Overcoming the Pitfalls of Insufficient Defensive Programming
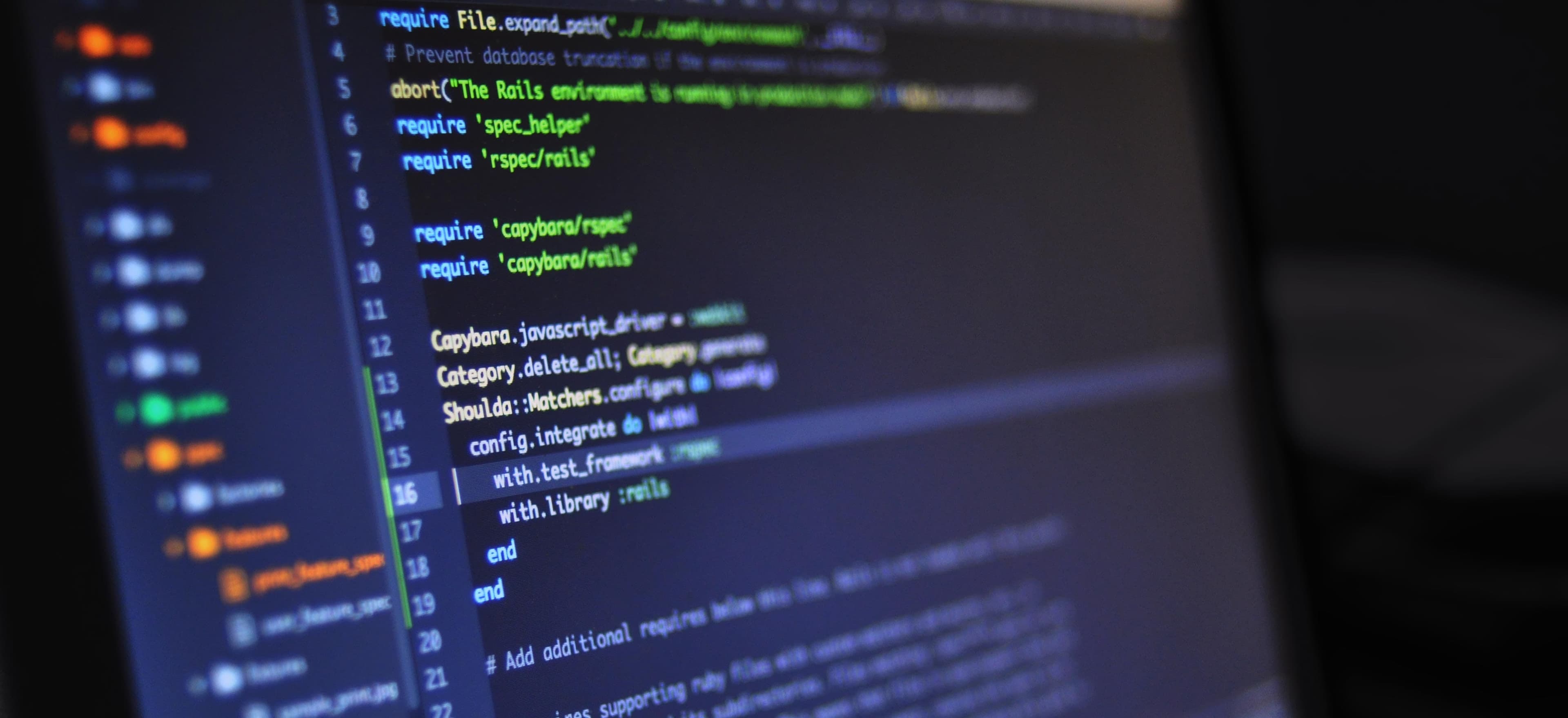
- Published on
Overcoming the Pitfalls of Insufficient Defensive Programming
Defensive programming is a critical concept in software development, especially in Java, where robustness and reliability are paramount. It involves writing code that anticipates and defends against potential failures, ensuring that the software behaves predictably and gracefully in unexpected situations.
In this article, we'll explore the common pitfalls of insufficient defensive programming in Java and learn how to overcome them. By the end, you'll have a solid understanding of the best practices for implementing defensive programming in your Java projects.
Pitfall 1: Null Pointer Exceptions
In Java, null pointer exceptions are among the most common runtime errors. They occur when a program attempts to use a reference that points to null. This can happen when a method returns null instead of an expected object, or when developers forget to check for null before accessing an object's methods or properties.
To address this issue, defensive programming techniques such as null checks and Optional types should be employed. Let's take a look at how this can be achieved in Java:
public String processString(String input) {
if (input != null) {
return input.toLowerCase();
}
return ""; // or throw an exception as appropriate
}
In the example above, we check if the input string is not null before calling the toLowerCase()
method. This simple null check can prevent a null pointer exception from occurring.
Pitfall 2: Array Index Out of Bounds
Another common pitfall is accessing elements beyond the bounds of an array. It can lead to ArrayIndexOutOfBoundsException, causing the program to terminate unexpectedly.
Defensive programming can help prevent this issue by validating array indices before accessing elements. Here's an example:
public void processArray(int[] arr, int index) {
if (index >= 0 && index < arr.length) {
int value = arr[index];
// Process the value
} else {
// Handle out of bounds condition
}
}
In the above code, we check if the index is within the bounds of the array before accessing the element at that index, thereby avoiding potential ArrayIndexOutOfBoundsException.
Pitfall 3: Resource Leaks
Failure to release resources such as file handles, database connections, or network sockets can lead to resource leaks, ultimately impacting the performance and stability of the application.
Defensive programming, in this case, involves proper resource management using try-with-resources or try-finally blocks to ensure that resources are released regardless of whether an exception occurs. Let's see an example:
public void processFile(String filePath) {
try (FileReader reader = new FileReader(filePath);
BufferedReader bufferedReader = new BufferedReader(reader)) {
// Read and process the file content
} catch (IOException e) {
// Handle the exception
}
}
In this code snippet, the try-with-resources statement ensures that the FileReader and BufferedReader are properly closed after the processing, preventing resource leaks.
Pitfall 4: Unhandled Exceptions
Unchecked exceptions that propagate up the call stack without being caught and handled can disrupt the normal flow of the program and lead to unexpected termination.
Defensive programming involves catching and handling exceptions at an appropriate level to maintain the stability of the application. Consider the following example:
public void performOperation() {
try {
// Code that may potentially throw an exception
} catch (SpecificException ex) {
// Handle the specific exception
} catch (Exception e) {
// Handle other exceptions
} finally {
// Perform clean-up activities if necessary
}
}
In this case, the try-catch-finally block ensures that exceptions are caught, handled, and appropriate clean-up activities are performed, contributing to the overall robustness of the software.
Pitfall 5: Insecure Input Handling
Insufficient validation and sanitization of user input can lead to security vulnerabilities such as injection attacks, cross-site scripting, and more.
Defensive programming involves validating and sanitizing input data to prevent such vulnerabilities. Utilizing libraries like OWASP ESAPI or frameworks like Spring Security can provide robust input validation and protection from common security threats.
Key Takeaways
In conclusion, defensive programming is crucial for building robust and reliable Java applications. By addressing common pitfalls such as null pointer exceptions, array index out of bounds errors, resource leaks, unhandled exceptions, and insecure input handling, developers can significantly improve the quality and resilience of their software.
Adopting defensive programming practices not only enhances the stability of the application but also contributes to a better user experience and overall security. It's essential to integrate these techniques into the software development process from the outset, ensuring that defensive programming becomes a fundamental aspect of Java development.
By applying the principles and examples discussed in this article, developers can proactively address potential pitfalls and strengthen their Java code, delivering more stable and secure software solutions.
Remember, defensive programming is not about making your code infallible, but about anticipating and handling potential failures with grace and resilience.
Start integrating defensive programming into your Java projects today to build software that is not only functional and performant but also robust and reliable.