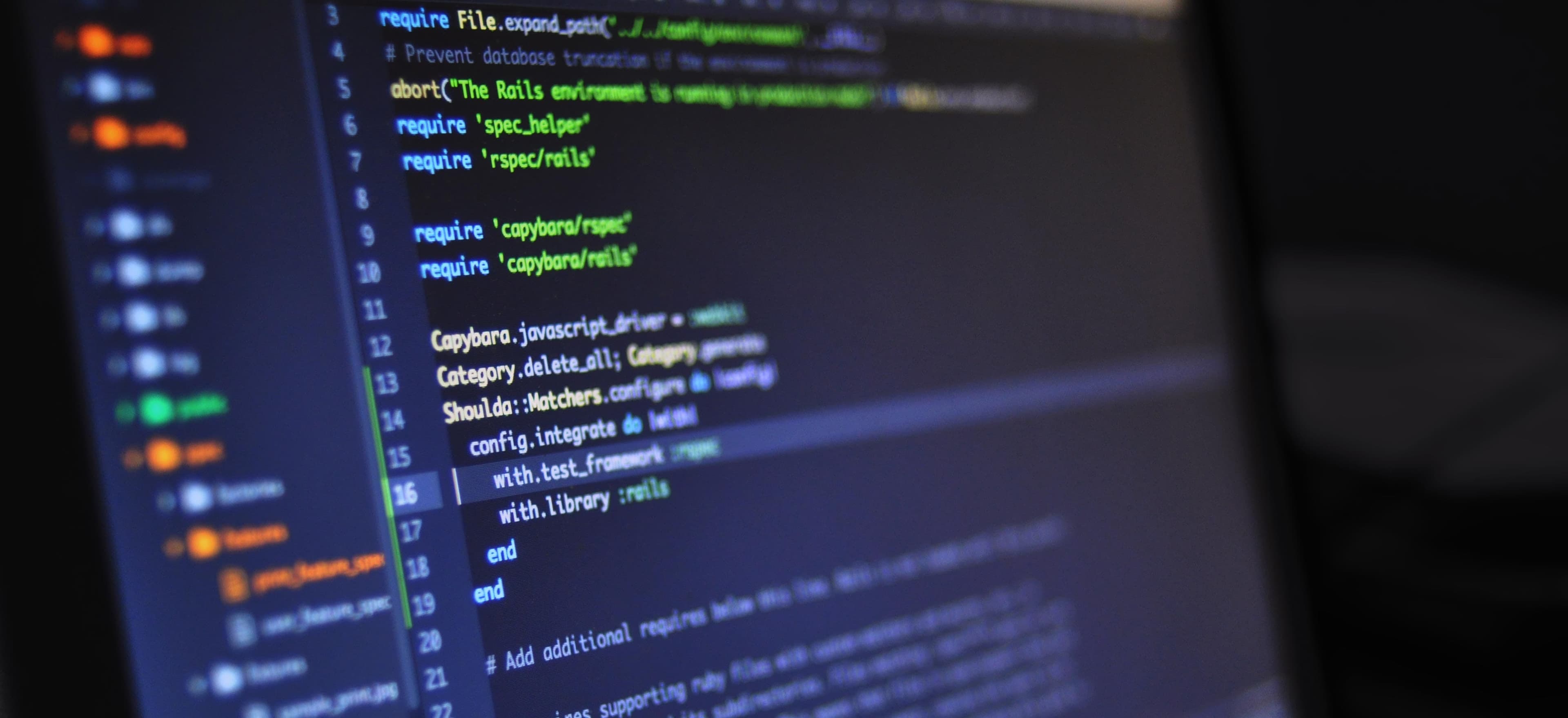
- Published on
Securing Your Data: Navigating the Maze of Database Threats
In today's digital age, data security is of paramount importance. With the increasing number of cyber threats, it's crucial to ensure that our databases are secure. In this blog post, we'll explore the various threats that databases face and how Java developers can protect their data from these vulnerabilities.
Understanding Database Threats
Before diving into the specifics of securing databases, it's essential to understand the various threats that databases are susceptible to. Some common threats include:
-
SQL Injection: This is a technique where malicious actors insert malicious SQL statements into input fields, potentially giving them unauthorized access to the database.
-
Unauthorized Access: If proper access controls are not in place, unauthorized users may gain access to sensitive data.
-
Data Leakage: This occurs when sensitive data is unintentionally exposed to unauthorized users.
-
Cross-Site Scripting (XSS): Attackers inject malicious scripts into web pages viewed by other users.
Now that we've identified some common threats, let's explore how Java developers can mitigate these risks.
Using Prepared Statements to Prevent SQL Injection
One of the most common and dangerous threats to databases is SQL injection. To prevent SQL injection attacks, Java developers should use prepared statements when interacting with the database.
Here's an example of using prepared statements with JDBC:
String username = request.getParameter("username");
String password = request.getParameter("password");
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost/database", "username", "password");
PreparedStatement preparedStatement = connection.prepareStatement("SELECT * FROM users WHERE username = ? AND password = ?");
preparedStatement.setString(1, username);
preparedStatement.setString(2, password);
ResultSet resultSet = preparedStatement.executeQuery();
In this example, we use a prepared statement to safely pass the username
and password
parameters to the database, preventing any malicious SQL injection attempts. This simple yet powerful technique can greatly enhance the security of your database interactions.
Implementing Role-Based Access Control
Unauthorized access to databases can lead to catastrophic breaches. Implementing role-based access control (RBAC) can help mitigate this risk. RBAC restricts access to certain parts of the database based on the user's role.
Let's see how we can implement RBAC using Java's Spring Security framework:
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/admin/**").hasRole("ADMIN")
.antMatchers("/user/**").hasRole("USER")
.anyRequest().authenticated()
.and()
.formLogin();
}
// Other security configurations...
}
In this example, we configure Spring Security to restrict access to certain URLs based on the user's role. This ensures that only authorized users can access specific parts of the application, thereby enhancing the overall security of the database.
Data Encryption at Rest and in Transit
In addition to preventing unauthorized access, it's also crucial to ensure that data is encrypted both at rest and in transit. Java developers can achieve this by using encryption libraries such as Bouncy Castle or Java's built-in javax.crypto
package.
When interacting with the database, sensitive data like passwords or credit card numbers should be encrypted before being stored. Furthermore, when data is transmitted over the network, it should be encrypted to prevent eavesdropping.
Regular Security Audits and Patch Management
Even with robust security measures in place, it's crucial to regularly audit your database for potential vulnerabilities. This involves conducting security assessments, vulnerability scanning, and penetration testing to identify and remediate any weaknesses.
Additionally, staying up to date with security patches for your database management system is essential. Security patches often contain crucial updates and fixes for known vulnerabilities, so ensuring that your database system is always up to date is paramount to its security.
The Last Word
Securing your data is a complex and ongoing process. By understanding the various threats that databases face and implementing robust security measures, Java developers can protect their data from potential breaches. Utilizing techniques such as prepared statements for SQL injection prevention, role-based access control, data encryption, and regular security audits can greatly enhance the security of your database.
By staying vigilant and proactive in addressing potential security threats, Java developers can navigate the maze of database threats and safeguard their data from malicious actors.
Remember, data security is not a one-time task, but an ongoing commitment to protecting sensitive information from ever-evolving threats.
Now that we've explored strategies for securing databases in Java applications, it's crucial to stay informed about the latest developments in the field of data security and to continuously adapt our practices to meet new challenges.
For more in-depth insights into database security, check out Best Practices for Database Security and OWASP Top Ten.
Stay safe, and keep your data secure!