Mastering Speed & Accuracy: Lambda Architecture Explained
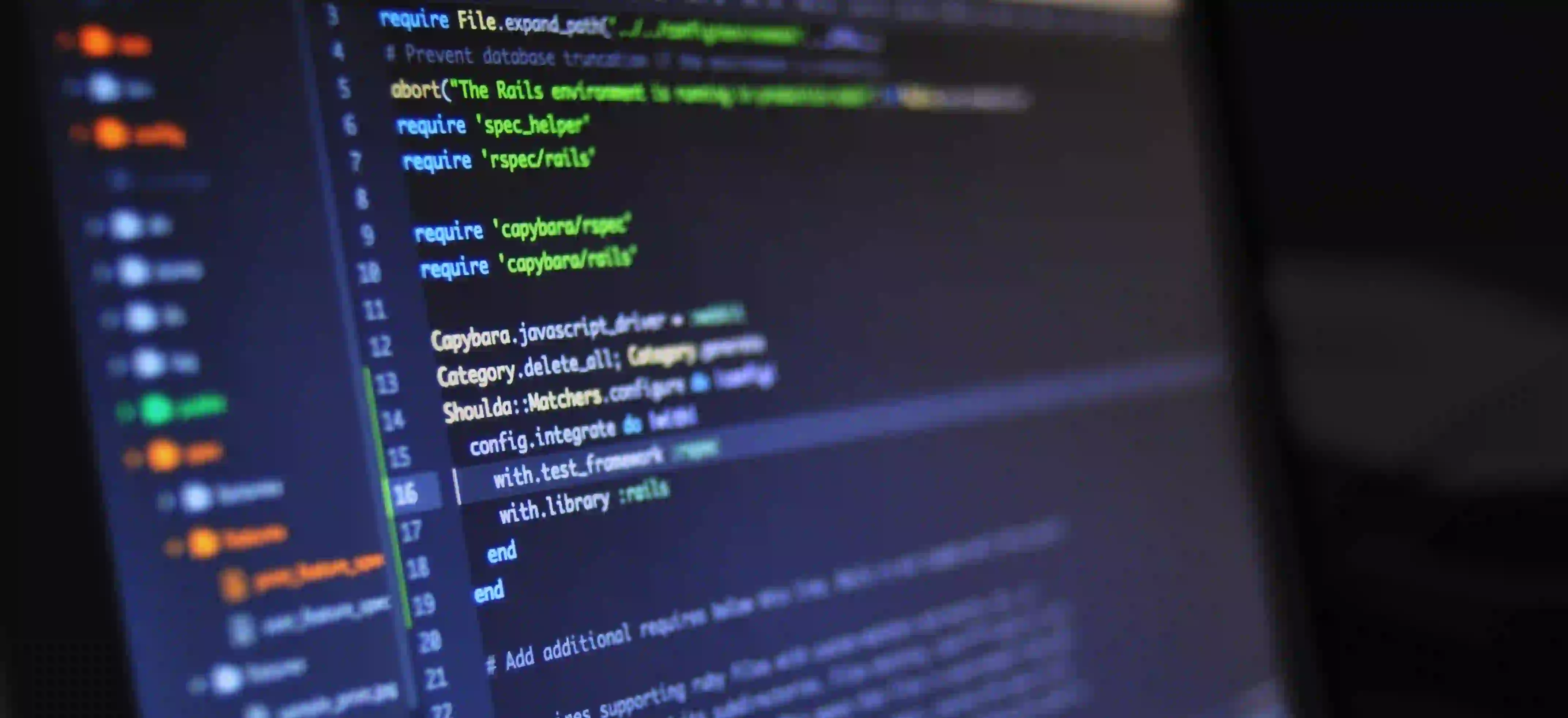
Mastering Speed & Accuracy: Lambda Architecture Explained
In the fast-paced world of big data and analytics, both speed and accuracy play a pivotal role in extracting valuable insights. This is where Lambda Architecture comes into the picture, a resilient, scalable, and fault-tolerant data processing architecture. It's designed to handle massive quantities of data by taking advantage of both batch and stream processing methods. In this blog post, we’ll unravel the mysteries of Lambda Architecture, providing you with a strong foundation and technical insights to leverage this architecture in your Java applications.
What is Lambda Architecture?
Lambda Architecture was proposed by Nathan Marz as a solution to the challenges of dealing with immense volumes of data while attempting to balance latency, throughput, and fault tolerance. The core idea behind this architecture is to run two parallel systems: one for processing real-time streaming data and another for batch processing. This dual approach ensures that you can perform comprehensive analytics and maintain the system’s integrity without sacrificing real-time data processing capabilities.
Key Components of Lambda Architecture
Lambda Architecture is built on three primary layers:
-
Batch Layer (Cold Path): Responsible for handling vast amounts of data in batches, focusing on comprehensive analysis, and storing an immutable, append-only set of raw data.
-
Speed Layer (Hot Path): Processes data in real-time, providing swift analytics but with potentially less accuracy.
-
Serving Layer: Merges the output from both the batch and speed layers to provide a comprehensive and accurate view of the data.
Implementing Lambda Architecture in Java
Java, with its robust ecosystem and performance, is an excellent choice for implementing Lambda Architecture. Let's explore how to apply this architecture in a Java environment with Apache Kafka for stream processing and Apache Hadoop for batch processing.
Prerequisites
Ensure you have the latest versions of Java Development Kit (JDK), Apache Kafka, and Apache Hadoop installed on your system. For installation instructions, you can visit the official Apache Kafka documentation and the official Apache Hadoop documentation.
Step 1: Setting Up the Batch Layer with Hadoop
The batch layer aims to provide comprehensive insights over large datasets. Apache Hadoop, with its HDFS for storage and MapReduce for processing, is an ideal choice for this layer.
Example MapReduce Job in Java:
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class WordCount {
public static class TokenizerMapper
extends Mapper<Object, Text, Text, IntWritable>{
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
StringTokenizer itr = new StringTokenizer(value.toString());
while (itr.hasMoreTokens()) {
word.set(itr.nextToken());
context.write(word, one);
}
}
}
public static class IntSumReducer
extends Reducer<Text,IntWritable,Text,IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values,
Context context
) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
result.set(sum);
context.write(key, result);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(WordCount.class);
job.setMapperClass(TokenizerMapper.class);
job.setCombinerClass(IntSumReducer.class);
job.setReducerClass(IntSumReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
This code snippet demonstrates a simple MapReduce job in Java for word count analysis. It emphasizes the methodology of batch processing in Lambda Architecture.
Step 2: Establishing the Speed Layer with Kafka
For processing real-time streaming data, Apache Kafka is a powerful tool that can handle high-throughput, low-latency data feeds. Kafka Streams, a client library for building applications and microservices, allows for real-time data processing within the Kafka ecosystem.
Example Kafka Streams Application in Java:
import org.apache.kafka.common.serialization.Serdes;
import org.apache.kafka.streams.KafkaStreams;
import org.apache.kafka.streams.StreamsBuilder;
import org.apache.kafka.streams.StreamsConfig;
import org.apache.kafka.streams.kstream.KStream;
import java.util.Properties;
public class StreamProcessor {
public static void main(final String[] args) {
Properties props = new Properties();
props.put(StreamsConfig.APPLICATION_ID_CONFIG, "stream-processor");
props.put(StreamsConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(StreamsConfig.DEFAULT_KEY_SERDE_CLASS_CONFIG, Serdes.String().getClass());
props.put(StreamsConfig.DEFAULT_VALUE_SERDE_CLASS_CONFIG, Serdes.String().getClass());
StreamsBuilder builder = new StreamsBuilder();
KStream<String, String> source = builder.stream("input-topic");
source.mapValues(value -> value.toUpperCase()).to("output-topic");
KafkaStreams streams = new KafkaStreams(builder.build(), props);
streams.start();
}
}
This code snippet highlights real-time processing of streaming data using Kafka Streams. It takes inputs from a Kafka topic, transforms the data in real-time, and outputs to another topic.
Step 3: Combining Batch and Speed Layers
The Serving Layer is where the outputs from the batch and speed layers are merged to provide a comprehensive view of the data. This layer often involves a combination of storage and querying capabilities to deliver accurate and timely results to end-users or downstream applications.
A common approach is to use Apache HBase or Cassandra for storing the processed data and enabling quick, efficient access to the integrated view of the data.
Best Practices and Considerations
When implementing Lambda Architecture, especially in a Java environment, consider the following best practices:
- Scalability: Design your system to scale horizontally by adding more nodes, ensuring it can handle increasing data volumes.
- Fault Tolerance: Employ redundancy and recovery mechanisms, such as checkpointing in Kafka Streams, to ensure system resilience.
- Maintenance: Be cautious about the complexity of maintaining two parallel systems. Automation and careful monitoring are key.
- Data Freshness: Adjust the frequency of batch processing and the latency of stream processing to balance between data comprehensiveness and timeliness.
- Unified Data Model: Strive for a consistent data model across both layers to simplify the merging logic in the Serving Layer.
Closing the Chapter
Lambda Architecture offers a robust framework for dealing with massive datasets, allowing for both thorough, batch-based analytics and real-time data processing. By leveraging Java alongside technologies like Apache Kafka and Apache Hadoop, developers can implement high-performance, resilient data processing systems. Understanding the principles and components of Lambda Architecture is crucial for architects and developers aiming to master the balance between speed and accuracy in big data analytics.
For those looking to explore further, diving deep into Apache Kafka’s documentation or exploring Apache Hadoop's capabilities are excellent next steps. Embracing Lambda Architecture with Java can unlock new possibilities in data processing, driving insights and value at unprecedented scales and speeds.