Streamline Your Testing: JUnit, Selenium & Gradle Integration
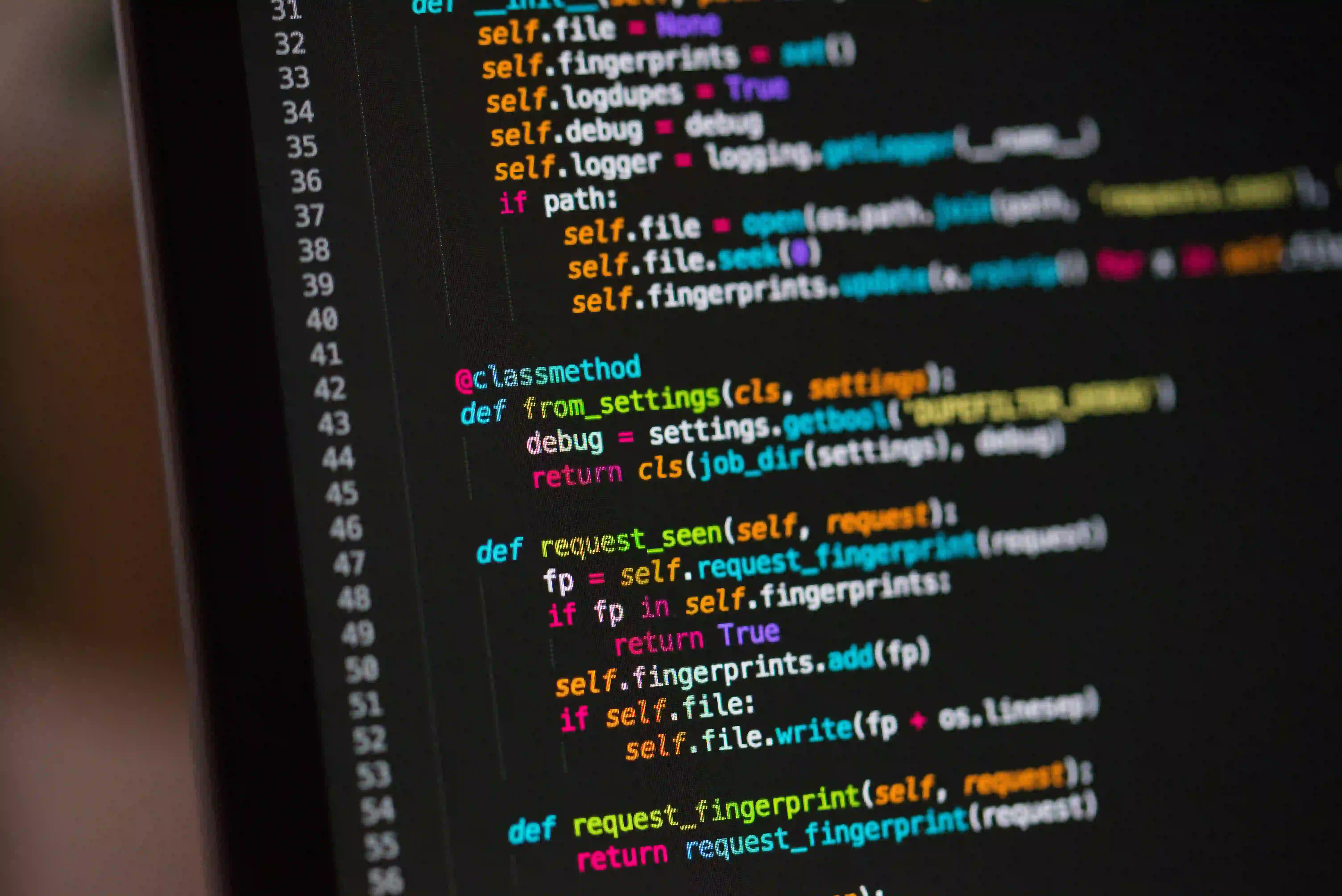
Streamline Your Testing: JUnit, Selenium & Gradle Integration
Testing is an integral part of the software development process, ensuring that your code functions as expected and continues to do so as it evolves. In Java development, leveraging tools like JUnit for unit testing and Selenium for automated UI testing can enhance the quality and reliability of your code. Furthermore, integrating these tools with Gradle, a powerful build automation system, can simplify the testing process and improve the efficiency of your workflow.
In this article, we will explore the seamless integration of JUnit, Selenium, and Gradle to streamline your testing processes, from writing and running tests to automating UI tests using Selenium, all within the Gradle build system.
Setting Up JUnit with Gradle
JUnit is a widely-used testing framework for Java, designed to perform unit testing. Integrating JUnit with Gradle is straightforward and offers a structured approach to manage and execute tests as part of the build lifecycle. Let's dive into integrating JUnit with Gradle.
First, ensure that the testCompile
dependency for JUnit is included in the build.gradle
file:
dependencies {
testCompile 'junit:junit:4.12'
}
With JUnit set up, you can write your test classes and methods, and Gradle will automatically recognize and execute them as part of the build process.
Integrating Selenium for UI Testing
UI testing is crucial in ensuring the functionality and performance of web applications. Selenium, a powerful tool for automating web browsers, provides a robust framework for UI testing. Let's integrate Selenium with Gradle to streamline UI testing within our build process.
To use Selenium with Gradle, we need to include the WebDriver dependency in the build.gradle
file:
dependencies {
testCompile 'org.seleniumhq.selenium:selenium-java:3.141.59'
}
We can then create Selenium test classes to automate UI interactions and validations as part of our testing suite.
Writing JUnit Tests
Now that we have JUnit and Selenium integrated with Gradle, let's discuss writing tests using these frameworks. JUnit tests typically follow a structured approach, using annotations such as @Test
to denote test methods and leveraging assertions to validate expected behavior.
Consider the following example of a simple JUnit test class:
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calc = new Calculator();
int result = calc.add(3, 4);
assertEquals(7, result);
}
}
In this example, the @Test
annotation marks the testAddition
method as a test case. We create an instance of the Calculator
class, perform an addition operation, and use the assertEquals
method to assert that the result matches the expected value.
This demonstrates the simplicity and clarity of writing JUnit tests to validate specific functionality within your code.
Automating UI Tests with Selenium
When it comes to UI testing, Selenium offers a powerful solution for automating interactions with web applications. Leveraging Selenium with JUnit within the Gradle build system allows for seamless integration and execution of UI tests alongside other testing processes.
Let's consider an example of a Selenium test that automates a login scenario on a web application:
import org.junit.Test;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
import static org.junit.Assert.assertEquals;
public class LoginTest {
@Test
public void testLogin() {
System.setProperty("webdriver.chrome.driver", "path_to_chromedriver");
WebDriver driver = new ChromeDriver();
driver.get("https://example.com/login");
// Perform login actions
// Assert login success
driver.quit();
}
}
In this example, we instantiate a Chrome WebDriver, navigate to the login page, perform login actions (such as entering credentials and clicking the login button), and assert the expected outcome.
Running Tests with Gradle
Executing the tests within the Gradle build process is seamless and can be achieved using the test
task. Simply run the following command in your terminal:
./gradlew test
Gradle will automatically execute both the JUnit and Selenium tests, providing concise feedback on test results and overall build status.
Final Thoughts
Incorporating JUnit, Selenium, and Gradle into your testing workflow can significantly streamline the testing process, from unit testing individual components to automating UI interactions. The seamless integration of these tools within the Gradle build system enhances the efficiency and reliability of your testing efforts.
By following the steps outlined in this article, you can create a robust testing environment that ensures the functionality and stability of your Java applications. Embracing these tools not only improves the quality of your code but also fosters a culture of confidence in the reliability of your software.
Start integrating JUnit, Selenium, and Gradle into your testing workflow today, and experience the benefits of streamlined testing and enhanced code quality.