Streamline Your Day: 14 Keys to Mastering Workflow Automation
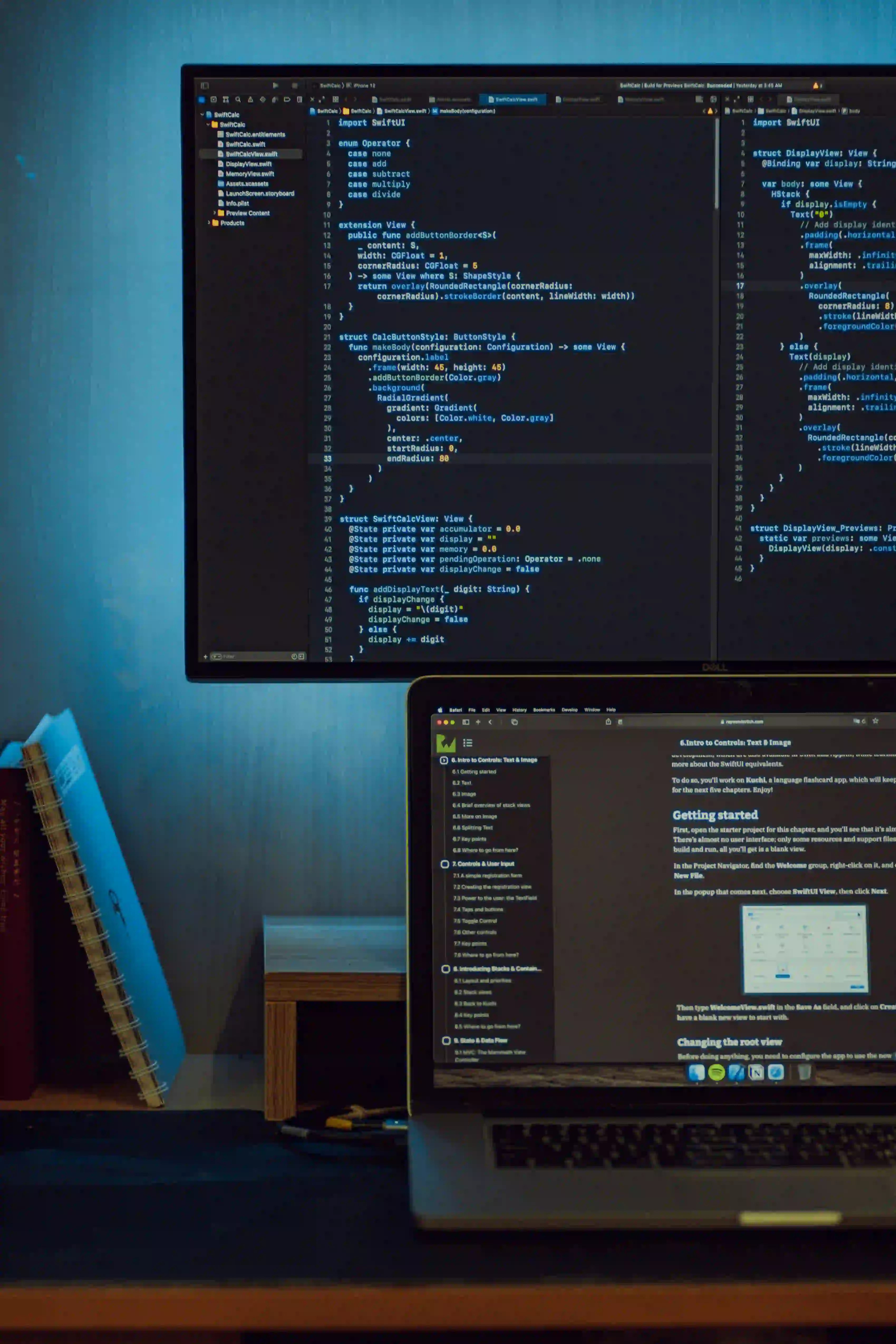
Mastering Workflow Automation in Java
In today's fast-paced world, mastering workflow automation is essential for staying ahead of the curve. With Java, an incredibly powerful and versatile programming language, you can streamline your daily tasks and enhance your productivity. In this blog post, we'll explore 14 key strategies for mastering workflow automation in Java.
1. Understand the Basics
Before diving into workflow automation, it's crucial to have a solid understanding of Java fundamentals. Familiarize yourself with key concepts such as classes, objects, methods, and data types. This knowledge forms the foundation for building efficient automation workflows in Java.
2. Leverage Java's Libraries
Java boasts a rich ecosystem of libraries and frameworks that can significantly simplify workflow automation. Leveraging libraries like Apache Commons IO for file operations, Log4j for logging, and Gson for JSON processing can expedite the development process and enhance the robustness of your automation workflows.
3. Embrace Lambda Expressions
Lambda expressions, introduced in Java 8, are a powerful feature for concise and functional programming. By embracing lambda expressions, you can write more expressive and compact code, particularly when working with collections and streams. For example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
numbers.stream().map(n -> n * 2).forEach(System.out::println);
4. Implement Multithreading
Efficient workflow automation often involves handling multiple tasks concurrently. Java's support for multithreading and concurrency makes it an ideal choice for building automation workflows that can execute tasks in parallel, thereby improving performance and responsiveness.
5. Use Design Patterns
Applying design patterns such as the Factory Method, Singleton, and Builder patterns in your workflow automation solutions can enhance maintainability, extensibility, and readability of your codebase. These proven best practices help in structuring your automation workflows and promoting code reusability.
6. Employ Exception Handling
Robust workflow automation necessitates robust error handling. In Java, proper exception handling using try-catch blocks ensures that your automation workflows gracefully handle unexpected scenarios, thus enhancing reliability and fault tolerance.
try {
// Code that may throw an exception
} catch (IOException e) {
// Handle the exception
logger.error("An IO exception occurred", e);
}
7. Harness the Power of APIs
Integrating with external systems, services, and data sources is often a key aspect of workflow automation. Java provides robust support for working with APIs through libraries like Retrofit for RESTful APIs, JDBC for database interaction, and JMS for messaging. This enables seamless integration of external functionalities into your automation workflows.
8. Utilize Reflection
Reflection empowers you to inspect and manipulate classes, interfaces, fields, and methods dynamically at runtime. Leveraging reflection in your automation workflows can enable dynamic configuration, customization, and extensibility, adding a layer of adaptability to your solutions.
9. Choose the Right Data Structures
Selecting the appropriate data structures such as lists, maps, sets, queues, and stacks is pivotal in designing efficient automation workflows. Each data structure has its strengths and use cases, and choosing the right one can optimize memory usage and improve the performance of your automation solutions.
10. Optimize IO Operations
Efficient handling of input/output (IO) operations is crucial for streamlined automation workflows. Leveraging NIO (New I/O) and asynchronous IO can significantly improve the efficiency of file and network operations, reducing latency and increasing throughput in your workflows.
11. Embrace Testing Best Practices
Testing is an integral part of building robust automation workflows. Embrace testing best practices such as unit testing with JUnit, integration testing, and mocking frameworks like Mockito to ensure the reliability and correctness of your automation solutions.
12. Implement Logging and Monitoring
Incorporating comprehensive logging using frameworks like Logback or Log4j, coupled with monitoring tools like Prometheus or ELK stack, allows you to gain insights into the behavior of your automation workflows. Proactive logging and monitoring facilitate debugging, performance optimization, and proactive issue identification.
13. Design for Scalability
As your automation workflows grow in complexity and scope, designing for scalability becomes imperative. Employ techniques such as load balancing, caching, asynchronous processing, and distributed computing to ensure that your workflows can handle increasing workloads without sacrificing performance or stability.
14. Stay Abreast of Java Updates
Java continuously evolves, with new features, enhancements, and performance improvements introduced in each release. Keeping abreast of the latest Java updates ensures that you leverage the full potential of the language, its libraries, and the JVM for optimizing your workflow automation solutions.
In conclusion, mastering workflow automation in Java involves a holistic approach encompassing fundamental understanding, leveraging language features and libraries, employing best practices, and staying current with the evolving Java ecosystem. By implementing the 14 keys discussed in this post, you can elevate your workflow automation capabilities and drive efficiency in your development processes.
Start incorporating these strategies into your Java workflow automation endeavors, and witness a transformation in your productivity and the robustness of your solutions.
Remember, the key to success lies not just in automation, but in mastering the art of automation, and Java equips you with the tools to do just that.
Happy automating!