Overcoming Thread Starvation in Spring Boot WebFlux Apps
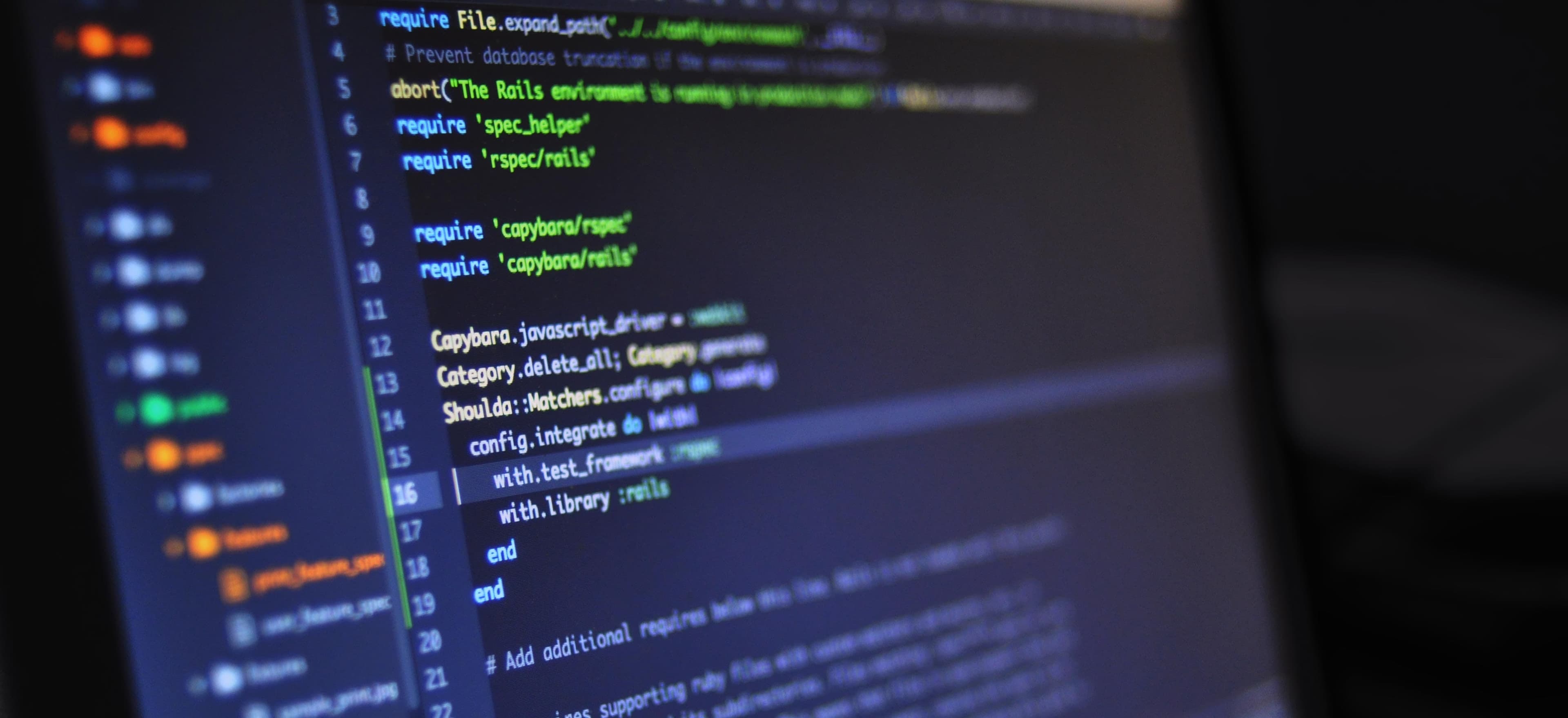
- Published on
Overcoming Thread Starvation in Spring Boot WebFlux Apps
When it comes to developing high-performance, non-blocking applications in Java, Spring Boot WebFlux is a popular choice. WebFlux provides a reactive programming model that allows for handling a large number of concurrent connections with a small number of threads. However, as with any asynchronous programming model, developers need to be aware of potential issues such as thread starvation.
Thread starvation occurs when all available threads in the application are blocked, waiting for some resource to become available. In the context of Spring Boot WebFlux applications, this can happen if the reactive streams are not being handled properly, causing the threads to be blocked and unavailable to handle incoming requests.
In this article, we'll explore the concept of thread starvation in the context of Spring Boot WebFlux applications, and we'll discuss strategies to overcome this issue to ensure optimal performance of your reactive applications.
Understanding Thread Starvation in WebFlux
In a typical Spring Boot WebFlux application, incoming requests are handled by a limited number of worker threads, which are used to process the reactive streams. If these worker threads are blocked for a prolonged period, it can lead to thread starvation, ultimately impacting the responsiveness and throughput of the application.
One common scenario that can lead to thread starvation is when a reactive stream performs a blocking operation, such as calling a synchronous external service or performing a time-consuming computation. If this blocking operation is not handled properly, it can cause the worker threads to be tied up, waiting for the operation to complete, thereby preventing them from processing other incoming requests.
Overcoming Thread Starvation
1. Avoiding Blocking Operations
One of the fundamental principles of building reactive applications is to avoid blocking operations. In the context of Spring Boot WebFlux, this means ensuring that your reactive streams don't perform any synchronous, blocking operations that can tie up the worker threads. Instead, use non-blocking operations or delegate the blocking operations to a separate thread pool to prevent thread starvation.
Flux<User> getUsers() {
return userRepository.findAll()
.subscribeOn(Schedulers.boundedElastic());
}
In this example, the userRepository.findAll()
method performs a blocking database operation. By using subscribeOn(Schedulers.boundedElastic())
, the operation is offloaded to a separate thread pool, preventing the worker threads from being blocked.
2. Increasing Thread Pool Size
Another approach to overcome thread starvation is to increase the size of the worker thread pool. By configuring a larger thread pool, you can accommodate a higher number of concurrent requests without experiencing thread starvation. However, increasing the thread pool size should be done judiciously, considering the available resources and the nature of the workload.
@Configuration
public class WebFluxConfig implements WebFluxConfigurer {
@Override
public void configureHttpMessageCodecs(ServerCodecConfigurer configurer) {
configurer.defaultCodecs().maxInMemorySize(16 * 1024 * 1024);
}
@Bean
public HandlerMapping handlerMapping() {
ThreadPoolTaskExecutor executor = new ThreadPoolTaskExecutor();
executor.setCorePoolSize(10);
executor.setMaxPoolSize(10);
executor.setThreadNamePrefix("webflux-thread-");
executor.initialize();
Map<String, WebSocketHandler> map = new HashMap<>();
map.put("/websocket", new MyWebSocketHandler());
SimpleUrlHandlerMapping mapping = new SimpleUrlHandlerMapping();
mapping.setUrlMap(map);
mapping.setOrder(10);
return mapping;
}
}
In this configuration, the ThreadPoolTaskExecutor
is used to define a custom thread pool with a core pool size and max pool size of 10. This ensures that a larger number of worker threads are available to handle incoming requests, thus reducing the likelihood of thread starvation.
3. Implementing Circuit Breakers
Circuit breakers are a pattern for handling faults in distributed systems. By implementing circuit breakers in your WebFlux application, you can prevent cascading failures and mitigate the impact of any thread starvation caused by faulty or slow external services.
Spring Cloud provides a powerful circuit breaker implementation called Resilience4j, which can be easily integrated with Spring Boot WebFlux applications.
@Bean
public WebClient webClient() {
CircuitBreaker circuitBreaker = CircuitBreaker.ofDefaults("backendService");
return WebClient.builder()
.filter(CircuitBreakerWebFilter.from(circuitBreaker))
.build();
}
In this example, a CircuitBreaker
is created and integrated with a WebClient
instance. The circuit breaker will automatically open when the specified failure threshold is exceeded, preventing the application from making further requests to the faulty service, thus avoiding thread starvation caused by waiting on unresponsive external services.
Key Takeaways
Thread starvation is a critical concern in high-performance, non-blocking applications built using Spring Boot WebFlux. By understanding the causes of thread starvation and implementing strategies to mitigate this issue, you can ensure the optimal performance and responsiveness of your reactive applications.
In this article, we discussed the concept of thread starvation in the context of Spring Boot WebFlux applications, and we explored various approaches to overcome thread starvation, including avoiding blocking operations, increasing thread pool size, and implementing circuit breakers.
By following these best practices and leveraging the capabilities of Spring Boot WebFlux, you can build robust, high-performance reactive applications that effectively handle concurrent requests without succumbing to thread starvation.
Remember, in a world of non-blocking, reactive programming, addressing thread starvation is crucial for maintaining the scalability and responsiveness of your applications.
Reference:
Checkout our other articles