Conquering Stagnant Sprints With Product Burndown Charts
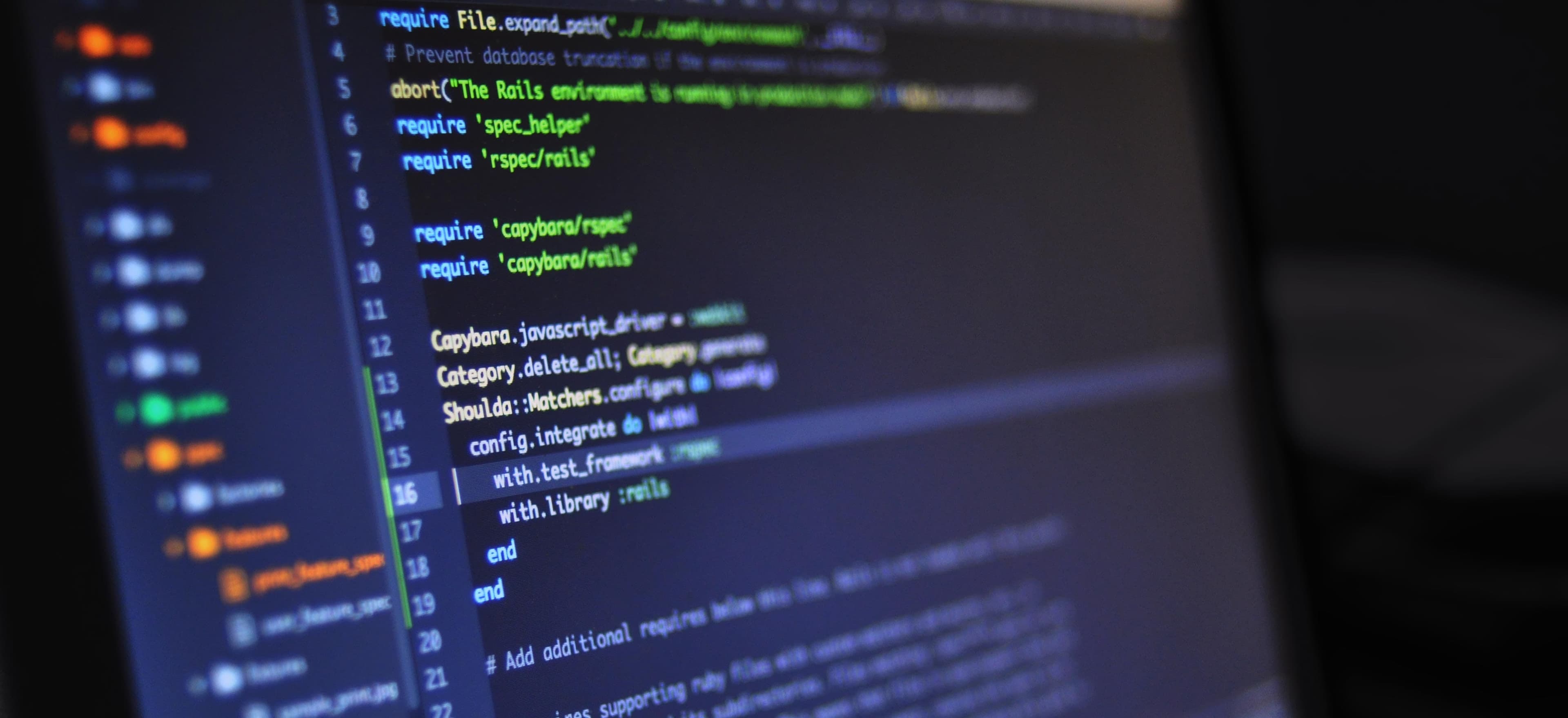
- Published on
Conquering Stagnant Sprints With Product Burndown Charts
In the world of Agile software development, sprint stagnation can be a challenging hurdle to overcome. Despite meticulous planning and diligent effort, projects can sometimes hit roadblocks that impede progress. Leveraging the power of product burndown charts can provide valuable insights into the trajectory of a sprint, allowing teams to identify bottlenecks, make informed decisions, and ultimately drive towards successful sprint completion.
Understanding Product Burndown Charts
A product burndown chart is a visual representation of the amount of work remaining in a sprint. It enables teams to track the progress of completing user stories, tasks, or other work items over the course of a sprint. The horizontal axis typically represents the time, divided into increments such as days or weeks, while the vertical axis represents the amount of work remaining. As work is completed or added, the chart dynamically adjusts to reflect the changes, providing a clear picture of the remaining work and the sprint's projected completion.
The Role of Product Burndown Charts in Agile Development
Product burndown charts play a crucial role in Agile development by providing teams with real-time insights into the status of a sprint. By visualizing the remaining work and comparing it to the ideal trajectory line, teams can quickly identify deviations and take proactive measures to address them. This can include reprioritizing tasks, reallocating resources, or identifying and resolving impediments that hinder progress. Ultimately, the transparency and immediacy of information offered by product burndown charts empower teams to adapt and optimize their efforts, leading to more predictable and successful sprint outcomes.
Implementing Product Burndown Charts in Java
Now, let's dive into the implementation of product burndown charts in Java. We'll explore how to leverage the power of Java to create a simple yet effective product burndown chart for Agile development.
Step 1: Setting Up the Project
First, we'll create a new Java project or navigate to an existing one where we want to implement the product burndown chart functionality. We'll ensure that we have the necessary dependencies for chart generation, such as the JFreeChart library.
Step 2: Data Collection and Calculation
Next, we'll focus on collecting and processing the data needed to populate the product burndown chart. This may involve retrieving the remaining work from a task management system, calculating the ideal trajectory line based on the sprint duration, and organizing the data for chart creation.
// Sample code for data collection and calculation
public class BurndownData {
public List<Integer> remainingWorkPerDay;
public int totalSprintDays;
// Method to calculate ideal trajectory line
public List<Integer> calculateIdealTrajectory() {
List<Integer> idealTrajectory = new ArrayList<>();
int workPerDay = remainingWorkPerDay.get(0) / totalSprintDays;
for (int day = 0; day <= totalSprintDays; day++) {
int idealWorkRemaining = remainingWorkPerDay.get(0) - (workPerDay * day);
idealTrajectory.add(idealWorkRemaining);
}
return idealTrajectory;
}
}
In this snippet, we define a BurndownData
class to encapsulate the remaining work data and sprint duration. The calculateIdealTrajectory
method computes the ideal trajectory line by evenly distributing the remaining work across the sprint days.
Step 3: Chart Generation
With the data prepared, we can now proceed to generate the product burndown chart using the JFreeChart library. We'll create a method to visualize the remaining work and ideal trajectory line on the chart.
// Sample code for chart generation
public class BurndownChart {
public void generateChart(List<Integer> remainingWork, List<Integer> idealTrajectory, int totalSprintDays) {
// Code to create and customize the burndown chart using JFreeChart
// Omitted for brevity
}
}
In the BurndownChart
class, the generateChart
method takes the remaining work data, ideal trajectory line, and total sprint days as inputs to create the burndown chart. Customization of the chart layout, styles, and axes can be added as needed.
Step 4: Integration and Visualization
Finally, we'll integrate the chart generation functionality into our Agile development tool or reporting system to visualize the product burndown chart. This may involve triggering the chart generation at regular intervals or in response to updates in the remaining work data.
My Closing Thoughts on the Matter
Product burndown charts serve as invaluable tools for Agile teams, offering a clear and concise representation of sprint progress. By implementing product burndown charts in Java, teams can harness the power of visualization to pinpoint obstacles, adapt their strategies, and drive towards successful sprint completion. With real-time insights at their disposal, teams are empowered to steer sprints towards optimal outcomes, ensuring that Agile development stays true to its core principles of adaptability and continuous improvement.
Incorporating product burndown charts in Agile development can significantly enhance the project management process. Understanding the process of generating these charts can be the key to unlocking their potential for improving sprint efficiency and overall team productivity.
As you delve deeper into the realm of Agile development and Java programming, keep in mind that product burndown charts offer not just a visual representation of progress, but also a roadmap to success for Agile teams.
To further explore the importance of visualization in Agile development, check out this article on Agile metrics and gain a broader understanding of measuring Agile performance.
Remember, the journey to mastering Agile development and harnessing the power of Java is an ongoing process, and each new tool and technique you adopt will bring you closer to achieving your goals.
Checkout our other articles