Array to List in Java: A Common Pitfall Explained!
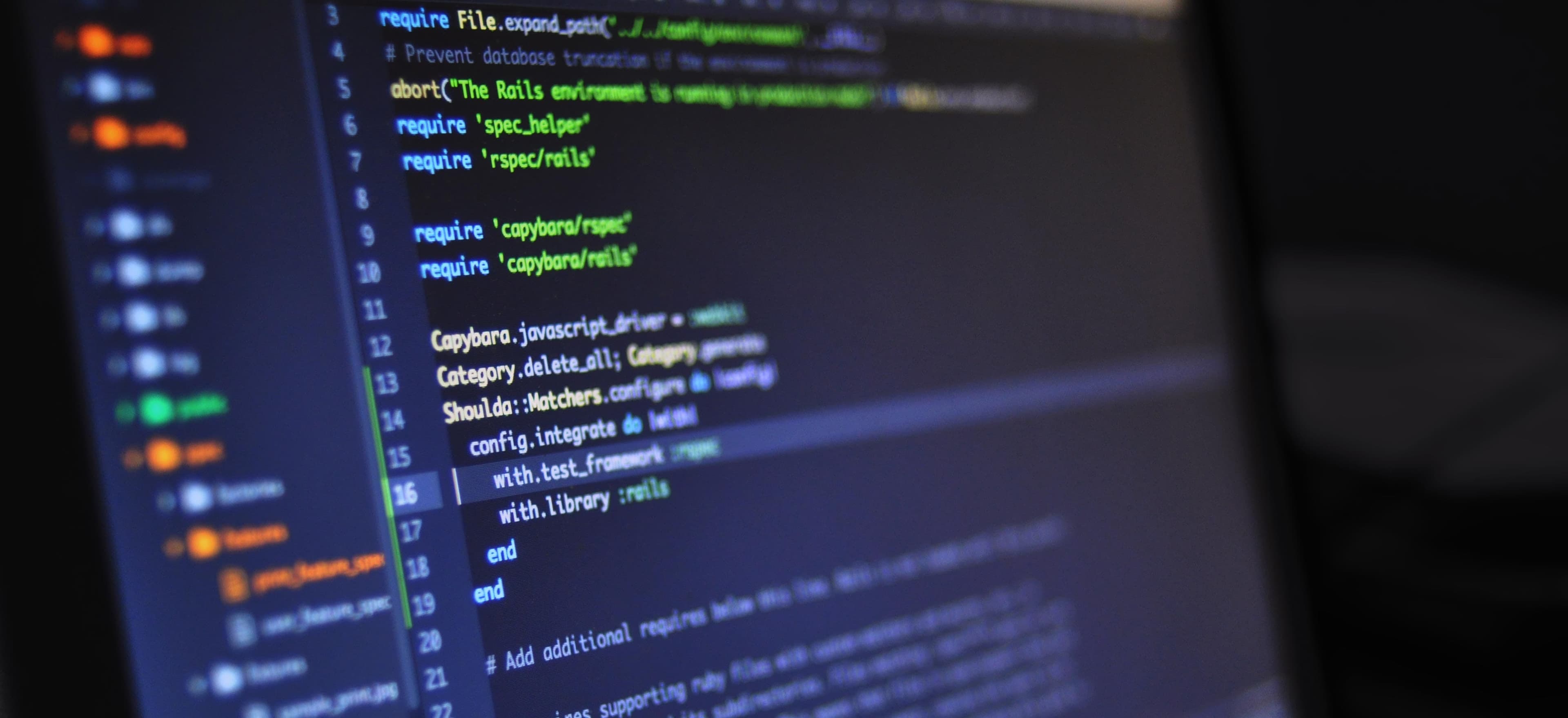
- Published on
Converting an Array to a List in Java: Avoiding Common Pitfalls
When it comes to Java programming, working with arrays and lists is a common task. While both arrays and lists serve the purpose of storing elements, they have different characteristics and capabilities. Java arrays are fixed in size, while lists, such as ArrayList or LinkedList, can dynamically adjust their size.
One frequent operation in Java programming is the need to convert an array to a list. At first glance, this seems like a simple and straightforward task. However, a common pitfall often arises during this process, causing unexpected behavior in the code.
In this article, we will explore the potential pitfalls of converting an array to a list in Java, and how to effectively navigate and avoid them.
The Misconception of Arrays.asList()
The Arrays
class in Java provides a method called asList()
that can be used to convert an array to a list. At first glance, this method seems like a convenient way to achieve the desired conversion. However, a critical point to note is that Arrays.asList()
returns a fixed-size list backed by the original array. This means that any structural modifications to the returned list (e.g., adding or removing elements) will result in an UnsupportedOperationException
.
Let's illustrate this with an example:
Integer[] myArray = {1, 2, 3, 4, 5};
List<Integer> myList = Arrays.asList(myArray);
myList.add(6); // This line will throw UnsupportedOperationException
In the above example, attempting to add an element to the list obtained from Arrays.asList()
will result in a runtime UnsupportedOperationException
, as the returned list is fixed-size and does not support structural modifications.
Converting Array to ArrayList: The Solution
To avoid the limitation posed by Arrays.asList()
, a reliable approach is to utilize the ArrayList
constructor that accepts a collection. By using this approach, we can create a modifiable ArrayList
from the original array without encountering the pitfalls observed with Arrays.asList()
.
Let's consider the corrected implementation:
Integer[] myArray = {1, 2, 3, 4, 5};
List<Integer> myList = new ArrayList<>(Arrays.asList(myArray));
myList.add(6); // This will add the element 6 to the list without any issues
By constructing a new ArrayList
from the Arrays.asList()
result, we effectively create a modifiable list without constraints, allowing seamless additions, removals, and other operations.
Dealing with Primitive Arrays: Introducing the Stream API
When working with primitive arrays (e.g., int[], double[], etc.), the approach of using Arrays.asList()
is not applicable, as it only works with reference types (e.g., Integer[], String[], etc.). In such cases, Java 8 introduced the Stream API, which provides a succinct and elegant solution for converting primitive arrays to lists.
Let's delve into an example utilizing the Stream API for this purpose:
int[] intArray = {1, 2, 3, 4, 5};
List<Integer> intList = Arrays.stream(intArray).boxed().collect(Collectors.toList());
In the above code snippet, the Arrays.stream(intArray)
method starts a stream of the elements in the intArray
, which are then boxed using the boxed()
method to convert them into their corresponding wrapper objects (e.g., Integer). After boxing, the collected elements are gathered into a list using the collect(Collectors.toList())
operation.
Performance Considerations: Array to List Conversion
When discussing the conversion of an array to a list, performance considerations come into play. It is essential to evaluate the performance implications of different approaches, particularly when dealing with large datasets or in performance-critical applications.
The Arrays.asList()
method, as mentioned earlier, returns a fixed-size list backed by the original array, providing a limited capability for modifications. On the other hand, when using the ArrayList
constructor or the Stream API for the conversion, an entirely new list is constructed, allowing for unfettered modifications, but incurring the overhead of creating a new object.
In scenarios where the original array is subject to frequent changes, using the ArrayList
constructor or the Stream API might incur additional overhead due to the creation of new list instances. Careful consideration of the specific requirements and characteristics of the data being manipulated is crucial in determining the most appropriate approach from both functional and performance perspectives.
Final Thoughts
In summary, the process of converting an array to a list in Java is a common operation with several nuances to consider. While the Arrays.asList()
method provides a convenient solution, its limitations in terms of modifiability must be understood and navigated. The approach of utilizing the ArrayList
constructor or the Stream API presents effective alternatives, each with its respective merits and considerations, particularly concerning performance and modifiability.
By comprehensively understanding the implications and alternatives when converting an array to a list, Java developers can make informed decisions, ensuring code correctness, maintainability, and performance optimization.
In the ever-evolving landscape of Java programming, a thorough understanding of fundamental operations such as array to list conversion empowers developers to write robust, efficient, and maintainable code.
For further exploration of Java arrays and lists, consider diving into Oracle's documentation on Java Collections and the official Java documentation for Arrays.
Stay tuned for more insightful Java programming articles!