Revamping Java UI: Mastering JMetro Combo Box
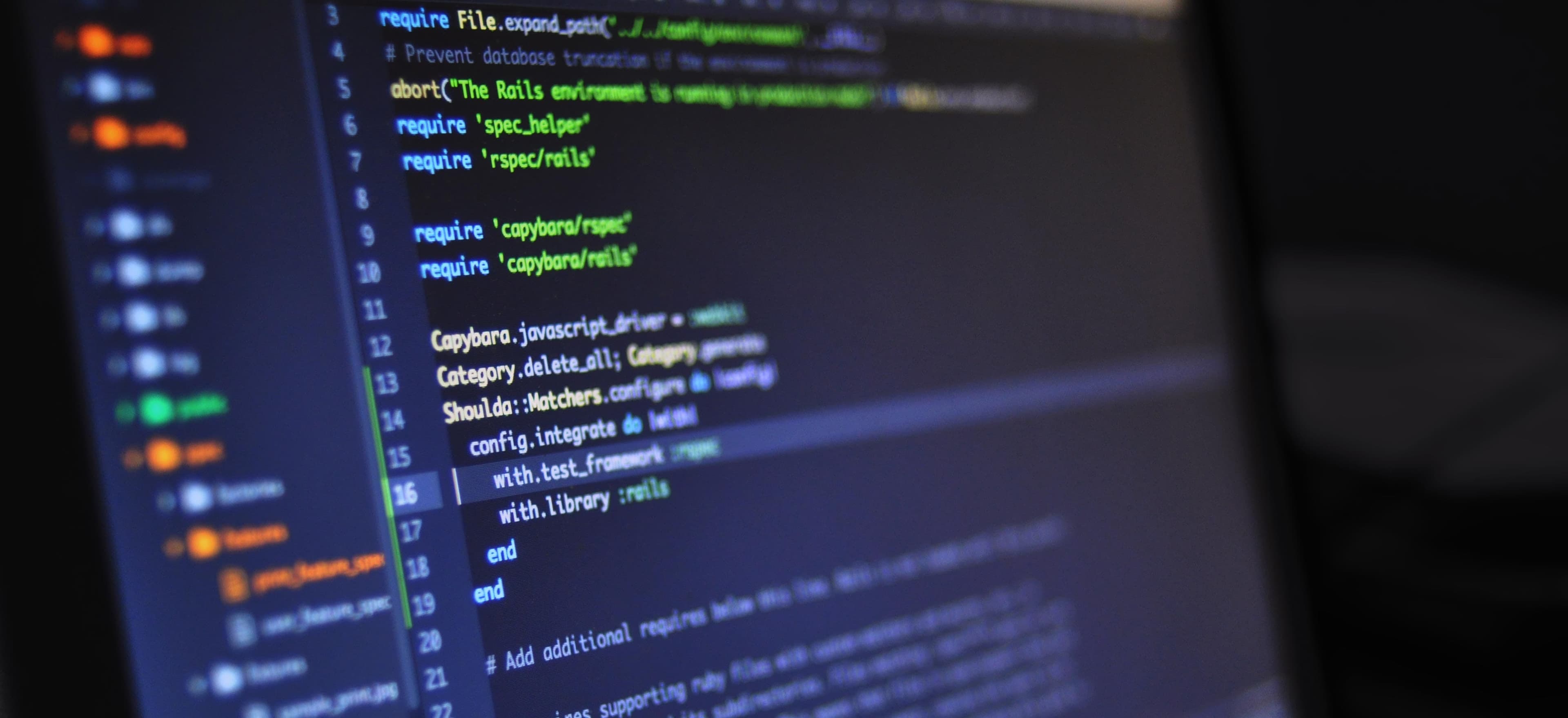
- Published on
Revamping Java UI: Mastering JMetro Combo Box
In the realm of Java UI, the JMetro library has emerged as a transformative tool for enhancing the aesthetics and functionality of applications. In this article, we will delve into the art of revamping Java UI by mastering the JMetro Combo Box. We will explore the steps to implement and customize the Combo Box component, offering insights and exemplary code to empower Java developers in elevating their user interfaces.
Introducing JMetro Combo Box
The Combo Box, a fundamental component in Java UI, serves as a versatile input control, allowing users to select from a predefined list of options. Leveraging the JMetro library, the Combo Box gains a modernized appearance, aligning with the Windows 10 design language. This not only enhances the visual appeal of the application but also contributes to a seamless user experience.
Getting Started with JMetro Combo Box
To begin, ensure that the JMetro library is integrated into your Java project. Maven users can simply add the following dependency to their pom.xml
file:
<dependency>
<groupId>com.dlsc.forms</groupId>
<artifactId>jmetro</artifactId>
<version>11.6.19</version>
</dependency>
If you are using Gradle, include the following in your build.gradle
file:
implementation 'com.dlsc.forms:jmetro:11.6.19'
Now, let's delve into the implementation of the JMetro Combo Box, starting with the instantiation and basic configuration.
import com.dlsc.forms.jfx.ComboBox;
import com.dlsc.forms.jmetro.JMetro;
// Instantiate the JMetro Combo Box
ComboBox<String> comboBox = new ComboBox<>();
// Add options to the Combo Box
comboBox.getItems().addAll("Option 1", "Option 2", "Option 3");
// Apply JMetro styling
JMetro jMetro = new JMetro(comboBox);
jMetro.setStyle(JMetro.Style.LIGHT); // Choose from LIGHT or DARK
The above code sets the foundation for a JMetro-styled Combo Box, integrating it into the application's UI and populating it with options. The JMetro
class is used to apply the Windows 10 design to the Combo Box, with the option to customize the style based on the desired theme.
Customizing JMetro Combo Box
A key advantage of JMetro is the ability to customize the appearance and behavior of the Combo Box. Let's explore various customization options, starting with the adjustment of the item rendering using cell factories.
// Custom rendering using cell factories
comboBox.setCellFactory((ListView<String> l) -> new ListCell<>() {
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (item == null || empty) {
setText(null);
} else {
setText(item);
setFont(Font.font("Verdana", 14)); // Custom font
setTextFill(Color.web("#2E2E2E")); // Custom text color
}
}
});
// Custom rendering for the selected item
comboBox.setButtonCell(new ListCell<>() {
@Override
protected void updateItem(String item, boolean empty) {
super.updateItem(item, empty);
if (item == null || empty) {
setText(null);
} else {
setText(item);
setFont(Font.font("Verdana", 14)); // Custom font
setTextFill(Color.web("#2E2E2E")); // Custom text color
}
}
});
The above code showcases how to customize the rendering of items within the Combo Box. By leveraging cell factories, we can tailor the appearance of the items and the selected item to align with the application's design and branding.
Furthermore, JMetro empowers developers to modify the styling of the Combo Box popup, offering a cohesive visual experience.
// Customize the Combo Box popup
comboBox.setPopupStyle("-fx-background-color: #F4F4F4; " +
"-fx-border-color: #BDBDBD; " +
"-fx-border-width: 1;");
In the provided code snippet, we override the default background and border colors of the Combo Box popup, aligning it with the overall color palette of the application.
Handling Events and Interactions
As with any UI component, the Combo Box necessitates seamless event handling and interaction management. JMetro facilitates the integration of event listeners, empowering developers to respond to user actions effectively.
// Event handling for Combo Box selection
comboBox.getSelectionModel().selectedItemProperty().addListener((obs, oldVal, newVal) -> {
if (newVal != null) {
System.out.println("Selected item: " + newVal);
// Additional logic based on selected item
}
});
In the above code, we set up a listener to capture the selection changes within the Combo Box. This enables the application to react dynamically to user selections, providing a responsive and engaging user experience.
In Conclusion, Here is What Matters
In conclusion, mastering the JMetro Combo Box empowers Java developers to elevate their UIs with a modern and polished aesthetic. By seamlessly integrating JMetro and leveraging its customization capabilities, developers can craft visually appealing and user-friendly Combo Box components that align with the Windows 10 design language.
As you embark on the journey of revamping Java UI, harness the power of JMetro to transform your Combo Box components, paving the way for exceptional user experiences.
Embrace the art of UI revamping with JMetro Combo Box - where functionality meets aesthetic finesse.
For further exploration, delve into the official JMetro documentation and expand your proficiency in crafting compelling Java UIs.
Are you ready to revamp your Java UI with JMetro Combo Box? Unleash the potential of modernized and customizable Combo Box components in your applications. Elevate the visual allure and user experience with JMetro.