Bytes in Java: Mastering File-to-Array Conversion
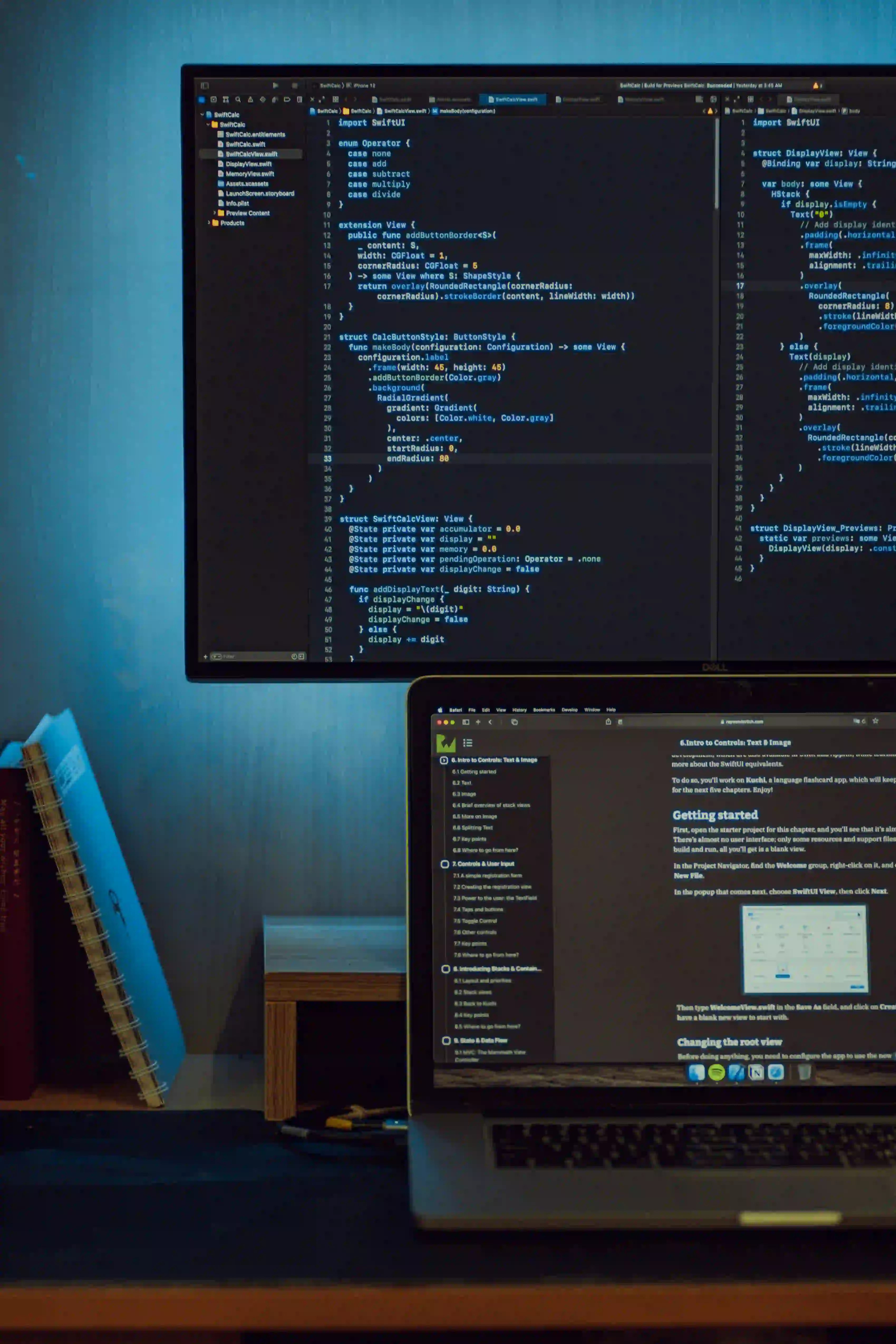
Mastering File-to-Array Conversion in Java
Working with files and converting their contents to arrays is a fundamental operation in Java programming. Whether you are reading a text file, an image file, or any other type of file, it's crucial to understand how to efficiently handle file-to-array conversions. In this blog post, we will delve into the best practices for mastering file-to-array conversion in Java, covering various techniques, potential challenges, and how to overcome them.
Understanding the Basics
Before we dive into the code, let's take a moment to understand the basic concepts involved in file-to-array conversion.
Input and Output Streams
In Java, file operations are often performed using input and output streams. An input stream is used to read data from a source, while an output stream is used to write data to a destination. When working with file-to-array conversion, we primarily use input streams to read the contents of a file into an array.
Byte Arrays
A byte array is a fundamental data structure in Java used to store a sequence of bytes. When dealing with file content, it is common to read the bytes from a file into a byte array for further processing.
Reading File Content into a Byte Array
Now, let's explore how to read the content of a file into a byte array in Java. We'll discuss two common approaches to achieve this: using plain Java IO and leveraging the NIO (New I/O) package, which provides more advanced features for file operations.
Using Java IO
import java.io.ByteArrayOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
public class FileToArrayConverter {
public byte[] convertFileToByteArray(String filePath) throws IOException {
File file = new File(filePath);
try (FileInputStream fis = new FileInputStream(file);
ByteArrayOutputStream bos = new ByteArrayOutputStream()) {
byte[] buffer = new byte[1024];
int length;
while ((length = fis.read(buffer)) != -1) {
bos.write(buffer, 0, length);
}
return bos.toByteArray();
}
}
}
In the code snippet above, we create a FileToArrayConverter
class with a method convertFileToByteArray
that takes the file path as input and returns its content as a byte array. Inside the method, we use a FileInputStream
to read the file's content into a byte array using a buffer to optimize the process. The ByteArrayOutputStream
is then used to collect the bytes read from the file.
Using NIO (New I/O)
Java NIO introduces the java.nio.file.Files
class, providing a more modern and efficient way to read file contents into a byte array.
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
public class FileToArrayNIOConverter {
public byte[] convertFileToByteArrayNIO(String filePath) throws IOException {
Path path = Paths.get(filePath);
return Files.readAllBytes(path);
}
}
In the NIO-based approach, the FileToArrayNIOConverter
class contains a method convertFileToByteArrayNIO
that utilizes the Files.readAllBytes
method to directly read the contents of the file into a byte array. This method provides a more concise and modern way of achieving the same result.
Which Approach to Choose?
Both approaches are valid, but when working with large files, the NIO approach is generally more efficient due to its underlying mechanisms for asynchronous, non-blocking I/O operations and improved buffer management.
Dealing with Potential Challenges
During file-to-array conversion, several challenges may arise, such as handling large files, managing memory efficiently, and ensuring proper error handling. Let's discuss some strategies to address these challenges.
Handling Large Files
When dealing with large files, it's crucial to consider memory limitations. Reading an entire large file into memory at once can lead to OutOfMemoryError. To handle large files more efficiently, consider using techniques such as memory mapping with NIO or processing the file in smaller chunks.
Managing Memory Efficiently
In both the Java IO and NIO approaches discussed earlier, it's important to close the input streams and free up system resources after reading the file content. This can be achieved by using try-with-resources or ensuring explicit stream closure in a finally
block.
// Example of closing input stream using try-with-resources
try (FileInputStream fis = new FileInputStream(file);
ByteArrayOutputStream bos = new ByteArrayOutputStream()) {
// Read file content into byte array
} catch (IOException e) {
// Handle exception
}
Error Handling
When working with file operations, it's critical to anticipate and handle potential IO exceptions that may occur. Proper error handling ensures graceful degradation and robustness in your file-to-array conversion logic.
Key Takeaways
Mastering file-to-array conversion in Java is essential for a wide range of applications, from reading configuration files to parsing binary data. By understanding the basic concepts, choosing the appropriate approach for reading file content into a byte array, and addressing potential challenges, you can effectively utilize file-to-array conversion in your Java projects.
In this blog post, we covered the fundamentals of file-to-array conversion, explored practical code examples using both Java IO and NIO, and discussed strategies for dealing with challenges associated with this process. Armed with this knowledge, you are now well-equipped to manage file-to-array conversion with confidence in your Java applications.
Happy coding!
Java Documentation on File IO Java NIO Guide
Remember, mastering file-to-array conversion is not just about the 'how,' it's also about the 'why.' Understanding the intricacies behind different file-to-array conversion techniques and knowing when to apply them ensures efficient and robust file handling in your Java applications.