Overcoming JSON Storage Limitations in Postgres
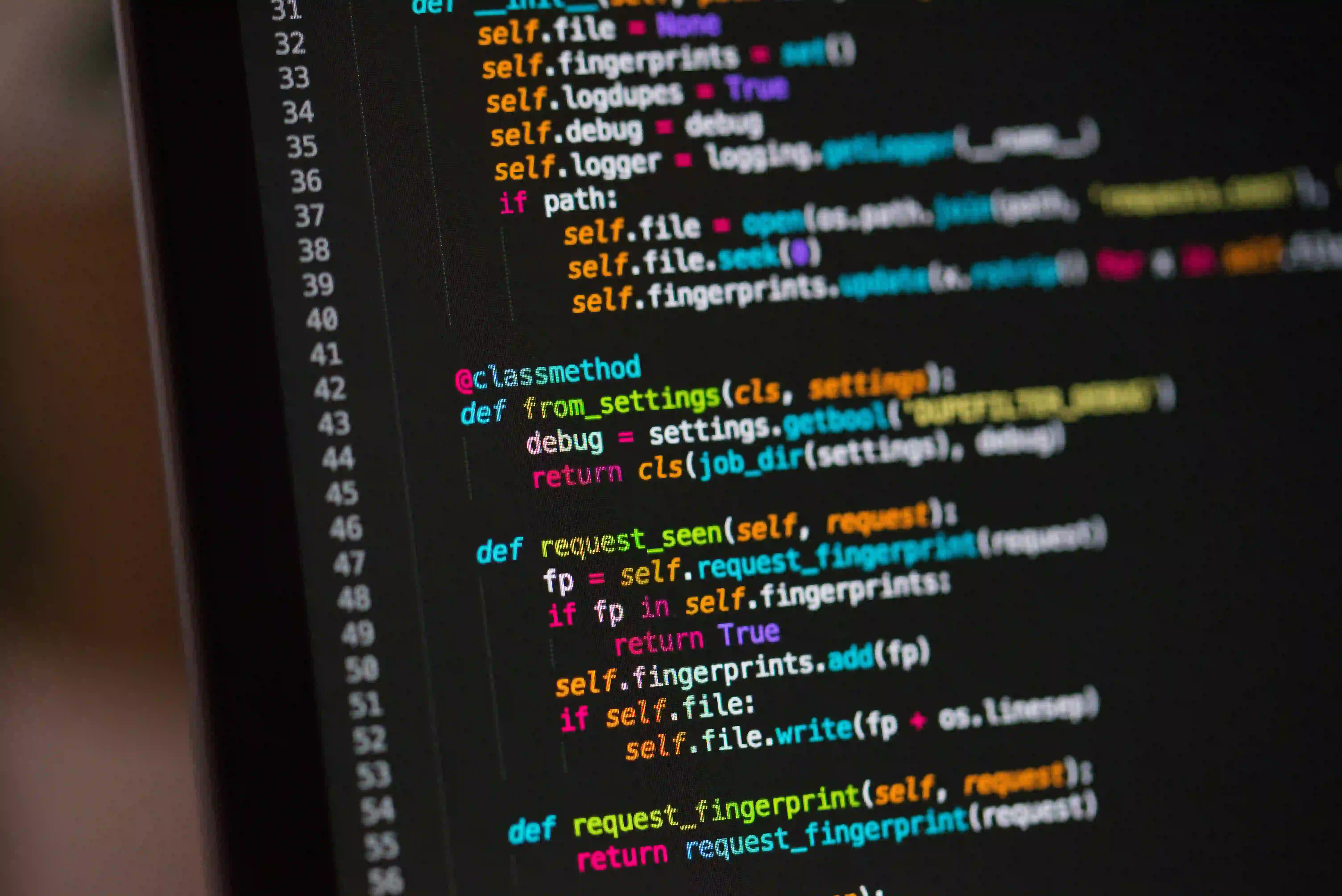
Overcoming JSON Storage Limitations in Postgres
When it comes to database management, PostgreSQL is a popular choice due to its robust features, reliability, and extensibility. One of its standout features is the ability to store and query JSON data. However, there are limitations when it comes to storing large JSON objects in PostgreSQL. In this article, we will explore these limitations and discuss strategies for overcoming them.
Understanding the JSONB Data Type
In PostgreSQL, JSON data can be stored using the jsonb
data type. This data type allows for efficient storage, indexing, and querying of JSON data. However, there is a default size limit for JSONB objects in PostgreSQL, which is set by the max TOAST chunk size
configuration parameter.
Working with Large JSON Objects
When dealing with large JSON objects that exceed the default size limit, you may encounter errors such as "out of memory" or "value too long for type character varying." These errors indicate that the JSONB object exceeds the storage limitations set by PostgreSQL.
Overcoming Storage Limitations
1. Using External Storage
One approach to overcome the storage limitations of JSON objects in PostgreSQL is to use external storage. Instead of storing the entire JSON object in the database, you can store the object in a file system or distributed file storage system, such as Amazon S3 or Google Cloud Storage. In the database, you can store a reference to the external location of the JSON file, allowing you to retrieve the JSON data when needed.
2. Data Normalization
Another strategy is to normalize the JSON data by breaking it down into smaller, more manageable pieces. Instead of storing a single large JSON object, you can break it down into separate tables and establish relationships between them using foreign keys. This approach not only helps overcome storage limitations but also improves query performance and data integrity.
3. Compression Techniques
PostgreSQL provides support for data compression using techniques such as TOAST (The Oversized-Attribute Storage Technique). By compressing JSON data, you can effectively reduce its storage footprint within the database. This approach can be particularly useful when working with repetitive or redundant JSON structures.
Implementing External Storage in Java
Let's explore how we can implement the external storage approach using Java and PostgreSQL.
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
public class JsonStorageDemo {
public static void main(String[] args) {
String url = "jdbc:postgresql://localhost:5432/mydatabase";
String user = "username";
String password = "password";
try (Connection connection = DriverManager.getConnection(url, user, password)) {
String jsonFilePath = "s3://bucketname/jsondata.json"; // Replace with actual external storage location
String query = "INSERT INTO json_storage (json_data_url) VALUES (?)";
try (PreparedStatement statement = connection.prepareStatement(query)) {
statement.setString(1, jsonFilePath);
statement.executeUpdate();
System.out.println("JSON data reference stored successfully.");
} catch (SQLException e) {
System.err.println("Error executing SQL statement: " + e.getMessage());
}
} catch (SQLException e) {
System.err.println("Error connecting to the database: " + e.getMessage());
}
}
}
In this Java example, we establish a connection to the PostgreSQL database and store a reference to the external location of the JSON data using a prepared statement. This demonstrates how Java can be used to implement the external storage approach for overcoming JSON storage limitations in PostgreSQL.
In Conclusion, Here is What Matters
PostgreSQL's support for JSON data provides flexibility and versatility, but it's important to be aware of the storage limitations when working with large JSON objects. By leveraging strategies such as external storage, data normalization, and compression techniques, you can overcome these limitations and effectively manage large JSON data in PostgreSQL. Additionally, using Java to interact with PostgreSQL allows for seamless implementation of these strategies within your applications.
As you continue to work with JSON data in PostgreSQL, it's crucial to stay updated with the latest advancements and best practices in database management. Incorporating these strategies will not only expand your knowledge but also enhance the efficiency and scalability of your database architecture.
To delve deeper into JSON data handling in PostgreSQL, you can explore the official documentation on PostgreSQL JSON functions and operators and PostgreSQL JSONB functions and operators.
By understanding the limitations and employing effective strategies, you can optimize the storage and retrieval of JSON data in PostgreSQL, ensuring the seamless operation and performance of your applications.