Top Books That Skyrocketed My Coding Skills & Career
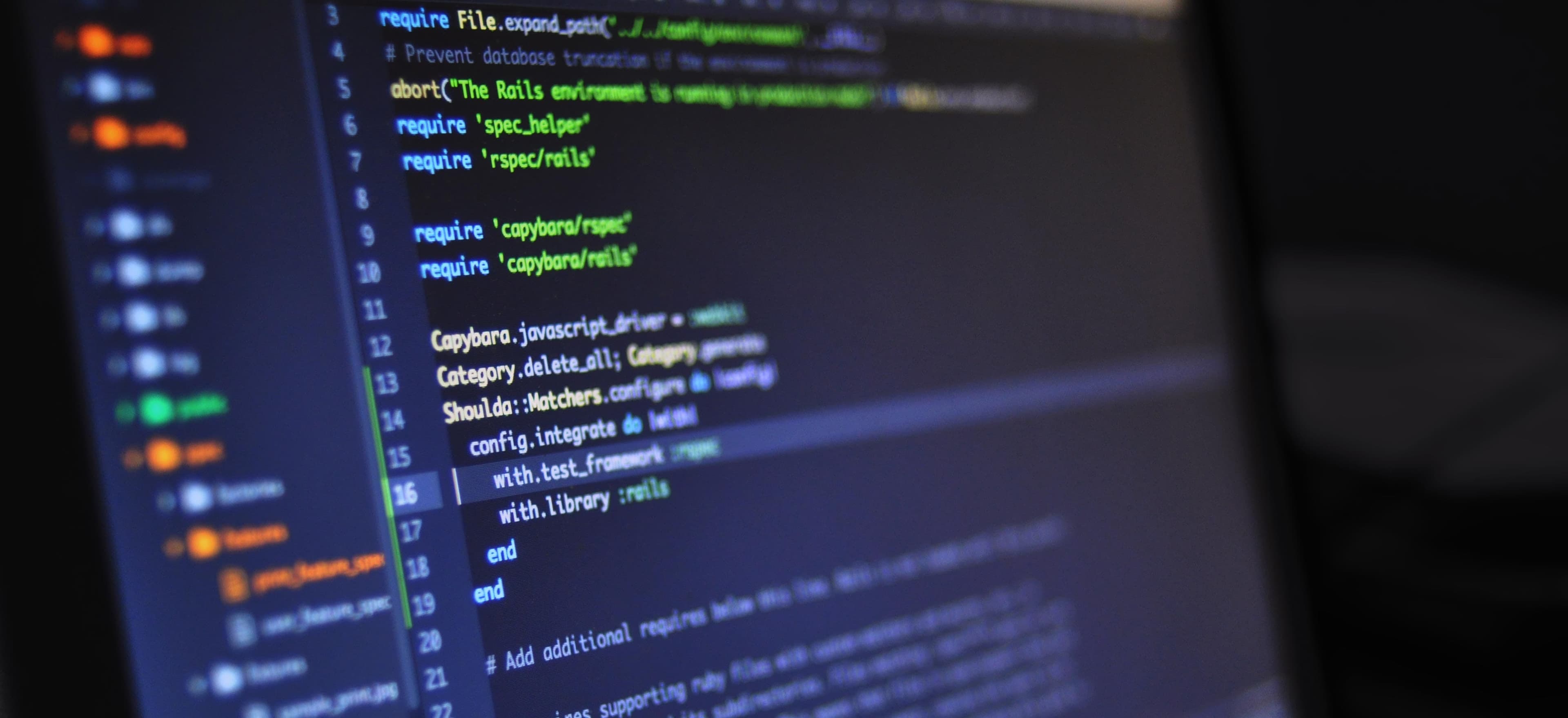
- Published on
Top Books That Skyrocketed My Coding Skills & Career
As a software developer, I've come to realize that improving my coding skills is an ongoing journey. Over the years, I've read numerous books that have significantly enhanced my understanding of Java and propelled my career to new heights. In this blog post, I'll share some of the top books that have had a profound impact on my coding proficiency and career progression.
Effective Java by Joshua Bloch
!Effective Java
Effective Java by Joshua Bloch is an essential read for any Java developer looking to elevate their coding skills. This book provides 78 best practices for writing high-quality Java code, covering a wide range of topics such as object creation, classes and interfaces, lambda expressions, and concurrency. Each item in the book offers clear explanations of the "what" and "why" behind the recommended practices, empowering developers to write robust and maintainable code.
Code Snippet from Effective Java
// Item 1: Consider static factory methods instead of constructors
public static Complex valueOf(double re, double im) {
return new Complex(re, im);
}
Why It Matters: Using static factory methods provides flexibility in the creation of objects and allows for improved code readability.
Clean Code by Robert C. Martin
!Clean Code
Clean Code by Robert C. Martin is a masterpiece that delves into the principles and practices of writing clean, maintainable, and elegant code. This book offers practical advice on how to refactor existing code, choose meaningful names, and craft functions that are clear and concise. By following the principles outlined in the book, developers can significantly enhance the readability and extensibility of their Java codebase.
Code Snippet from Clean Code
public boolean isPrime(int number) {
if (number <= 1) return false;
for (int i = 2; i <= Math.sqrt(number); i++) {
if (number % i == 0) return false;
}
return true;
}
Why It Matters: Writing clean and understandable code enhances maintainability and reduces the likelihood of introducing bugs.
Java Concurrency in Practice by Brian Goetz et al.
!Java Concurrency in Practice
Java Concurrency in Practice by Brian Goetz et al. is a comprehensive guide to writing concurrent programs in Java. The book covers fundamental concepts such as thread safety, synchronization, and execution control, providing practical strategies for designing concurrent and scalable Java applications. By mastering the concepts presented in this book, developers can effectively tackle the challenges associated with concurrency and build robust, responsive, and efficient multithreaded applications.
Code Snippet from Java Concurrency in Practice
public class ConcurrentTaskExecutor {
private final Executor executor = Executors.newFixedThreadPool(5);
public void executeTask(Runnable task) {
executor.execute(task);
}
}
Why It Matters: Understanding Java concurrency is crucial for developing high-performance and responsive applications, especially in today's multi-core and distributed computing environments.
My Closing Thoughts on the Matter
These books have not only honed my coding skills but have also played a pivotal role in shaping my career as a Java developer. By internalizing the insights and best practices shared in these books, I've been able to write cleaner, more maintainable code, effectively leverage concurrency for performance gains, and ultimately stand out as a proficient and valuable asset in the software development industry.
Reading these books has been a transformative experience, and I wholeheartedly recommend them to every Java developer who aspires to reach new heights in their coding journey.
Start exploring these books, and let them propel your coding skills and career to the next level! Give them a read and watch your Java knowledge and career prospects soar!
Checkout our other articles