Unlocking Success: The Power of Second-Level Thinking
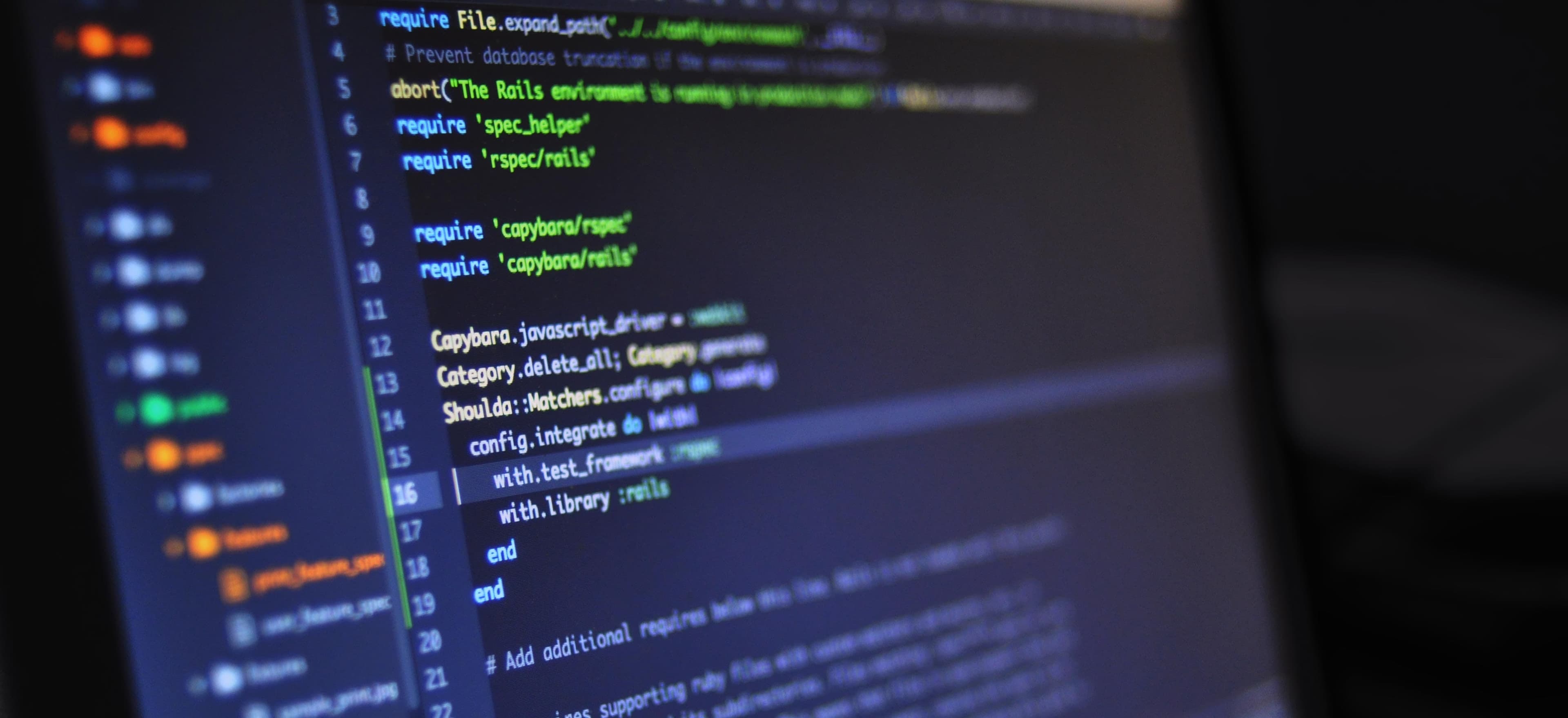
- Published on
The Power of Second-Level Thinking in Java Programming
In the world of Java programming, success often comes down to the ability to think beyond the obvious. Second-level thinking, a concept popularized by renowned investor Howard Marks, involves considering not just the immediate consequences of a decision, but also the broader and longer-term implications. This approach is equally applicable in the realm of writing high-quality Java code, as it allows developers to anticipate potential pitfalls and design more robust solutions.
Embracing Complexity with Second-Level Thinking
Java is a versatile and powerful language, enabling developers to tackle a wide range of projects, from enterprise-level applications to mobile development. However, this flexibility also introduces complexity. Second-level thinking encourages developers to embrace this complexity, acknowledging that a successful Java application is more than just the sum of its individual components.
Consider the following scenario: when designing a new feature for an existing Java application, a first-level thinker may focus solely on the immediate functionality. They may implement the feature in a straightforward manner, addressing the immediate requirements without considering how it may interact with future updates or scalability.
On the other hand, a second-level thinker would take a more comprehensive approach. They would consider the broader implications of the new feature, such as its impact on the overall architecture, its potential dependencies, and its adaptability to future enhancements. This mindset enables developers to build more resilient and adaptable solutions, positioning their Java applications for long-term success.
Implementing Second-Level Thinking in Java Programming
To illustrate the practical application of second-level thinking in Java programming, let's consider a common scenario: implementing error handling in a web application. A first-level approach might involve handling each specific error case individually, leading to a cumbersome and repetitive code structure.
try {
// Code that may throw an exception
} catch (IOException e) {
// Handle IOException
} catch (SQLException e) {
// Handle SQLException
} catch (CustomException e) {
// Handle CustomException
}
While this code addresses immediate error handling needs, a second-level thinker would recognize the opportunity to create a more robust and maintainable solution. By leveraging the concept of second-level thinking, the developer can design a centralized error handling mechanism using exception chaining and logging, improving code readability and maintainability.
try {
// Code that may throw an exception
} catch (Exception e) {
logger.error("An error occurred", e);
// Additional error handling or rethrowing the exception
}
In this approach, the second-level thinker anticipates potential future exceptions and creates a more comprehensive error handling strategy that aligns with the long-term maintainability of the application. This demonstrates how second-level thinking in Java programming extends beyond immediate requirements to encompass future-proofing and adaptability.
Leveraging Design Patterns with Second-Level Thinking
Another area where second-level thinking is invaluable in Java programming is the utilization of design patterns. Design patterns provide proven solutions to recurring design problems, offering a structured approach to software development. While first-level thinking may result in the direct application of a design pattern to address a specific issue, a second-level thinker explores the underlying reasons behind the pattern and its implications in different contexts.
For example, when implementing the Singleton pattern to ensure a class has only one instance, a first-level thinker may focus solely on the immediate need for a single instance and implement the pattern without considering potential drawbacks in a multithreaded environment.
A second-level thinker, however, would delve deeper into the intricacies of the Singleton pattern and acknowledge the importance of thread safety. By integrating this awareness into the implementation, they ensure the Singleton instance is both lazily initialized and thread-safe, demonstrating a holistic understanding of the pattern and its implications.
The Impact of Second-Level Thinking on Code Quality
The adoption of second-level thinking in Java programming enhances not only the functionality of the code but also its quality and maintainability. By anticipating future requirements and potential complexities, developers can design more extensible and adaptable solutions that stand the test of time.
Moreover, second-level thinking encourages a proactive approach to problem-solving, fostering a mindset that prioritizes thorough analysis and comprehensive solutions over short-term fixes. This approach ultimately leads to a reduction in technical debt, as developers address potential issues before they escalate, resulting in more robust and stable Java applications.
Final Thoughts
In the dynamic and ever-evolving landscape of Java programming, second-level thinking serves as a guiding principle for ensuring the long-term success of applications. By considering the broader implications of design decisions, embracing complexity, and leveraging design patterns with a holistic understanding, developers can elevate the quality of their code and position their applications for scalability and adaptability.
Embracing second-level thinking requires a shift in mindset, encouraging developers to move beyond immediate requirements and consider the long-term impact of their design choices. This approach not only enhances the functionality of Java applications but also fosters a culture of excellence and foresight within development teams.
As Java developers continue to navigate the complexities of software development, the power of second-level thinking stands as a steadfast ally, empowering them to unlock the full potential of their code and pave the way for continued success in an ever-changing technological landscape.
Remember, the next time you sit down to write Java code, take a moment to elevate your thinking to the second level. The results might just exceed your expectations.
For further exploration, delve into the nuances of second-level thinking by delving into the Java documentation and exploring the intricate details of design patterns through resources such as the Gang of Four book. Happy coding!
Checkout our other articles