Tackling Concurrency Challenges with Java 8 Lambdas
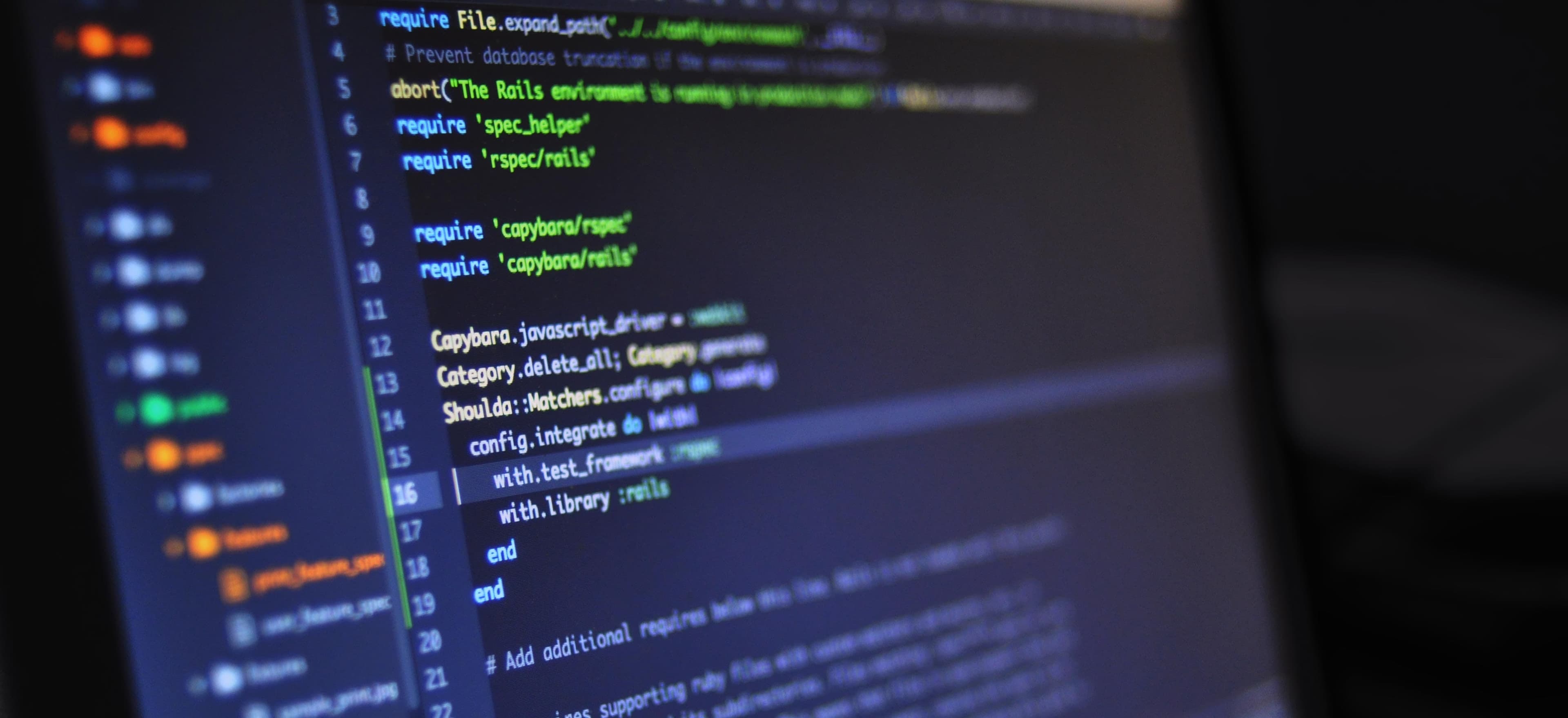
- Published on
Tackling Concurrency Challenges with Java 8 Lambdas
Java 8 introduced a new way of writing code with the addition of lambdas and the Stream API. While these features are often associated with functional programming and data manipulation, they also offer a powerful way to tackle concurrency challenges. In this blog post, we'll explore how Java 8 lambdas can be used to simplify and streamline concurrent programming tasks.
The Traditional Approach to Concurrency in Java
Before Java 8, writing concurrent code in Java often involved working with explicit Thread
objects, managing synchronization through the synchronized
keyword, and dealing with the complexities of shared mutable state. While effective, this approach could lead to cumbersome and error-prone code, making it hard to reason about the behavior of concurrent programs.
Introducing Java 8 Lambdas for Concurrency
With the introduction of lambdas and the Stream API in Java 8, developers gained a more expressive and concise way to work with collections and perform operations in a parallel manner. This functional programming paradigm opened up new possibilities for tackling concurrency challenges.
Simplifying Parallel Operations with Lambdas
One of the key features of Java 8 lambdas is their ability to enable parallel processing of collections through the Stream API. By simply calling the parallelStream()
method on a collection, developers can leverage the power of multi-core processors to speed up data processing tasks.
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10);
int sum = numbers.parallelStream()
.filter(n -> n % 2 == 0)
.mapToInt(n -> n * 2)
.sum();
In the code snippet above, we use a parallel stream to filter even numbers and then map each number to its doubled value before calculating the sum. Behind the scenes, Java takes care of distributing the workload across multiple threads, making it easier to harness the power of concurrency without explicitly managing threads and synchronization.
Enhanced Error Handling with Java 8 Lambdas
Handling exceptions in concurrent code has also been simplified with Java 8 lambdas. The CompletableFuture
class, introduced in Java 8, allows developers to chain asynchronous operations and handle exceptions using fluent, lambda-based syntax.
CompletableFuture<Integer> futureResult = CompletableFuture.supplyAsync(() -> {
// Perform asynchronous computation
return performComputation();
}).thenApply(result -> result * 2)
.exceptionally(ex -> {
log.error("An error occurred: " + ex.getMessage());
return 0;
});
In the above example, we use CompletableFuture
to perform an asynchronous computation and then apply a transformation to the result. If an exception occurs during the computation or transformation, the exceptionally
method allows us to gracefully handle the error using a lambda expression.
Leveraging Immutability and Pure Functions
Java 8 lambdas encourage the use of immutability and pure functions, which are essential principles in writing thread-safe and concurrent code. By embracing immutability, concurrent access to shared data becomes less error-prone, as there is no risk of unexpected modifications from different threads. Pure functions, which produce the same result for the same inputs and have no side effects, enable safer parallel execution without introducing hidden dependencies or conflicts.
My Closing Thoughts on the Matter
Java 8 lambdas have brought a breath of fresh air to concurrent programming in Java, providing a more expressive, concise, and safer way to handle concurrent operations. By leveraging the power of functional programming, developers can simplify parallel processing, enhance error handling, and embrace best practices such as immutability and pure functions.
In conclusion, the adoption of Java 8 lambdas opens up new avenues for addressing concurrency challenges and empowers developers to write more robust and maintainable concurrent code.
To delve deeper into concurrent programming and Java 8 lambdas, I recommend checking out the official Java documentation and exploring Java Concurrency in Practice by Brian Goetz and team.
Keep coding with confidence and concurrency in mind!
Checkout our other articles