Java 17 LTS: Solving Modern Development Challenges
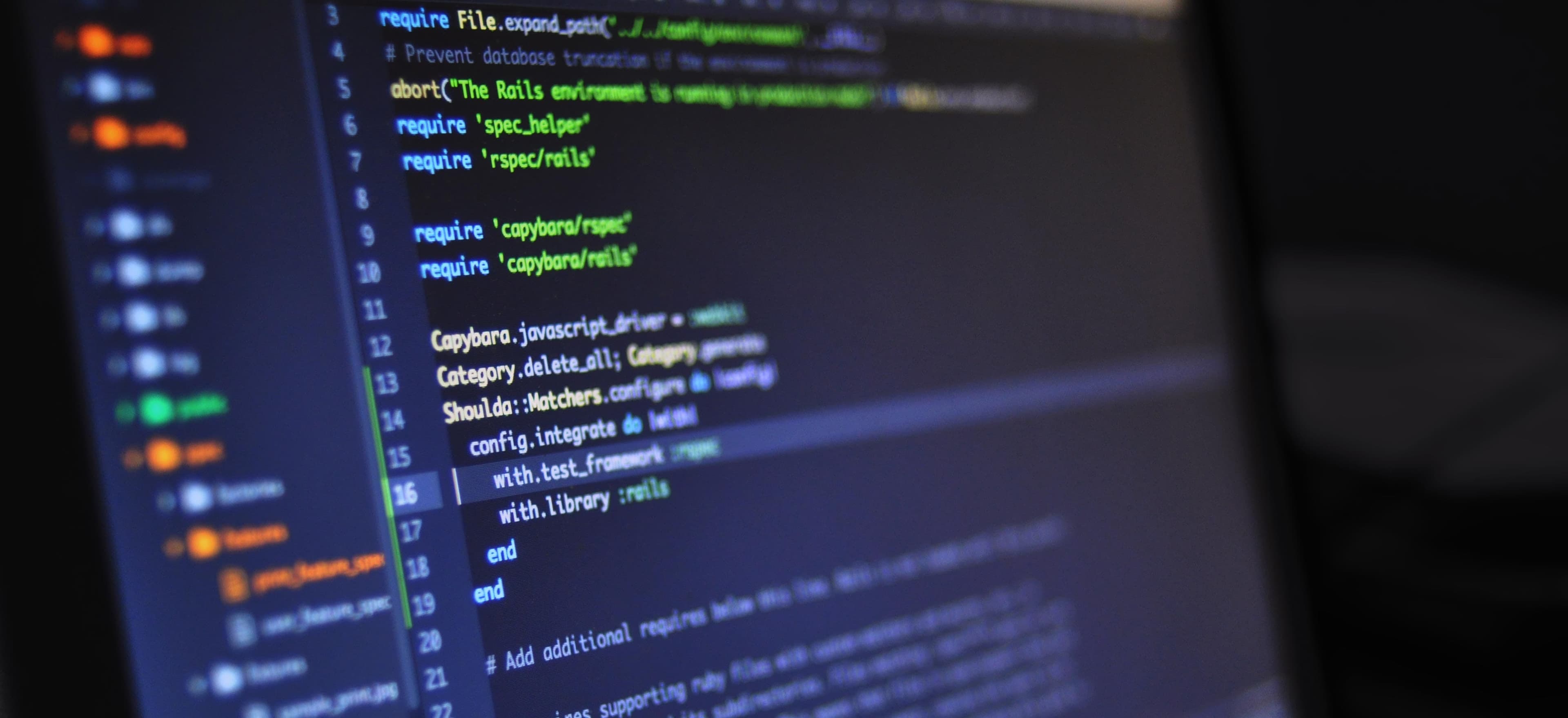
- Published on
Java 17 LTS: Solving Modern Development Challenges
Java, one of the most popular programming languages, has continuously evolved to meet the demands of modern software development. Java 17, released as a Long-Term Support (LTS) version, brings a host of features and improvements that directly address contemporary development challenges. In this article, we'll explore how Java 17 tackles these challenges and empowers developers to write efficient, maintainable, and secure code.
Seamless Migration to Java 17
One of the primary concerns for developers is the seamless migration to a new Java release. Java 17 continues the tradition of maintaining backwards compatibility, ensuring a smooth transition for existing codebases. Additionally, tools like jdeps can assist in identifying any potential hurdles during migration.
// Example of how Java maintains backwards compatibility
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Enhanced Performance with Sealed Classes
Java 17 introduces sealed classes, a feature that allows developers to precisely control which classes can implement a given interface or extend a specific class. This fine-grained control fosters better performance by enabling the compiler to make more efficient decisions at runtime.
// Example of a sealed class
public sealed interface Shape permits Circle, Rectangle {
double area();
}
final class Circle implements Shape {
// Implementation of area for a circle
}
final class Rectangle implements Shape {
// Implementation of area for a rectangle
}
Pattern Matching for Switch
Another significant improvement in Java 17 is the enhancement of the switch statement with pattern matching. This feature simplifies code and enhances readability by allowing developers to conditionally extract components from objects.
// Example of pattern matching for switch
public double calculateArea(Shape shape) {
return switch (shape) {
case Circle c -> Math.PI * c.getRadius() * c.getRadius();
case Rectangle r -> r.getWidth() * r.getHeight();
};
}
Enhanced Security with Edwards-Curve Digital Signature Algorithm (EdDSA)
Security is a paramount concern in modern software development. Java 17 addresses this by adding support for the Edwards-Curve Digital Signature Algorithm (EdDSA). EdDSA is known for its robustness and efficiency, making it a valuable addition to Java's security capabilities.
// Example of using EdDSA for digital signatures
import java.security.*;
import java.security.spec.*;
public byte[] signData(byte[] data, PrivateKey privateKey) throws NoSuchAlgorithmException, InvalidKeyException, SignatureException {
Signature signature = Signature.getInstance("EdDSA");
signature.initSign(privateKey);
signature.update(data);
return signature.sign();
}
Enhanced Productivity with Foreign Function and Memory API (Incubator)
Java 17 introduces the Foreign Function and Memory API as an incubating feature. This API allows Java programs to call native code and interact with native memory in a more efficient and safe manner. This feature is particularly beneficial for developers working on performance-critical applications.
// Example of using the Foreign Function and Memory API to interact with native code
import jdk.incubator.foreign.*;
public static void main(String[] args) {
try (MemorySegment segment =
MemorySegment.allocateNative(1024)) {
// Interact with native memory through the segment
// ...
}
}
Lessons Learned
Java 17 LTS stands as a robust solution for addressing modern development challenges. Its seamless migration, enhanced performance with sealed classes, improved readability with pattern matching for switch, enhanced security with EdDSA, and increased productivity with the Foreign Function and Memory API, collectively make Java 17 an indispensable tool for developers.
Embrace Java 17 to unlock the full potential of modern software development while staying ahead in the rapidly-evolving tech landscape. With its arsenal of features and improvements, Java 17 LTS is positioned to be a crucial ally for developers striving to create efficient, secure, and maintainable software.
Checkout our other articles