Why Java Streams Miscount: A Deep Dive into Count()
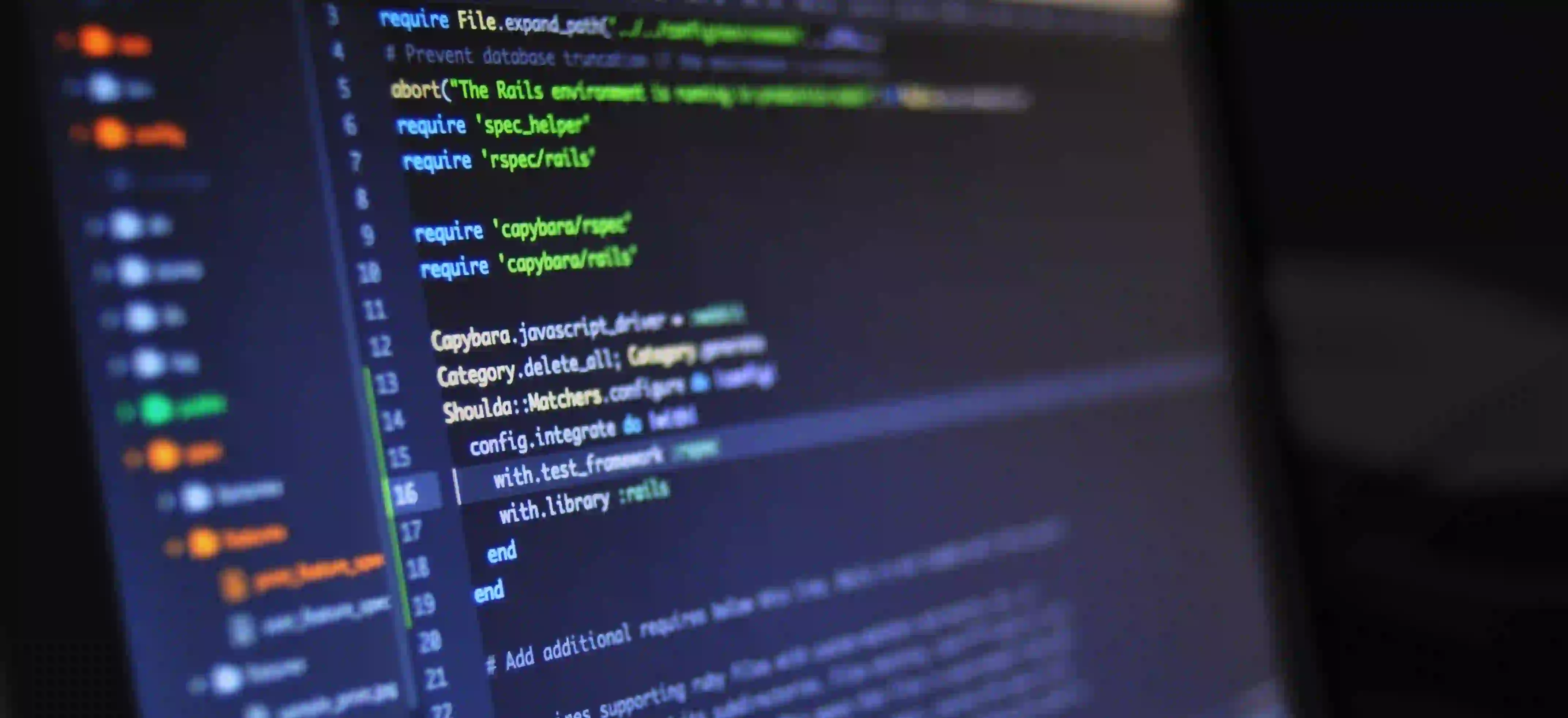
Why Java Streams Miscount: A Deep Dive into Count()
In the world of Java programming, streams have revolutionized the way we handle collections, offering a more functional approach to processing sequences of elements. However, as with any robust system, understanding the intricacies of how Java streams operate, especially when it comes to the count()
method, is crucial for avoiding subtle bugs and performance issues that could creep into your code. In this deep dive, we'll unravel the mysteries behind why Java streams might miscount and how to leverage them correctly.
Understanding Java Streams
Before diving into the nuances of the count()
method, let's briefly revisit the concept of Java streams. Introduced in Java 8, streams represent a sequence of elements supporting sequential and parallel aggregate operations. For more detailed insights into streams, the official Java documentation is an excellent resource.
Streams can be sourced from collections, arrays, or I/O channels and support various operations, which can be classified as intermediate (transform the stream without consuming it, e.g., filter
, map
) or terminal (produce a result or side-effect, e.g., forEach
, reduce
, count
).
The Count() Method: A Closer Look
The count()
method is a terminal operation returning the count of elements in the stream. At first glance, it appears straightforward:
long count = Stream.of(1, 2, 3, 4, 5).count();
System.out.println(count); // Outputs 5
Here, the count()
method accurately tallies the number of elements. So, where do the potential issues with miscounting arise?
Common Pitfalls with Count()
- Parallel Streams and Non-Thread-Safe Operations:
When working with parallel streams, operations that are not thread-safe can lead to unpredictable counts. Consider the case where we're using a non-thread-safe collection within a parallel stream:
List<Integer> list = new ArrayList<>(Arrays.asList(1, 2, 3, 4, 5, 6, 7, 8, 9, 10));
long count = list.parallelStream()
.filter(e -> e % 2 == 0)
.count();
System.out.println(count); // Expected output is 5
While this specific example might not directly cause an incorrect count, augmenting the stream with non-thread-safe operations (like modifying the source collection concurrently) can result in erroneous behavior. Always ensure thread safety when dealing with parallel streams.
- Stateful Lambda Expressions:
Utilizing stateful lambda expressions within stream operations can also lead to miscounts:
Set<Integer> seen = Collections.synchronizedSet(new HashSet<>());
long count = Stream.of(1, 1, 2, 2, 3, 3, 4, 4)
.parallel()
.filter(e -> seen.add(e)) // Stateful lambda expression
.count();
System.out.println(count); // Output might not be 4 due to race conditions
Here, the filter
operation uses a stateful lambda expression, which could lead to race conditions and incorrect counting in a parallel stream context.
Best Practices for Accurate Counts
- Avoid modifying the source during stream operations: Ensure that the source of your stream remains unchanged during the stream's life to prevent unexpected behavior.
- Steer clear of stateful operations in parallel streams: To maintain thread safety, avoid stateful lambda expressions within parallel streams.
- Use appropriate collection types for parallel processing: For parallel streams, consider using thread-safe collections like
ConcurrentHashMap
orCopyOnWriteArrayList
to ensure accurate operations.
Debugging Miscounts: An Example
Let's tackle a practical example. Imagine we're trying to count the unique occurrences of words in a text. Utilizing a parallel stream to improve performance could seem like a logical step. However, if we don't correctly aggregate the results, miscounts can occur.
String[] words = {"hello", "world", "hello", "streams", "world", "parallel"};
Map<String, Integer> wordOccurrences = new ConcurrentHashMap<>();
Arrays.stream(words)
.parallel()
.forEach(word -> wordOccurrences.merge(word, 1, Integer::sum));
long uniqueWords = wordOccurrences.keySet().stream().count();
System.out.println("Unique words: " + uniqueWords);
In this example, by using a ConcurrentHashMap
and the merge
method, we ensure thread-safe updates to the map, leading to an accurate count of unique words.
Conclusion
While Java streams offer a powerful framework for processing data, understanding their subtleties is essential to harness their full potential. Miscounts with the count()
method typically emerge from parallel stream issues or stateful operations, which can be mitigated by following best practices for thread safety and stream usage.
For more complex operations, refer to additional resources and advanced guides like the Oracle Streams documentation to deepen your understanding of stream behaviors and capabilities.
In summary, with careful attention to detail and adherence to best practices, Java streams can be a potent tool in your programming arsenal, allowing for efficient and correct data processing.