Simplifying Code Complexity: Master The Decorator Pattern!
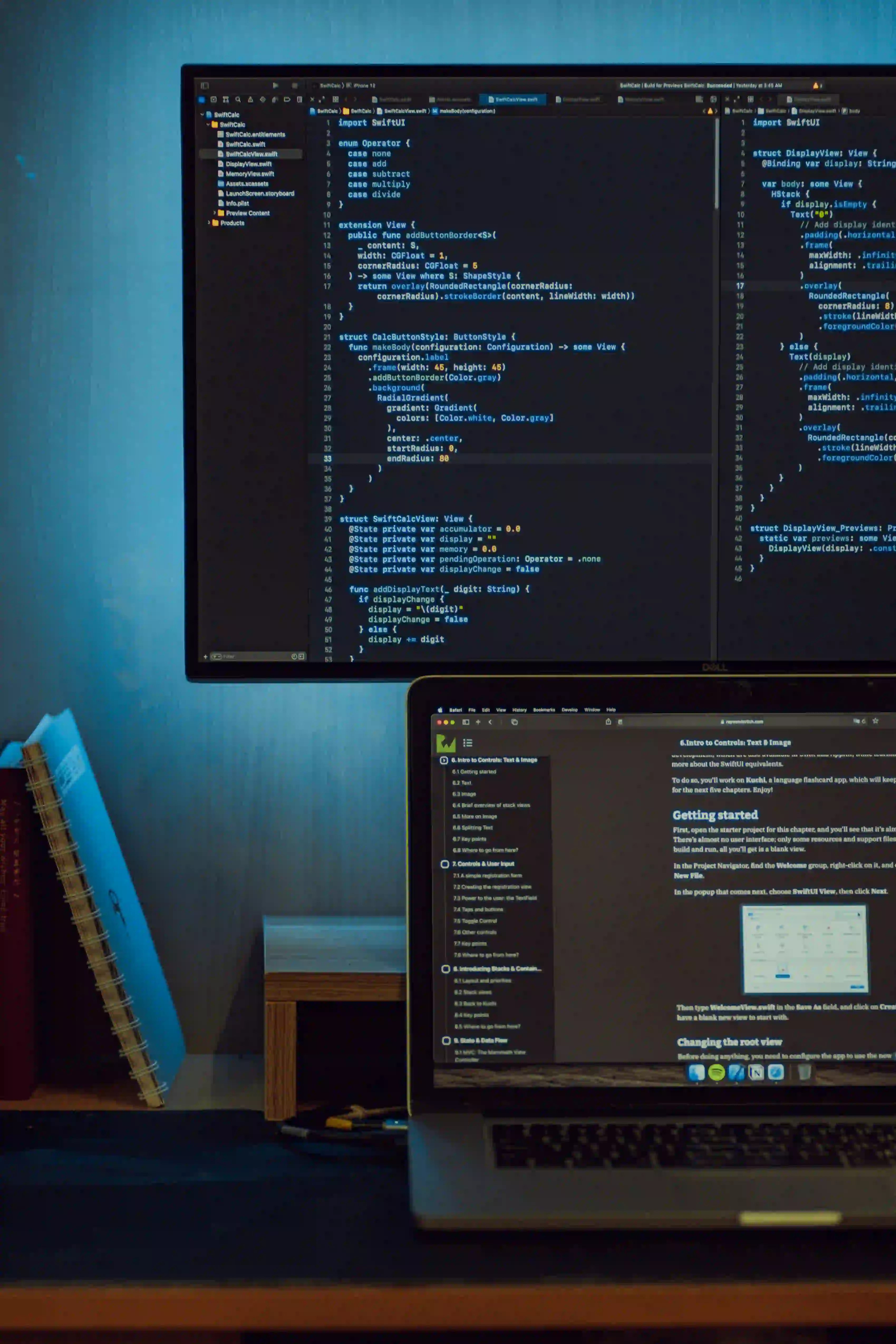
Simplifying Code Complexity: Master The Decorator Pattern!
In the world of object-oriented programming, it's crucial to have design patterns in your arsenal to simplify code complexity. One such pattern is the Decorator pattern, which allows behavior to be added to individual objects dynamically, providing a flexible alternative to subclassing for extending functionality.
Understanding the Decorator Pattern
At its core, the Decorator pattern attaches additional responsibilities to objects dynamically. This pattern is particularly useful when the need for subclassing to extend functionality leads to a combinatorial explosion of classes. Instead of defining multiple subclasses, each containing a particular combination of behavior, the Decorator pattern offers a more flexible and efficient solution.
The primary concept behind the Decorator pattern is to define a set of decorator classes that are used to wrap concrete components. These decorators serve as wrappers that modify the behavior of the component being decorated, without altering its structure.
The Anatomy of the Decorator Pattern
Let's delve into the basic components of the Decorator pattern:
-
Component Interface: This defines the interface for objects that can have responsibilities added to them dynamically.
-
Concrete Component: This is the class being wrapped by the decorators. It implements the Component interface and defines the core behavior.
-
Decorator: This is an abstract class that also implements the Component interface. It has a reference to an instance of the Component and provides an interface that conforms to the Component's interface.
-
Concrete Decorators: These are the classes that extend the Decorator class to add specific behaviors. Each Concrete Decorator adds its own unique behavior before and/or after delegating to the wrapped object.
Implementing the Decorator Pattern in Java
To illustrate the Decorator pattern in action, let's consider a simple example involving beverage customization. We'll start with a Beverage
interface representing the base component and a SimpleBeverage
class as the concrete component.
public interface Beverage {
String getDescription();
double cost();
}
public class SimpleBeverage implements Beverage {
@Override
public String getDescription() {
return "Simple Beverage";
}
@Override
public double cost() {
return 2.0;
}
}
In the example above, the SimpleBeverage
class serves as the core component, implementing the Beverage
interface with basic functionality.
Now, let's create the BeverageDecorator
abstract class, which implements the Beverage
interface and acts as the base for all concrete decorators.
public abstract class BeverageDecorator implements Beverage {
protected Beverage beverage;
public BeverageDecorator(Beverage beverage) {
this.beverage = beverage;
}
@Override
public String getDescription() {
return beverage.getDescription();
}
@Override
public double cost() {
return beverage.cost();
}
}
The BeverageDecorator
class provides a common interface for all concrete decorators to follow. It maintains a reference to a Beverage
object and delegates calls to it.
Next, let's create a concrete decorator called Milk
that adds the cost and description of milk to the beverage.
public class Milk extends BeverageDecorator {
public Milk(Beverage beverage) {
super(beverage);
}
@Override
public String getDescription() {
return beverage.getDescription() + ", Milk";
}
@Override
public double cost() {
return beverage.cost() + 0.5;
}
}
In the Milk
class, we extend the BeverageDecorator
and provide custom implementation for the getDescription
and cost
methods to add the specific behavior of adding milk to the beverage.
Leveraging the Decorator Pattern
With the Beverage
, SimpleBeverage
, BeverageDecorator
, and Milk
classes in place, we can now utilize the Decorator pattern to create and customize beverages without the need to create multiple subclasses or modify existing classes.
Beverage beverage = new SimpleBeverage();
System.out.println(beverage.getDescription() + ": $" + beverage.cost());
// Add milk to the beverage
beverage = new Milk(beverage);
System.out.println(beverage.getDescription() + ": $" + beverage.cost());
By employing the Decorator pattern, we were able to dynamically add the behavior of adding milk to the beverage without altering the core SimpleBeverage
class. This approach not only simplifies the code by eliminating the need for multiple subclasses but also allows for greater flexibility in combining different behaviors.
Benefits of the Decorator Pattern
The Decorator pattern offers several advantages, including:
-
Flexibility: It allows for the dynamic addition of new behaviors to objects at runtime, providing greater flexibility compared to static inheritance.
-
Simplicity: By using composition and delegation, it simplifies the codebase by avoiding the proliferation of subclasses for every combination of behaviors.
-
Open-Closed Principle: It adheres to the Open-Closed principle, enabling the addition of new behaviors without modifying existing code.
Key Takeaways
In conclusion, the Decorator pattern is a powerful tool for simplifying code complexity by enabling the dynamic addition of behaviors to objects. By leveraging composition and delegation, this pattern promotes flexibility, maintainability, and extensibility in object-oriented designs.
Understanding and applying the Decorator pattern can significantly enhance the elegance and scalability of your codebase, making it a valuable addition to your design pattern repertoire.
To delve deeper into design patterns and their practical implementation in Java, check out the official Java documentation and the seminal book "Design Patterns: Elements of Reusable Object-Oriented Software" by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides.
Master the Decorator pattern and unlock a new level of code elegance and flexibility in your Java projects!