Why Your Java Program's Multithreading Might Fail
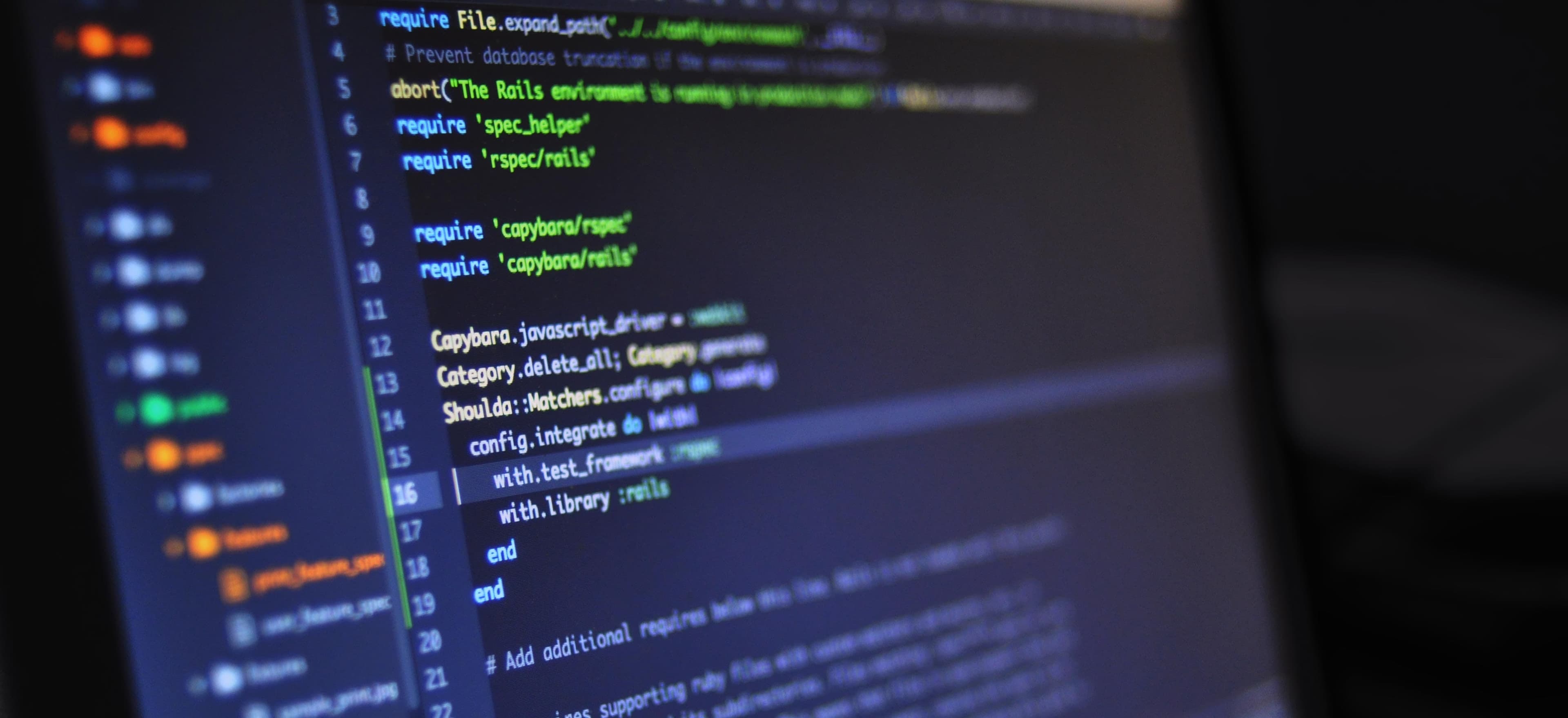
- Published on
Why Your Java Program's Multithreading Might Fail: A Comprehensive Guide
Multithreading in Java is a powerful tool that allows developers to write highly efficient and concurrent programs. However, architecting your Java applications to effectively utilize multithreading can be quite tricky. From deadlocks to race conditions, several pitfalls can hamper your program’s performance, or worse, cause unpredictable behavior. In this detailed guide, we'll explore why your Java program's multithreading might fail and how you can avoid these common pitfalls to create robust and efficient applications.
Understanding Java Multithreading
Before diving into the common issues, let’s briefly understand what multithreading in Java means. Multithreading is a Java feature that allows concurrent execution of two or more parts of a program for maximum utilization of CPU. Each part of such a program is called a thread, and each thread defines a separate path of execution. This is achieved by the java.lang.Thread
class or implementing the java.lang.Runnable
interface.
Multithreading is a sought-after feature for any programmer looking to improve application performance. Why? Because it allows you to perform complicated tasks without waiting for other tasks to finish, effectively reducing the overall execution time of your program. For a deeper dive into Java threads and Multithreading, I recommend reading the Java documentation on concurrency.
However, while multithreading can offer significant performance improvements, it also introduces complexity. Without proper management, this complexity can lead to various issues. Let's discuss these in detail.
1. Deadlocks
Deadlocks occur in a scenario where two or more threads are blocked forever, waiting for each other. This happens when multiple threads need the same locks but obtain them in a different order.
How to Avoid Deadlocks:
- Avoid Nested Locks: Always try to avoid giving a thread another lock when it already holds one.
- Use a Lock Ordering: Establish a global order in which locks are acquired and always acquire all locks in the provided order.
Example:
// Bad Practice: Nested Locks leading to potential deadlock
synchronized(lock1) {
synchronized(lock2) {
// Critical section code
}
}
// Good Practice: Using a single block where possible
synchronized(this) {
// Critical section code
}
2. Race Conditions
A race condition occurs when two or more threads access shared data and at least one thread modifies it without proper synchronization, leading to unpredictable results.
How to Avoid Race Conditions:
- Use Synchronization: Ensure that shared resources are accessed in a synchronized block or method.
- Leverage Concurrency API: Java’s
java.util.concurrent
package provides several utilities that facilitate managing shared resources more safely and effectively.
Example:
// Vulnerable to race conditions
public void increment() {
count++; // Non-synchronized access to shared mutable state
}
// Thread-safe via synchronized method
public synchronized void safeIncrement() {
count++; // Synchronized access, ensuring thread safety
}
3. Thread Starvation
Thread starvation happens when a thread is perpetually denied access to resources or the CPU. This most commonly occurs in thread pool scenarios or with incorrect use of thread priorities.
How to Avoid Thread Starvation:
- Use Fair Locks: Java’s
ReentrantLock
class allows you to create fair locks that abide by the order of locking request, reducing the chances of starvation. - Proper Thread Pool Management: Ensure that the thread pool size is optimized not to exceed the system’s handling capacity, and use executor services to manage the thread lifecycle efficiently.
Example Using Fair Lock:
ReentrantLock fairLock = new ReentrantLock(true); // Fair Lock
fairLock.lock();
try {
// Critical section protected by fair lock
} finally {
fairLock.unlock();
}
4. Memory Consistency Errors
Memory consistency errors occur due to inconsistent views of shared memory when different threads see different values for the same variable.
How to Avoid Memory Consistency Errors:
- Use
volatile
Keyword: It ensures that changes to a variable are propagated predictably to other threads. - Use Atomic Classes: Java provides classes in the
java.util.concurrent.atomic
package that support lock-free, thread-safe programming on single variables.
Example Using Volatile:
volatile boolean flag = true;
// Thread 1
public void run() {
while(flag) {
// Do work
}
}
// Thread 2
public void stop() {
flag = false;
}
Best Practices and Tools
Understanding these pitfalls and implementing the strategies mentioned can significantly reduce multithreading issues. Additionally, leveraging tools like FindBugs or PMD can help identify potential thread safety concerns in your code. For more insights into best practices for multithreading in Java, visiting the official Java tutorials can be immensely beneficial.
Lessons Learned
Multithreading in Java can dramatically increase the performance of your applications. However, without careful design and consideration, multithreading can introduce hard-to-debug issues into your application. By understanding the common causes of failure in Java’s multithreading mentioned above and implementing the suggested solutions, you can harness the power of concurrent programming while mitigating its risks.
Remember, mastering Java's multithreading requires patience and practice. Keep experimenting, learning from mistakes, and staying updated with Java's concurrency utilities and best practices. Happy coding!