of modifying the original numbers
. This approach minimizes side effects and facilitates easier debugging.
Higher-Order Functions
// Filtering even numbers
val evenNumbers = numbers.filter(_ % 2 == 0)
Here, the filter
function is a higher-order function that takes a predicate and returns a new list with elements satisfying the predicate. Leveraging higher-order functions promotes reusability and expressive code.
Pattern Matching
// Pattern matching to process different cases
def processData(data: Any): String = data match {
case str: String => s"Received a string: $str"
case num: Int => s"Received an integer: $num"
case _ => "Unknown data"
}
Pattern matching simplifies complex conditional statements and enhances the robustness of code, making it a valuable asset in Scala's functional paradigm.
Leveraging Scala's Type System
Scala's rich type system, including powerful features like traits, case classes, and algebraic data types, can initially seem intricate. However, comprehending and leveraging these features can lead to more robust and expressive code.
Case Classes
// Defining a case class
case class Person(name: String, age: Int)
val person = Person("Alice", 30)
With case classes, we effortlessly create immutable data models with built-in equality checks, pattern matching support, and companion objects for free.
Traits
// Defining a trait
trait Shape {
def area: Double
def color: String
}
// Implementing the trait with a case class
case class Circle(radius: Double, color: String) extends Shape {
def area: Double = math.Pi * radius * radius
}
Traits enable the creation of composable and reusable abstractions, promoting code modularity and flexibility.
Type Inference
// Inferred type based on the return value
def add(a: Int, b: Int) = a + b
Scala's type inference reduces verbosity while maintaining type safety, offering a balance between clarity and brevity.
Tackling Concurrency and Asynchronous Programming
Scala's robust support for concurrency and asynchronous programming is both a blessing and a challenge. Understanding and effectively utilizing features like futures, actors, and the Future
API can significantly enhance the scalability and responsiveness of applications.
Futures
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
// Asynchronous computation with Future
val futureResult: Future[Int] = Future {
// Perform some asynchronous computation
42
}
// Handling the future result
futureResult.onComplete {
case Success(result) => println(s"Computation result: $result")
case Failure(ex) => println(s"Computation failed: ${ex.getMessage}")
}
Futures facilitate non-blocking, asynchronous computations, allowing for efficient use of resources and improved responsiveness.
Actors
import akka.actor.{Actor, ActorSystem, Props}
// Defining an actor
class HelloActor extends Actor {
def receive = {
case "hello" => println("Hello back at you")
}
}
// Creating an actor system and supervising actor
val system = ActorSystem("HelloSystem")
val helloActor = system.actorOf(Props[HelloActor], name = "helloactor")
// Sending a message to the actor
helloActor ! "hello"
Actors provide a powerful concurrency model, simplifying the handling of parallel tasks and encapsulating stateful computations.
Embracing Tooling and Ecosystem
Scala's tooling and ecosystem play a vital role in enhancing productivity and code quality. Leveraging build tools like sbt, testing frameworks like ScalaTest, and popular libraries like Akka and Play Framework can streamline development processes and expand your Scala prowess.
sbt (Scala Build Tool)
sbt simplifies the building and management of Scala projects, offering features like incremental compilation, dependency management, and plugin support.
ScalaTest
ScalaTest empowers developers to write expressive and comprehensive tests, promoting a test-driven development approach and ensuring code reliability.
Akka
Akka provides a toolkit for building concurrent, distributed, and resilient applications, reinforcing the capabilities of Scala in handling complex use cases.
Summary
Scala's intricacies and advanced features may pose a challenge, but with a systematic approach and perseverance, mastering the language is well within reach. Embracing functional programming, leveraging the type system, tackling concurrency, and embracing the tooling and ecosystem are pivotal steps in overcoming Scala's steep learning curve. So, dive into the world of Scala with confidence, explore its rich potential, and elevate your programming prowess.
Now, armed with a deeper understanding of Scala and its nuances, you're ready to conquer new horizons with this powerful and expressive language. Happy coding!
Remember, the journey to mastering Scala is as enriching as the destination itself. Happy coding!