Generating Better Java Code in IntelliJ with Google Guava
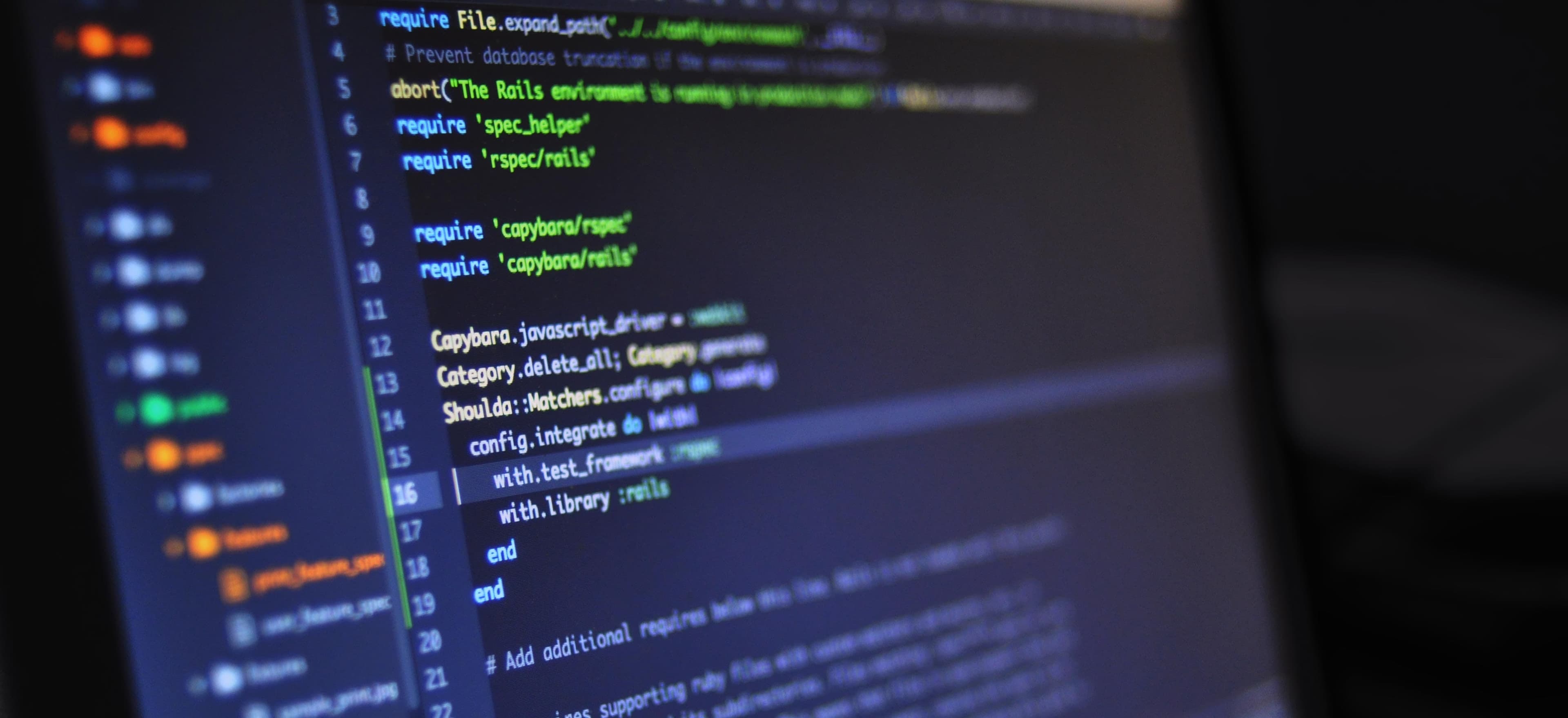
- Published on
Generating Better Java Code in IntelliJ with Google Guava
When it comes to writing efficient and clean Java code, using the right tools and libraries can make a world of difference. In this article, we'll explore how Google Guava, a set of core libraries for Java, can be integrated into IntelliJ IDEA to streamline and enhance Java development.
What is Google Guava?
Google Guava is an open-source set of core libraries for Java, developed by Google. It provides utility methods and classes for a wide range of tasks such as collections, caching, functional programming, and much more. Guava's goal is to make Java development simpler and more robust by offering a rich set of features that complement the standard Java libraries.
Setting Up Google Guava in IntelliJ IDEA
Before diving into the specifics of using Google Guava in IntelliJ, it's important to ensure that the Guava library is added to the project. This can be accomplished by adding the Guava dependency to the pom.xml
file for Maven projects or the build.gradle
file for Gradle projects.
For Maven:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>30.1-jre</version>
</dependency>
For Gradle:
implementation 'com.google.guava:guava:30.1-jre'
With the dependency added, IntelliJ IDEA will automatically download and synchronize the Guava library into the project.
Using Google Guava in IntelliJ IDEA
Once Guava is set up within the project, IntelliJ IDEA provides a seamless experience for leveraging its functionality. Let's explore some of the ways in which Google Guava can be used to write better Java code.
Using Preconditions
In Java, it's common to check method preconditions using explicit if
conditions. Google Guava simplifies this process by providing the Preconditions
class, which offers static methods for checking preconditions and throwing exceptions if the check fails.
For example:
public void processOrder(Order order) {
Preconditions.checkNotNull(order, "Order cannot be null");
// Process the order
}
In this example, the checkNotNull
method ensures that the order
object is not null, throwing an IllegalArgumentException
with the specified error message if it is.
Immutable Collections
Google Guava provides a set of immutable collections, making it easier to ensure that a collection cannot be modified once it's created. This can help prevent unintended changes and improve the predictability of the code.
List<String> immutableList = ImmutableList.of("one", "two", "three");
In this case, immutableList
is an immutable list that cannot be modified after creation, adding a layer of safety to the code.
Functional Programming Support
Google Guava includes utilities to support functional programming in Java, such as the Function
and Predicate
interfaces. These interfaces can be used to define and compose functions and predicates in a more concise and expressive manner.
Function<String, Integer> lengthFunction = input -> input.length();
List<String> words = ImmutableList.of("apple", "banana", "orange");
List<Integer> wordLengths = Lists.transform(words, lengthFunction);
In this example, the transform
method applies the lengthFunction
to each element of the words
list, generating a list of word lengths.
More Powerful Utilities
Beyond the examples mentioned above, Google Guava offers a plethora of additional utilities, including:
-
Caching: The Guava
Cache
provides a powerful and thread-safe way to cache values, with support for automatic eviction policies and asynchronous loading. -
String Manipulation: Guava's
CharMatcher
andCaseFormat
classes offer convenient methods for string manipulation and formatting. -
File Utilities: Guava includes utilities for working with files, such as
Files
for reading and writing files, andResources
for managing classpath resources.
By leveraging these utilities, Java developers can write more concise, readable, and efficient code without reinventing the wheel.
Wrapping Up
Integrating Google Guava into IntelliJ IDEA can significantly improve the process of writing Java code. By taking advantage of Guava's rich set of utilities, developers can streamline their code, enhance its readability, and reduce the likelihood of common programming errors.
Incorporating best practices and leveraging powerful libraries like Google Guava is essential for Java developers striving to produce high-quality, maintainable code.
So, why wait? Boost your Java development efficiency by harnessing the power of Google Guava in IntelliJ IDEA today!
For further reference, you can check out the official Google Guava Documentation for detailed information about the library's features and usage.
Checkout our other articles