Java 8 Date API Woes: Unraveling Common Pitfalls
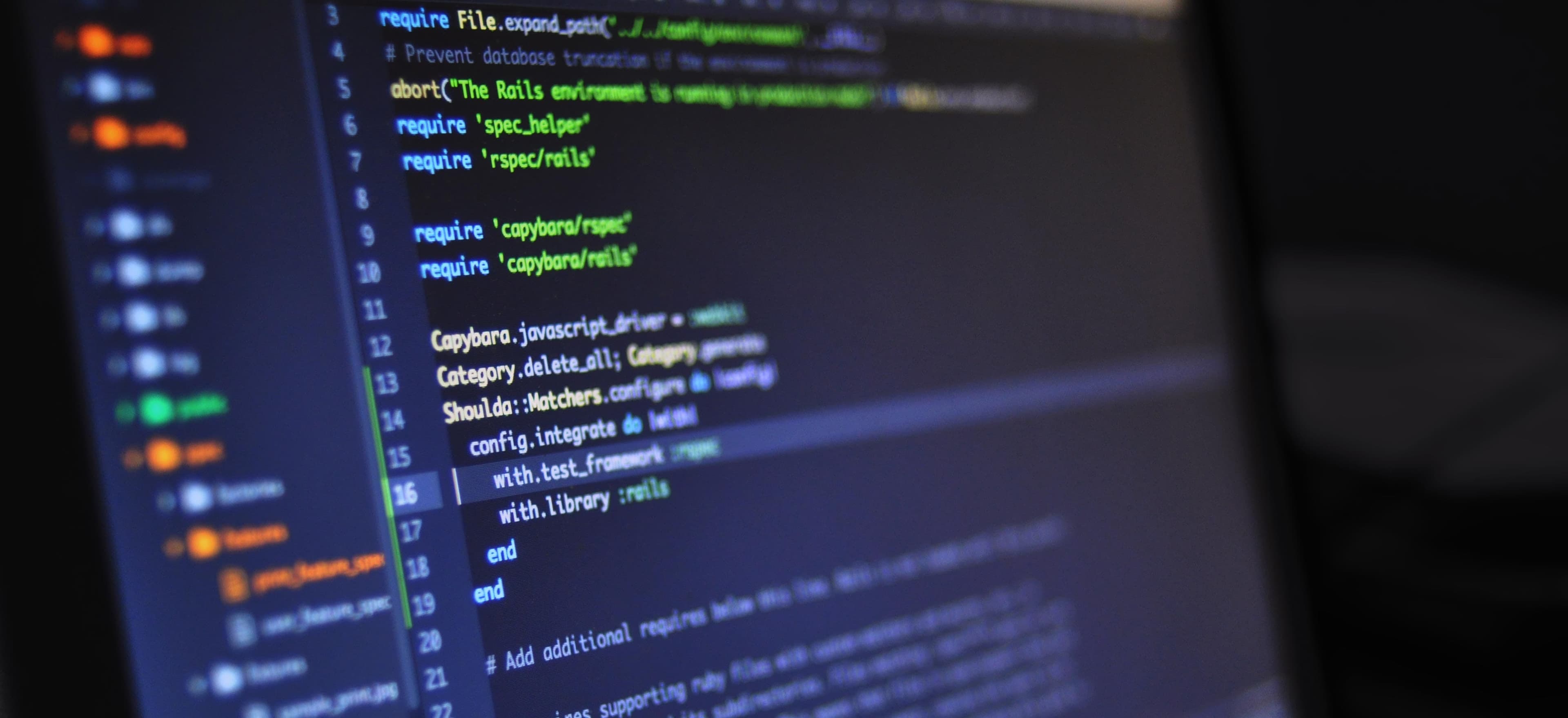
- Published on
Java 8 Date API Woes: Unraveling Common Pitfalls
Dealing with dates and times in Java has always been a bit of a hassle. With the introduction of the Date and Time API in Java 8, many hoped for a remedy to the long-standing issues. However, working with the Java 8 Date API has its own share of challenges and pitfalls that developers should be aware of. In this post, we will delve into some common pitfalls when using the Java 8 Date API and explore potential solutions.
Pitfall 1: Immutability and Chaining
One of the key features of the Java 8 Date API is immutability, which means that once a date/time object is created, its value cannot be changed. While immutability has its benefits in terms of thread safety and predictability, it also introduces challenges when performing operations in a chain.
Consider the following code snippet:
LocalDate date = LocalDate.now();
date.plusDays(7);
System.out.println(date);
One might expect the date to be seven days in the future. However, due to the immutability of the LocalDate
class, the plusDays
method returns a new LocalDate
object instead of modifying the original date
object. Therefore, the output will still be the current date.
To overcome this pitfall, it is essential to capture the result of the operation by reassigning the return value to the original variable:
LocalDate date = LocalDate.now();
date = date.plusDays(7);
System.out.println(date);
By reassigning the result of the plusDays
operation back to the date
variable, the desired outcome of adding seven days to the original date is achieved.
Pitfall 2: Timezone Handling
Handling timezones correctly is crucial when working with dates and times. The Java 8 Date API provides the ZoneId
class to represent timezones, and the ZonedDateTime
class to handle date and time with a timezone.
Let's take a look at a common pitfall related to timezone handling:
LocalDateTime localDateTime = LocalDateTime.now();
ZonedDateTime zonedDateTime = ZonedDateTime.of(localDateTime, ZoneId.of("Europe/Paris"));
System.out.println(zonedDateTime);
In this example, the LocalDateTime.now()
method returns the current date and time in the system's default timezone. However, when creating a ZonedDateTime
by combining a LocalDateTime
with a specific timezone using the of
method, it's crucial to be aware of the potential pitfalls related to daylight saving time and offset changes.
When dealing with timezones, it's recommended to use the ZoneOffset
class to capture the offset from UTC, or to rely on libraries such as ThreeTen-Extra to handle complex timezone operations.
Pitfall 3: Parsing and Formatting
Parsing and formatting dates and times is a common task in many applications. The Java 8 Date API provides the DateTimeFormatter
class to facilitate this, but there are still several pitfalls to watch out for.
String dateStr = "2022-12-31";
LocalDate date = LocalDate.parse(dateStr);
System.out.println(date);
The code above looks innocent, but it can throw a DateTimeParseException
at runtime due to the default format expected by the parse
method. The parse
method expects the input string to be in ISO-8601 format by default.
To avoid this pitfall, always specify the format expected while parsing:
String dateStr = "2022-12-31";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd");
LocalDate date = LocalDate.parse(dateStr, formatter);
System.out.println(date);
By providing a custom DateTimeFormatter
with the expected date format, the parsing operation becomes more robust and less error-prone.
Pitfall 4: Interoperability with Legacy Code
When working on projects that involve legacy code or external libraries that have not yet migrated to the Java 8 Date API, interoperability concerns may arise. It's not uncommon to encounter situations where you need to convert between the Java 8 Date API and the legacy java.util.Date
or java.util.Calendar
classes.
One common pitfall in such scenarios is improper handling of timezones during conversions, leading to ambiguity and potential bugs.
To mitigate this pitfall, it's advisable to leverage the Instant
class for conversions between the Java 8 Date API and legacy date/time classes. The Instant
class represents a point in time on the time-line in UTC and can be used as an intermediary when converting between different date/time representations.
Being conscious of the potential pitfalls and taking care to handle interoperability gracefully can save developers from headaches down the line.
My Closing Thoughts on the Matter
The Java 8 Date API brought significant improvements to date and time handling in Java, but it's not without its challenges. By being aware of the common pitfalls and knowing how to overcome them, developers can navigate the intricacies of the Java 8 Date API more effectively.
Incorporating best practices, leveraging external libraries where necessary, and remaining vigilant about timezone-related considerations can go a long way in taming the complexities of date and time manipulation in Java.
Remember, dates and times may appear simple on the surface, but beneath that simplicity lies a world of complexities that require attention to detail and a solid understanding of the tools at hand.
So, the next time you embark on a journey through the realm of dates and times in Java, arm yourself with the knowledge to conquer the Java 8 Date API pitfalls that may lie ahead.
Happy coding!
Ensure your date and time handling in Java is impeccable by avoiding common pitfalls. Learn more about Java 8 Date and Time API and the ThreeTen-Extra library for advanced timezone operations.