Solving Spring's Component Scanning Delays: A Deep Dive
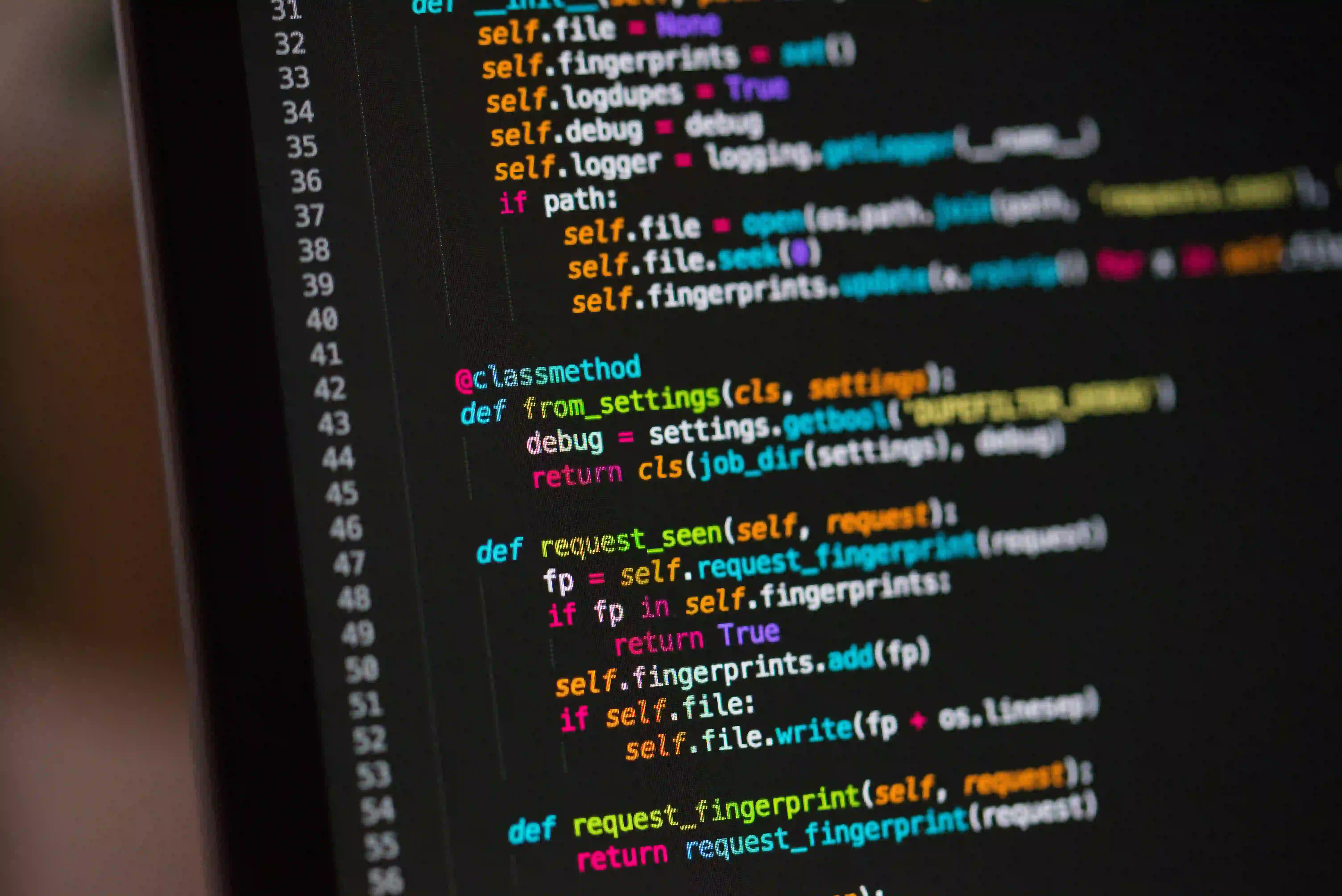
Solving Spring's Component Scanning Delays: A Deep Dive
When dealing with large Spring applications, one of the common pain points is the increasing time it takes for the application to start up due to component scanning delays. In this article, we will explore the causes behind these delays and discuss some effective strategies to mitigate them. We will focus on Java and Spring Framework to address these issues.
Understanding Component Scanning Delays
In a typical Spring application, component scanning is used to automatically detect and register Spring components such as @Component
, @Service
, @Repository
, and @Controller
classes. However, as the codebase grows, the number of classes to be scanned increases, leading to longer startup times.
One of the main reasons for these delays is the classpath scanning mechanism used by Spring. When the application starts, Spring scans the entire classpath to find and register components. As the number of classes and third-party libraries in the classpath grows, the scanning process becomes slower, ultimately affecting the application's startup time.
Strategies to Mitigate Component Scanning Delays
1. Narrow Down Component Scanning
Instead of scanning the entire application, it's often more efficient to narrow down the component scanning to specific packages or directories where the application's components are located. This can be achieved by specifying the base packages for component scanning using the @ComponentScan
annotation.
@Configuration
@ComponentScan("com.example.myapp.components")
public class AppConfig {
// Other configuration
}
By explicitly defining the packages to be scanned, we can reduce unnecessary scanning of unrelated classes, thereby improving startup time.
2. Lazy Initialization of Beans
In scenarios where certain beans are not required during application startup, we can leverage lazy initialization to defer bean creation until they are actually needed.
@Component
@Lazy
public class ExpensiveBean {
// Bean definition
}
By marking specific beans with @Lazy
, we can defer their instantiation, thereby reducing the initial startup time.
3. Utilize Classpath Scanning Exclusion Filters
Spring provides the ability to define custom filters to exclude specific classes from component scanning. This can be particularly useful when dealing with third-party libraries or frameworks where the scanning of certain classes is unnecessary.
@Configuration
@ComponentScan(basePackages = "com.example.myapp.components",
excludeFilters = @ComponentScan.Filter(type = FilterType.REGEX, pattern = "com.example.myapp.components.exclude.*"))
public class AppConfig {
// Other configuration
}
By utilizing exclusion filters, we can explicitly exclude certain classes or packages from the component scanning process, thereby improving scanning performance.
4. Explicitly Define Beans
In cases where component scanning delays are a concern, an alternative approach is to explicitly define beans using @Bean
methods within @Configuration
classes.
@Configuration
public class AppConfig {
@Bean
public MyBean myBean() {
return new MyBean();
}
// Other bean definitions
}
By defining beans explicitly, we bypass the component scanning process for those beans, leading to faster startup times.
5. Utilize Compile-Time Annotation Processors
Using compile-time annotation processors such as Spring annotation processor can help generate metadata about annotated components at compile time. This metadata can then be used to avoid expensive classpath scanning during application startup.
Final Thoughts
In large Spring applications, component scanning delays can significantly impact startup times. By understanding the underlying causes and employing effective strategies, we can mitigate these delays and ensure optimal application startup performance.
From narrowing down component scanning and utilizing lazy initialization of beans to leveraging exclusion filters and explicit bean definitions, various approaches can be tailored to address specific performance bottlenecks.
As developers, it's crucial to continuously evaluate and optimize the startup performance of Spring applications, ensuring a streamlined and responsive user experience.
By adopting the strategies discussed in this article, developers can proactively address component scanning delays, leading to improved overall performance and user satisfaction.
Remember, optimizing application performance is a continuous journey, and addressing component scanning delays is an essential part of that journey.