Maximizing Efficiency: How EJB Timeout Policies Aid Performance
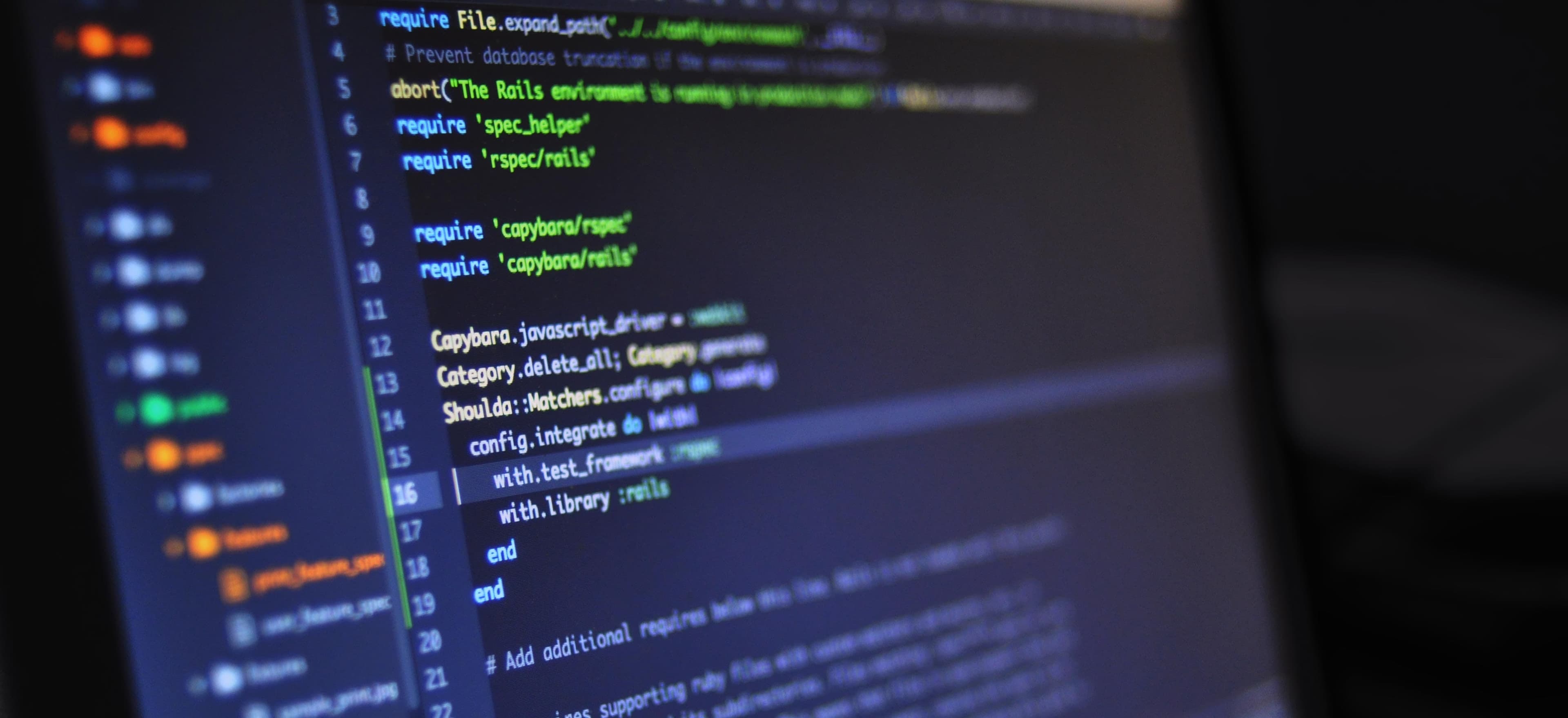
- Published on
Maximizing Efficiency: How EJB Timeout Policies Aid Performance
In the world of enterprise application development, ensuring optimal performance is paramount. This is where Enterprise JavaBeans (EJB) timeout policies come into play. EJBs are server-side, modular, reusable components that encapsulate the business logic of an application. They provide a robust and scalable framework for building distributed and transactional applications in Java. One crucial aspect of EJBs is their timeout policies, which play a significant role in maximizing efficiency and improving overall performance.
Understanding EJB Timeout and Its Importance
Timeouts occur when a method invocation on an EJB takes longer than the specified duration to complete. Such delays may arise due to network latency, database operations, or intensive computation. Identifying and addressing these timeouts is vital for maintaining a responsive and high-performing application.
EJBs provide a mechanism to define and control timeout behavior through the @Timeout
annotation and the javax.ejb.Timeout
interface. By utilizing timeout policies, developers can effectively manage long-running processes and resource-intensive operations within their EJBs. This not only enhances application responsiveness but also prevents resource wastage and potential bottlenecks.
The Role of EJB Timeout Policies in Performance Optimization
Optimizing Resource Utilization
EJB timeout policies enable developers to optimize resource allocation and utilization within their applications. By setting appropriate timeout values, they can ensure that system resources are efficiently utilized, preventing excessive consumption by long-running or stalled processes. This proactive management of resources contributes significantly to the overall performance and stability of the application.
Mitigating Performance Bottlenecks
In distributed environments, delays in method invocations can lead to performance bottlenecks, especially in scenarios involving remote EJB invocations or complex interactions with external systems. EJB timeout policies act as a safeguard against such bottlenecks, allowing developers to proactively address and mitigate potential performance issues. By establishing sensible timeout configurations, developers can preemptively tackle bottlenecks and maintain a smooth, uninterrupted flow of operations.
Enhancing Scalability
Efficient management of timeouts is pivotal for ensuring the scalability of EJB-based applications. By leveraging timeout policies, developers can design their applications to gracefully handle increasing loads without compromising responsiveness. This proactive approach to scalability empowers applications to adapt to growing user demands and concurrent requests, thereby bolstering their overall performance and user experience.
Implementing EJB Timeout Policies in Java
Let's delve into the implementation of EJB timeout policies in Java. Consider a scenario where a critical EJB method needs to be safeguarded against potential performance issues caused by prolonged execution times.
@Stateless
public class MyTimeoutEJB {
@Resource
private SessionContext context;
public void performTaskWithTimeout() {
TimerService timerService = context.getTimerService();
// Set the timeout duration to 5 seconds
Timer timer = timerService.createTimer(5000, "Timeout occurred");
}
@Timeout
public void handleTimeout(Timer timer) {
// Handle timeout event
}
}
In the above example, the MyTimeoutEJB
class demonstrates the use of EJB timeout policies. The performTaskWithTimeout
method initiates a timer with a specified duration of 5 seconds, and the handleTimeout
method is invoked if the operation exceeds this duration.
By employing such timeout configurations, developers can proactively manage the execution of critical EJB methods and prevent potential performance bottlenecks.
Best Practices for EJB Timeout Policies
To harness the full potential of EJB timeout policies for performance optimization, it's essential to adhere to best practices:
-
Set Sensible Timeout Values: Define timeout values based on the nature of the operations and the expected response times. Avoid excessively short or long timeouts that could either trigger premature timeouts or lead to prolonged delays.
-
Graceful Handling of Timeouts: Implement robust error-handling mechanisms to gracefully manage timeouts. This may involve retry strategies, fallback mechanisms, or informative logging to facilitate troubleshooting.
-
Monitor and Analyze Timeout Occurrences: Leverage monitoring tools and logging frameworks to track and analyze timeout occurrences. This insight can aid in identifying performance hotspots and fine-tuning timeout configurations.
-
Consider Asynchronous Processing: Evaluate the suitability of asynchronous processing for long-running tasks to decouple execution from the invoking thread, thereby minimizing the impact of potential timeouts.
-
Test and Tune: Thoroughly test and tune timeout configurations under varying load and network conditions to validate their effectiveness in real-world scenarios.
Lessons Learned
In conclusion, EJB timeout policies play a pivotal role in maximizing the efficiency and performance of enterprise applications developed using Java. By strategically defining and managing timeout behaviors, developers can proactively address performance bottlenecks, optimize resource utilization, and enhance the scalability of their applications. Adhering to best practices and diligently fine-tuning timeout configurations further empowers developers to deliver highly responsive and robust EJB-based solutions.
Efficient utilization of EJB timeout policies not only elevates the performance of applications but also contributes to a seamless and rewarding user experience. Embracing these practices equips developers with the tools to build resilient, scalable, and high-performing enterprise applications in Java.
Harness the power of EJB timeout policies to propel your Java-based applications to new heights of efficiency and performance!
Checkout our other articles