Mastering Time: Tackle Latency in Apache Flink Streams
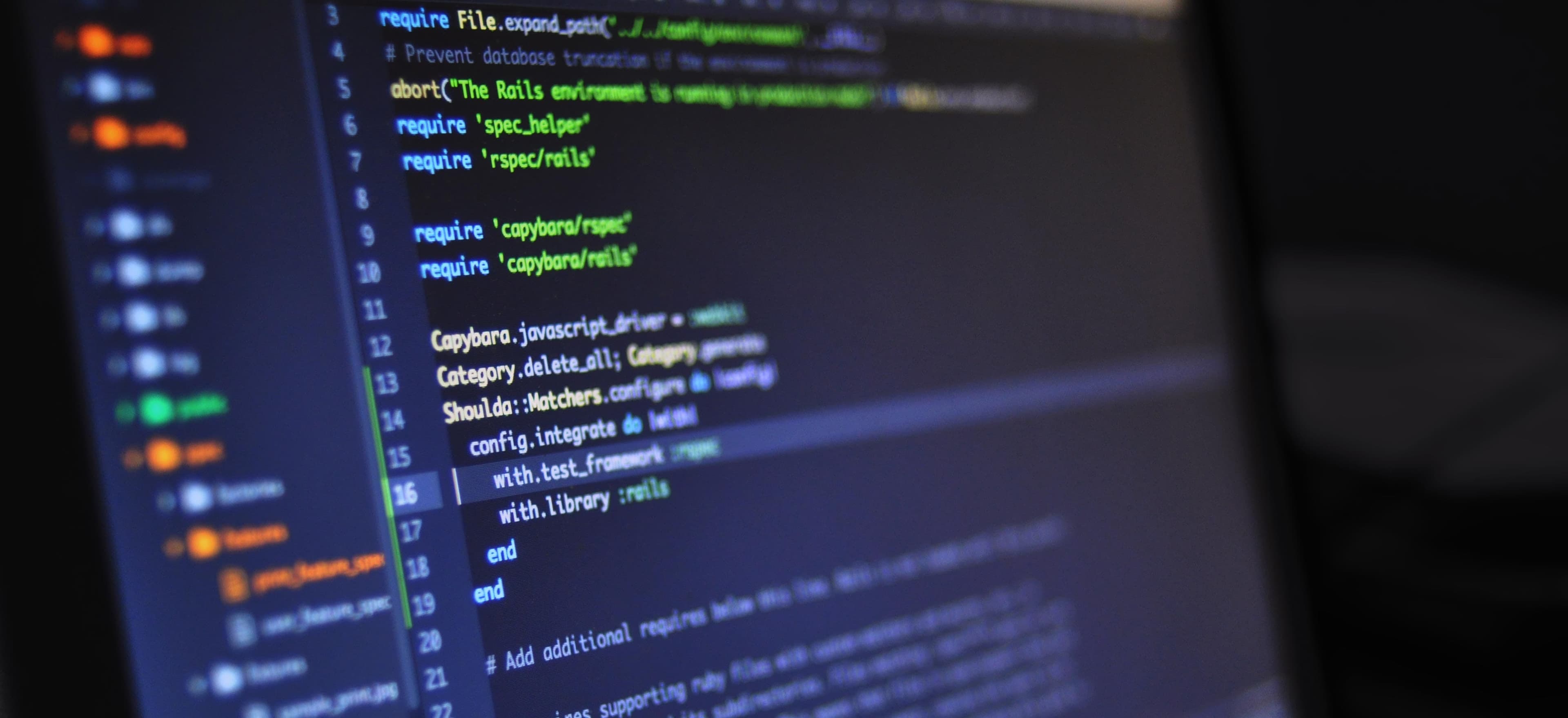
- Published on
Mastering Time: Tackle Latency in Apache Flink Streams
When it comes to real-time data processing, minimizing latency is crucial. Apache Flink, a powerful and scalable stream processing framework, provides robust features to handle event time and processing time. In this article, we'll delve into the concept of time in Apache Flink, understand the challenges of dealing with latency, and explore strategies to tackle latency in Flink streams.
Understanding Time in Apache Flink
Apache Flink introduces the distinction between event time and processing time to effectively handle streaming data. Event time refers to the time when an event actually occurred, while processing time denotes the time when an event is processed by the system. This differentiation is vital for accurate computations, especially when dealing with out-of-order events and delayed data arrivals.
Challenges of Latency in Stream Processing
Latency in stream processing can lead to inaccurate results and hinder the real-time analytics capabilities of a system. Several factors contribute to latency, including network delays, processing bottlenecks, and out-of-order event arrivals. Dealing with these challenges is imperative for ensuring the reliability and timeliness of data processing in Apache Flink applications.
Strategies to Tackle Latency
1. Watermarking
In Apache Flink, watermarks are used to track the progress of event time and handle delayed data. Watermarks indicate a certain time beyond which no more events are expected to arrive, allowing the system to process event time-based operations effectively. By incorporating watermarks into the Flink job, you can make informed decisions about event completeness and handle late data gracefully.
// Example of watermark generation in Flink
DataStream<T> stream = ...;
stream.assignTimestampsAndWatermarks(new BoundedOutOfOrdernessTimestampExtractor<T>(Time.seconds(5)) {
@Override
public long extractTimestamp(T element) {
return element.getTimestamp();
}
});
2. Windowing
Utilizing windowing techniques in Apache Flink enables the grouping of events based on time or other criteria, facilitating efficient processing and aggregation. By defining windows and applying functions such as tumbling, sliding, or session windows, you can manage event streams effectively and mitigate the impact of latency on computations.
// Example of tumbling window aggregation in Flink
DataStream<T> stream = ...;
stream.keyBy(...)
.window(TumblingEventTimeWindows.of(Time.minutes(1)))
.apply(new MyWindowFunction());
3. Monitoring and Optimization
Monitoring the latency metrics and resource utilization within Apache Flink applications is critical for identifying bottlenecks and optimizing performance. Tools like Flink's built-in metrics system and external monitoring solutions can provide insights into the processing pipeline, allowing you to fine-tune the job for improved latency handling.
Lessons Learned
Efficiently managing latency in Apache Flink streams is essential for achieving real-time processing capabilities and accurate data analytics. By leveraging features such as watermarks, windowing, and comprehensive monitoring, developers can tackle latency effectively and build robust stream processing applications.
In conclusion, mastering time in Apache Flink is key to conquering latency and unleashing the full potential of real-time data processing.
To dive deeper into Apache Flink and explore advanced time handling techniques, refer to the official documentation.