Solving Fibonacci in Java: Functional vs Imperative Showdown
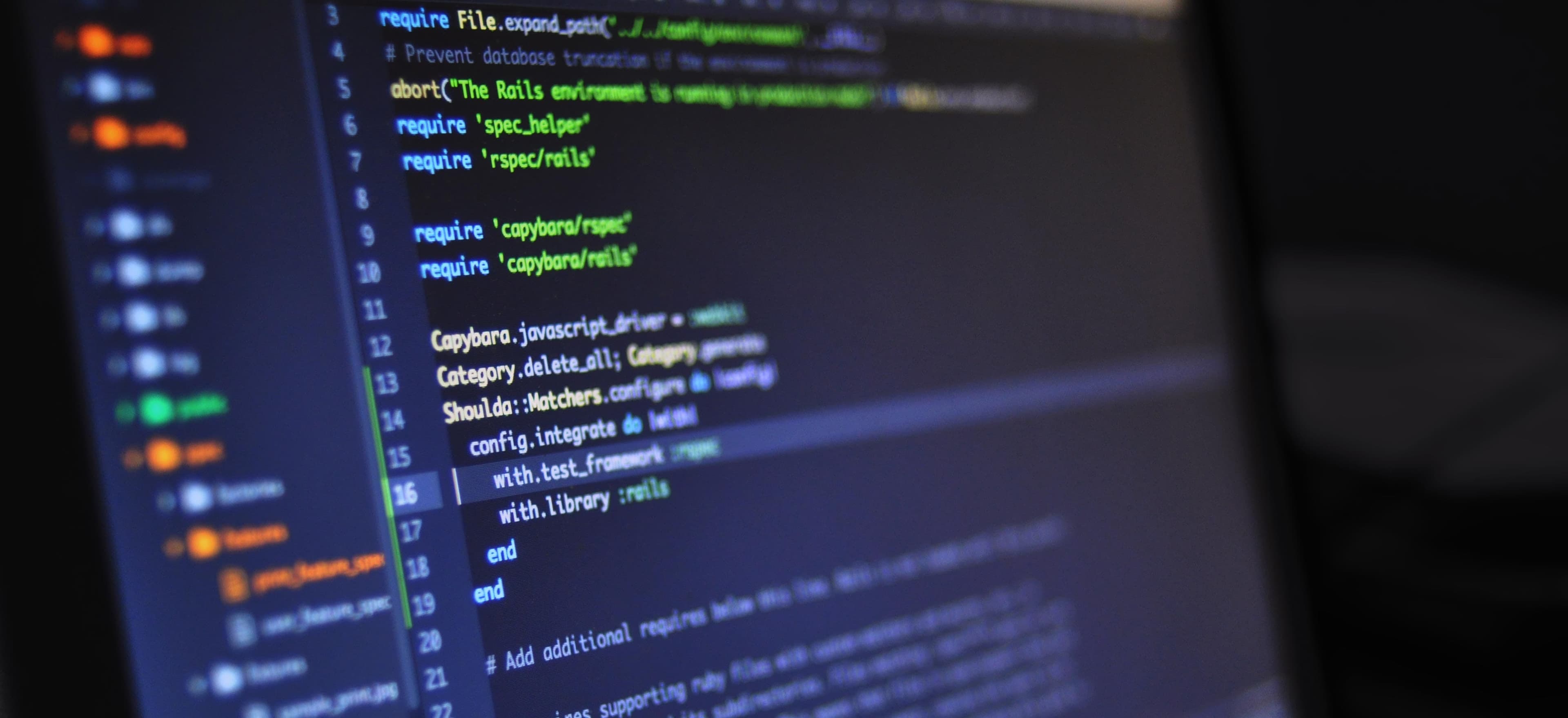
- Published on
Solving Fibonacci in Java: Functional vs Imperative Showdown
Java has long stood as a bastion of reliability in the programming world. With its object-oriented roots gripping tightly into systems large and small, it's no wonder Java continues to be a go-to language for developers across the globe. Within this diverse language, programming paradigms such as imperative and functional styles offer varied approaches to tackling problems. Today, we pit these two styles against each other in a classic computational showdown: the calculation of Fibonacci sequences.
Understanding the Fibonacci Sequence
Before we dive into code, let’s ensure we’re all on the same page. The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding ones, usually starting with 0 and 1. That is, the sequence starts 0, 1, 1, 2, 3, 5, 8, 13, and so forth.
This sequence, though simple, plays a significant role in both mathematics and computer science, serving as a prime example for algorithm explanation, efficiency discussions, and even in practical applications like computer graphics and stock market analysis.
The Imperative Approach
In Java, and programming in general, the imperative style is what most of us learn first. It involves giving the computer a sequence of statements to execute in order, often using loops and variables to keep track of state.
Here’s how you can calculate a Fibonacci number imperatively in Java:
public class Fibonacci {
public static int fibonacciImperative(int n) {
if (n <= 1) {
return n;
}
int first = 0, second = 1, fib = 1;
for (int i = 2; i <= n; i++) {
fib = first + second;
first = second;
second = fib;
}
return fib;
}
public static void main(String[] args) {
System.out.println(fibonacciImperative(9)); // Output: 34
}
}
This piece of code is straightforward and efficient for computing lower Fibonacci numbers. However, as n
gets larger, the time to compute increases dramatically, indicating a potential area for optimization.
The Functional Approach
Java’s venture into the functional programming paradigm, bolstered by the introduction of lambdas and streams in Java 8, offers a fresh perspective on problem-solving. Functional programming emphasizes immutability and higher-order functions.
Let’s look at a functional way to solve the Fibonacci problem:
import java.util.stream.Stream;
public class Fibonacci {
public static long fibonacciFunctional(int n) {
return Stream.iterate(new long[]{0, 1}, f -> new long[]{f[1], f[0] + f[1]})
.limit(n + 1)
.mapToLong(f -> f[0])
.max()
.getAsLong();
}
public static void main(String[] args) {
System.out.println(fibonacciFunctional(9)); // Output: 34
}
}
This code uses Stream.iterate
to generate Fibonacci numbers seamlessly. It emphasizes immutability – there's no changing of state as in the imperative version. This style avails a new way of thinking about the problem by focusing on what to compute rather than how to compute it.
Functional vs. Imperative: A Comparison
Both methods have their place, but how do they stack up against each other?
Readability
For programmers deeply engrained in the imperative style, the imperative solution might seem more readable. However, those accustomed to functional programming will find the functional approach more intuitive due to its declarative nature.
Performance
The imperative approach can be more performant for calculating a single Fibonacci number, especially for lower values of n
. The functional solution shines when dealing with sequences or when integrating into larger functional pipelines, although it might suffer from performance issues due to overheads associated with creating and managing streams.
Scalability
The functional approach is inherently more scalable due to its stateless nature, making it easier to parallelize operations across multiple cores or even distributed systems. Java’s Stream API includes features for parallel processing that can be leveraged for performance gains in the appropriate context.
Maintainability
Functional code, by virtue of its immutability and side-effect-free functions, tends to be more maintainable in the long run. It reduces the cognitive load by minimizing the need to track changing state, thus decreasing the likelihood of bugs related to state management.
When to Use What
Choosing between functional and imperative programming in Java often boils down to the specific problem at hand, the performance characteristics of your application, and your team’s familiarity with Java’s functional features. For tasks involving complex transformations or operations on collections, the functional approach can result in more concise and expressive code. On the other hand, for low-level, performance-critical code, the imperative style might be more appropriate.
Further Reading
To deepen your understanding of functional programming in Java and how it compares to the imperative style, the following resources are invaluable:
Both resources provide a wealth of information that can help refine your approach to Java programming, whether you're leaning towards the functional or staying with the imperative.
Lessons Learned
The Fibonacci sequence, while a simple concept, serves as a perfect battleground for the imperative and functional programming paradigms within Java. Each approach offers unique benefits and drawbacks, with the best choice often depending on the specific context of use. Embracing both styles can only serve to make you a more versatile Java developer, capable of leveraging the full power of this versatile language.
Understanding when to employ each paradigm is as crucial as understanding the paradigms themselves. As Java continues to evolve, keeping abreast of these developments ensures your skills remain sharp and relevant in the ever-changing landscape of software development.
As you continue to explore Java and its capabilities, remember the importance of choosing the right tool for the job. Whether you're counting Fibonacci numbers or building the next breakthrough application, Java's diverse array of programming styles has got you covered.
Happy coding!
Checkout our other articles