Effective Ways to Schedule Recurring Tasks in Java
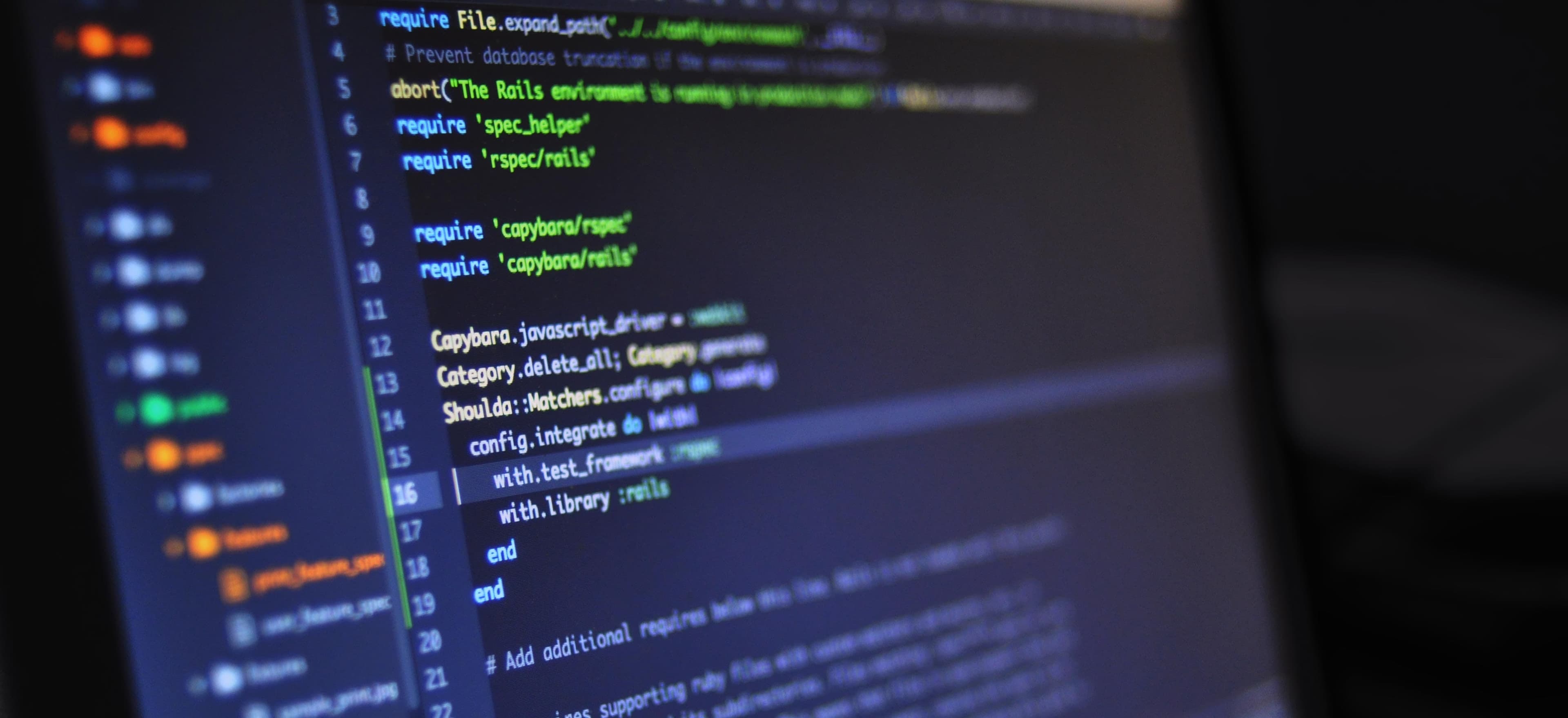
- Published on
Effective Ways to Schedule Recurring Tasks in Java
Scheduling recurring tasks is a fundamental requirement in many software applications. In Java, there are several ways to achieve this, each with its own strengths and use cases. In this post, we will explore some effective methods for scheduling recurring tasks in Java, including built-in APIs and third-party libraries.
1. java.util.Timer
and java.util.TimerTask
The java.util.Timer
and java.util.TimerTask
classes provide a simple way to schedule recurring tasks. You can create a Timer
and schedule a TimerTask
to be executed at fixed intervals. Here's an example:
import java.util.Timer;
import java.util.TimerTask;
public class ScheduledTaskExample {
public static void main(String[] args) {
Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
// Your task logic here
}
};
timer.schedule(task, 0, 1000); // Schedule the task to run every 1 second
}
}
One advantage of using Timer
and TimerTask
is their simplicity. However, they have limitations, such as not being suitable for long-running tasks and not handling exceptions gracefully.
2. java.util.concurrent.ScheduledExecutorService
The ScheduledExecutorService
interface in the java.util.concurrent
package provides a more flexible and robust way to schedule recurring tasks. It allows you to schedule tasks to execute after a certain delay or at fixed intervals. Here's an example:
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class ScheduledTaskExample {
public static void main(String[] args) {
ScheduledExecutorService executor = Executors.newScheduledThreadPool(1);
executor.scheduleAtFixedRate(() -> {
// Your task logic here
}, 0, 1, TimeUnit.SECONDS); // Schedule the task to run every 1 second
}
}
The ScheduledExecutorService
offers better control over the scheduling of tasks, exception handling, and support for long-running tasks. It is the recommended approach for scheduling tasks in modern Java applications.
3. Quartz Scheduler
Quartz is a popular third-party library for scheduling recurring tasks in Java. It provides a rich set of features for job scheduling, including cron-like expressions for more complex scheduling requirements. Here's an example of scheduling a job with Quartz:
import org.quartz.*;
import org.quartz.impl.StdSchedulerFactory;
public class QuartzSchedulerExample {
public static void main(String[] args) throws SchedulerException {
Scheduler scheduler = StdSchedulerFactory.getDefaultScheduler();
JobDetail job = JobBuilder.newJob(YourJob.class).build();
Trigger trigger = TriggerBuilder.newTrigger()
.withSchedule(SimpleScheduleBuilder.repeatSecondlyForever(1))
.build();
scheduler.start();
scheduler.scheduleJob(job, trigger);
}
public static class YourJob implements Job {
@Override
public void execute(JobExecutionContext context) throws JobExecutionException {
// Your task logic here
}
}
}
Quartz is suitable for enterprise-level applications with complex scheduling requirements. It provides features such as job persistence, clustering, and job chaining.
The Closing Argument
In Java, there are multiple ways to schedule recurring tasks, each with its own strengths and use cases. For simple tasks, java.util.Timer
and java.util.TimerTask
can be used, but for more robust and flexible task scheduling, java.util.concurrent.ScheduledExecutorService
is the recommended approach. Additionally, for enterprise-level applications with complex scheduling requirements, Quartz Scheduler is a powerful choice.
Choosing the right method for scheduling recurring tasks depends on the specific requirements of your application. Consider factors such as simplicity, flexibility, and scalability when making your decision.
By understanding and leveraging these scheduling techniques, you can effectively manage recurring tasks in your Java applications, ensuring that they run reliably and efficiently over time.
Remember to select the most suitable approach for your specific use case and always consider the trade-offs between simplicity and complexity to maintain an effective and manageable codebase.
Checkout our other articles