Revolutionize Coding: JDK 11's New Collection Method Explained
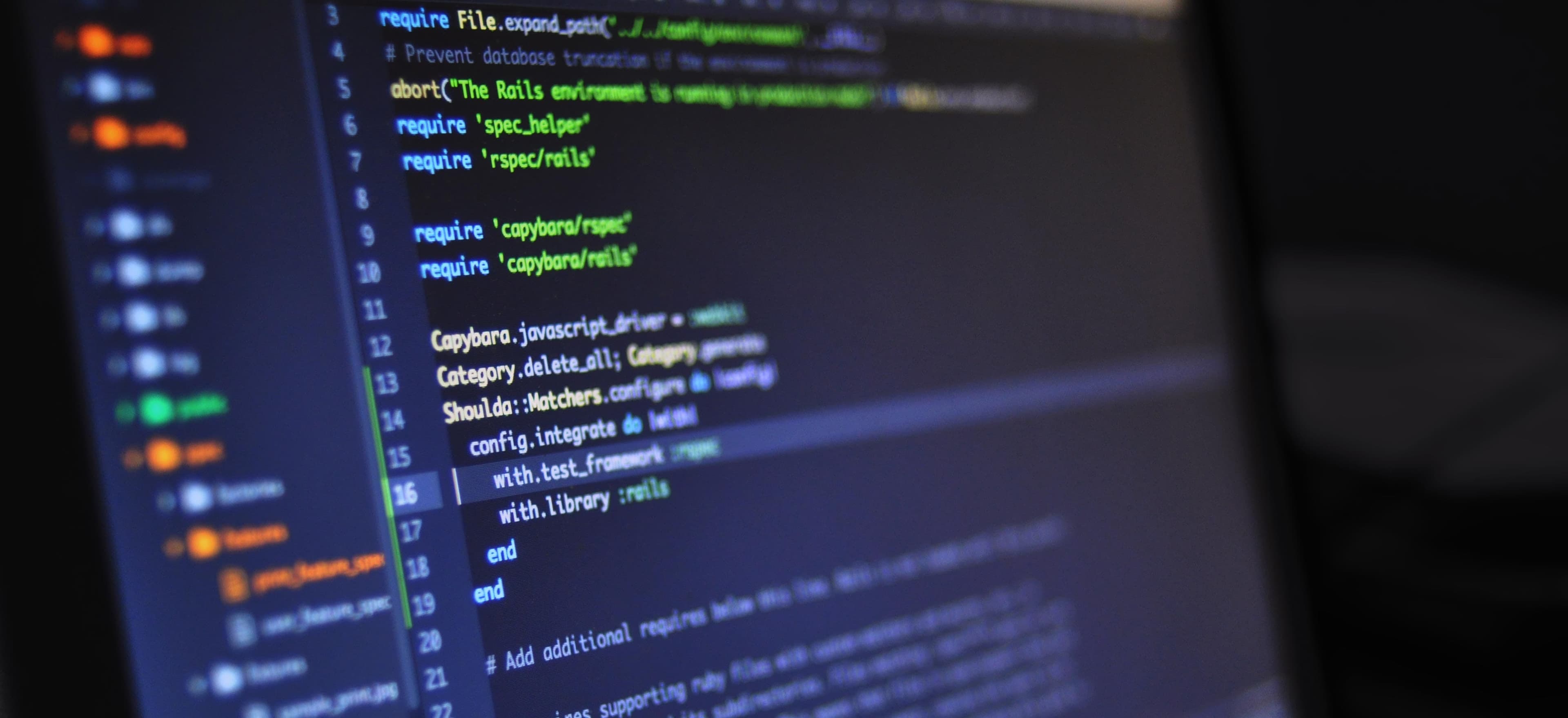
- Published on
Revolutionize Coding: JDK 11's New Collection Method Explained
Java 11, the latest LTS (Long Term Support) version, brings in several exciting features to the table. Among these features, one that stands out is the introduction of the Collectors
class method toUnmodifiableList()
, toUnmodifiableSet()
, and toUnmodifiableMap()
. These new methods provide a seamless way to create unmodifiable collections, thus enhancing the robustness and reliability of your code. In this article, we will explore these new methods, understand their significance, and grasp their implementation through code examples.
The Need for Unmodifiable Collections
In Java, collections are an integral part of the language, serving as containers for storing and manipulating groups of objects. While working with collections, it is often necessary to ensure that the collection remains unmodifiable, i.e., its contents cannot be altered after its creation. This is imperative in scenarios where immutability is desired, such as protecting the integrity of shared data structures, creating safe copies of collections, or ensuring thread safety in concurrent programming.
In earlier versions of Java, achieving unmodifiable collections required verbose and error-prone code, typically involving the use of the Collections.unmodifiableXXX()
methods. With the advent of Java 11, this process has been greatly simplified through the introduction of the toUnmodifiableX()
methods in the Collectors
class.
Getting Acquainted with toUnmodifiableList(), toUnmodifiableSet(), and toUnmodifiableMap()
The toUnmodifiableList()
, toUnmodifiableSet()
, and toUnmodifiableMap()
methods are added to the Collectors
class, which is a utility class that provides implementations of the Collector
interface. These methods are static and facilitate the creation of unmodifiable lists, sets, and maps respectively, using the Collectors
API, which is widely used in Java streams for reduction operations.
toUnmodifiableList()
The toUnmodifiableList()
method returns a Collector
that accumulates the input elements into an unmodifiable list in encounter order. Let's dive into a code example to see its usage in action:
import java.util.List;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class UnmodifiableListExample {
public static void main(String[] args) {
Stream<String> stream = Stream.of("Java", "is", "awesome");
List<String> unmodifiableList = stream.collect(Collectors.toUnmodifiableList());
// Attempting to modify the list will result in UnsupportedOperationException
unmodifiableList.add("!");
// Output: Exception in thread "main" java.lang.UnsupportedOperationException
}
}
In the above code, the toUnmodifiableList()
method creates an unmodifiable list from the elements of the input stream. Any attempt to modify this list will result in an UnsupportedOperationException
, thus ensuring its immutability.
toUnmodifiableSet()
Similar to toUnmodifiableList()
, the toUnmodifiableSet()
method returns a Collector
that accumulates the input elements into an unmodifiable set. Here's a concise demonstration of its usage:
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class UnmodifiableSetExample {
public static void main(String[] args) {
Stream<Integer> stream = Stream.of(1, 2, 3);
Set<Integer> unmodifiableSet = stream.collect(Collectors.toUnmodifiableSet());
// Attempting to add elements to the set will throw UnsupportedOperationException
unmodifiableSet.add(4);
// Output: Exception in thread "main" java.lang.UnsupportedOperationException
}
}
Once again, the resulting set, created using toUnmodifiableSet()
, is impervious to modifications, safeguarding its immutability.
toUnmodifiableMap()
The toUnmodifiableMap()
method produces a Collector
that accumulates elements into an unmodifiable map. Behold its usage in a practical example:
import java.util.Map;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class UnmodifiableMapExample {
public static void main(String[] args) {
Stream<String> stream = Stream.of("one", "two", "three");
Map<Integer, String> unmodifiableMap = stream.collect(Collectors.toUnmodifiableMap(String::length, s -> s));
// Attempting to modify the map will result in UnsupportedOperationException
unmodifiableMap.put(4, "four");
// Output: Exception in thread "main" java.lang.UnsupportedOperationException
}
}
In this case, the toUnmodifiableMap()
method seamlessly creates an unmodifiable map, posing restrictions on any attempts to alter its contents.
Why Should You Embrace toUnmodifiableX() Methods?
The introduction of the toUnmodifiableList()
, toUnmodifiableSet()
, and toUnmodifiableMap()
methods not only simplifies the process of creating unmodifiable collections but also promotes code readability and maintainability. The significance of these methods can be summarized as follows:
-
Immutability: The resulting unmodifiable collections offer immutability by preventing modifications to their contents, thereby ensuring data integrity and consistency.
-
Conciseness: The usage of these methods eliminates the need for boilerplate code traditionally required to achieve immutability, resulting in cleaner and more compact code.
-
Fail-fast Behavior: Attempts to modify these unmodifiable collections result in an immediate
UnsupportedOperationException
, aiding in quickly detecting and rectifying inadvertent mutative operations. -
Thread Safety: Unmodifiable collections are inherently thread-safe, making them ideal for concurrent programming scenarios.
Getting Started with Java 11 and Beyond
JDK 11's new toUnmodifiableX()
methods in the Collectors
class herald a paradigm shift in the way unmodifiable collections are created and utilized in Java. The simplicity, clarity, and effectiveness of these methods make them a valuable addition to every Java developer's toolkit. As the tech ecosystem continues to evolve, staying updated with the latest versions and features of Java is paramount for harnessing its true potential.
Whether you are exploring the latest enhancements in Java or diving into the world of programming for the first time, Java's rich ecosystem and comprehensive documentation make it an excellent choice for building robust and scalable software solutions.
As you continue to elevate your Java skills, keep an eye on upcoming JDK releases and the ever-expanding Java ecosystem, and embrace the possibilities they offer for revolutionizing your coding journey.
In conclusion, Java 11's new collection method, particularly the toUnmodifiableX()
methods, empowers developers to elevate their code's reliability, readability, and robustness by simplifying the creation of unmodifiable collections. Embracing these methods not only streamlines code implementation but also reinforces the best practices of immutability and thread safety, thus propelling Java development into a new era of efficiency and resilience.
Now, armed with the knowledge of these new methods, venture forth and revolutionize your coding practices with Java 11's enhanced capabilities.
For detailed information on the Collectors
class and the toUnmodifiableX()
methods, refer to the official Java documentation.
Start integrating these new methods into your Java projects, and experience the transformative power of Java 11's unmodifiable collections firsthand. Happy coding!