Streamline Your SPA: Separate API & Resources for Efficiency
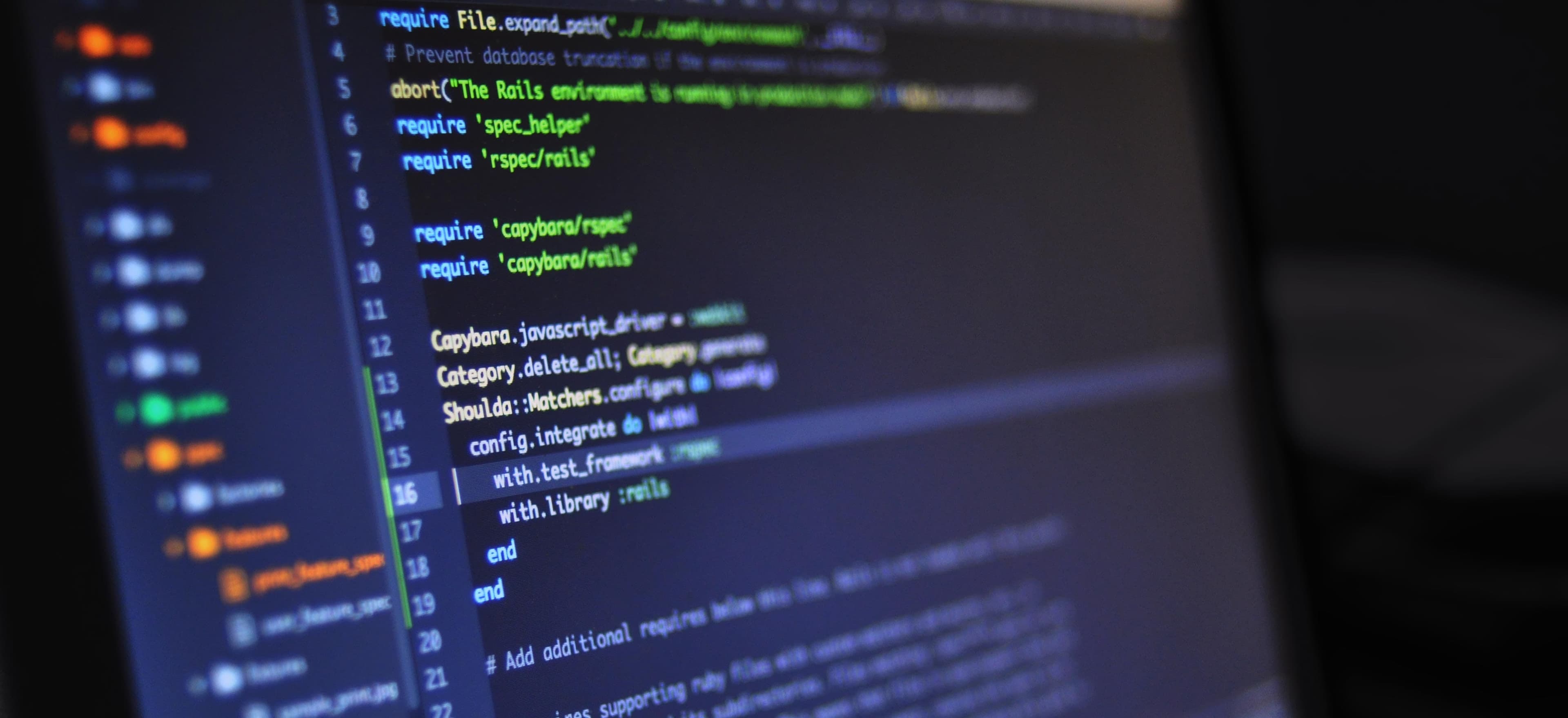
- Published on
Streamline Your Single Page Application: Separating API & Resources for Efficiency
In the modern web development landscape, Single Page Applications (SPAs) have gained immense popularity for their seamless user experience and dynamic content rendering. Java, with its robust backend capabilities, has become a prominent choice for building the API that powers these SPAs. However, in order to ensure optimal efficiency and maintainability, it's crucial to separate the API from the static resources of the SPA.
The Importance of Separation
When developing a SPA, it's common practice to build the frontend and backend as separate entities. This separation enables teams to work independently, promotes code reusability, and simplifies maintenance. By extending this concept to the division between the API and static resources, you can further enhance the efficiency of your application.
Separating the API
Separating the API involves creating a distinct Java application that serves as the backend for the SPA. This backend application is responsible for handling data manipulation, authentication, and business logic. By decoupling the API from the frontend resources, you create a clear boundary that allows for scalability, flexibility, and simplified testing.
Code Example: Separating API
// UserController.java
@RestController
@RequestMapping("/api/users")
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/{id}")
public ResponseEntity<User> getUserById(@PathVariable Long id) {
User user = userService.getUserById(id);
return ResponseEntity.ok(user);
}
// Additional API endpoints for user management
}
In the example above, the UserController
class represents the API endpoints for user-related operations. By separating this logic into a dedicated backend application, you can effectively manage user data without intertwining it with the frontend resources.
Separating Static Resources
On the other hand, the static resources of the SPA, such as HTML, CSS, and JavaScript files, are served by a separate web server or CDN. This approach ensures that the frontend assets are efficiently delivered to the client, benefiting from caching and content delivery networks. By decoupling the API and static resources, you create a more scalable and performant architecture for your SPA.
Code Example: Serving Static Resources
// WebConfig.java
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/**")
.addResourceLocations("classpath:/static/")
.setCacheControl(CacheControl.maxAge(365, TimeUnit.DAYS));
}
}
In the above code snippet, the WebConfig
class configures the resource handling for the static assets of the SPA. By separating the serving of static resources from the API, you can take advantage of browser caching and optimize the delivery of frontend assets.
Benefits of Separation
Improved Performance
Separating the API from the static resources allows for more efficient handling of client requests. The backend API can focus solely on processing data and business logic, while the frontend resources are optimized for speedy delivery to the client. This separation contributes to improved performance and a smoother user experience.
Enhanced Scalability
With a clear division between the API and static resources, you can independently scale each component based on specific requirements. This scalability empowers you to allocate resources where they are most needed, whether it's handling increased API traffic or serving a growing user base with the frontend resources.
Simplified Maintenance
Maintaining separate codebases for the API and static resources simplifies the development and maintenance process. Teams can work autonomously on frontend and backend components, streamlining the debugging, testing, and deployment activities. Any updates or modifications can be carried out with minimal impact on the overall application.
Closing Remarks
By separating the API and static resources, you can streamline the development and operation of your Single Page Application. This approach not only enhances performance and scalability but also simplifies maintenance and fosters a clear architectural distinction between the backend and frontend. Embracing this separation enables your Java-based SPA to operate with optimal efficiency and resilience in the ever-evolving digital landscape.
Integration of robust SPA frameworks like React, Angular, or Vue.js with a Java backend further solidifies the efficiency of this approach, ensuring seamless communication and data management between the frontend and backend. Embracing separation leads to a well-organized, easily maintainable, and robust architecture for modern web applications.
Implementing this strategy sets your application on a path toward enhanced performance, scalability, and maintainability, ensuring a seamless and efficient experience for your users and developers alike.
For further insights into improving the efficiency of your SPA, explore relevant resources on building scalable SPAs and separation of concerns in software architecture. Happy coding!
Checkout our other articles