Mastering Eclipse Plugins: Avoid Common Pitfalls!
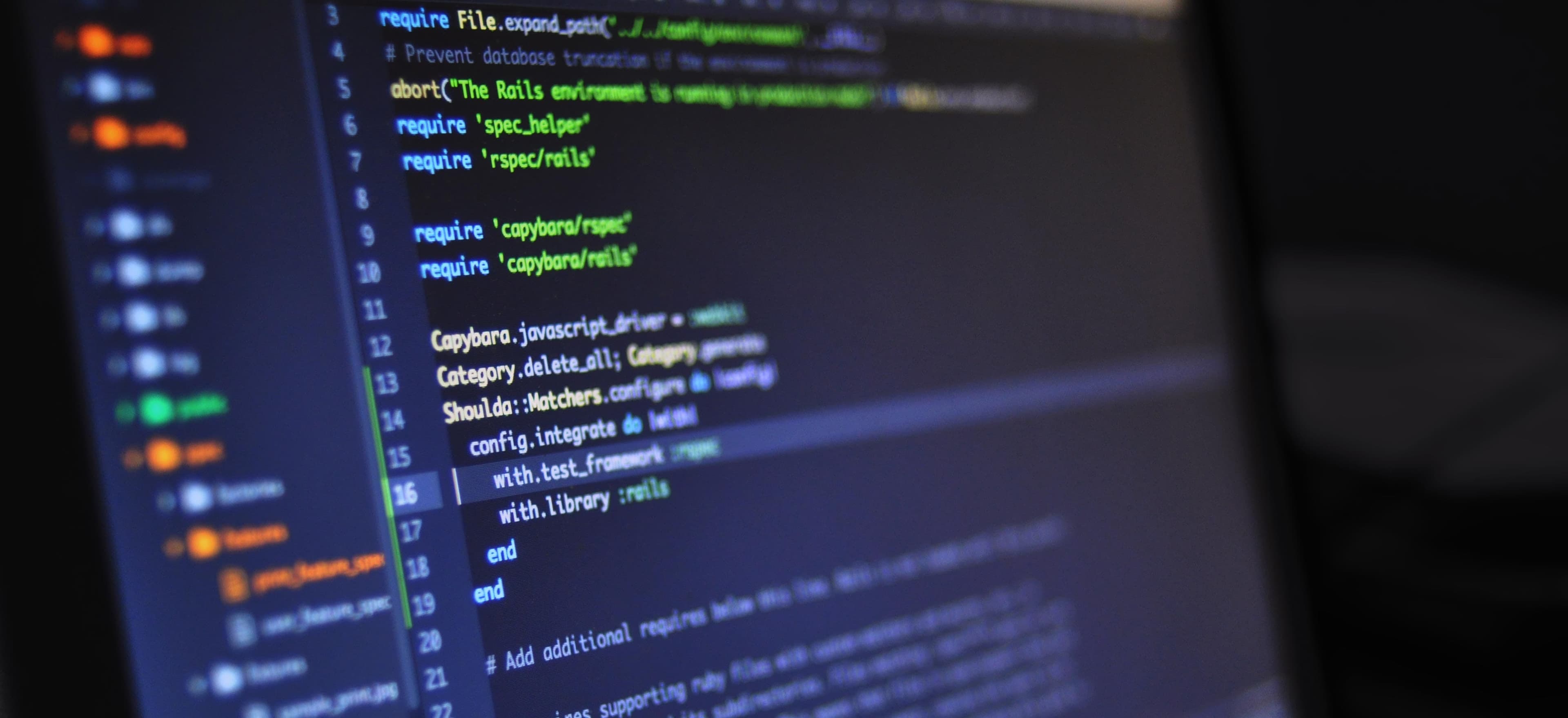
- Published on
Mastering Eclipse Plugins: Avoid Common Pitfalls!
If you are a Java developer working on building Eclipse plugins, you know that the process can be both rewarding and challenging. Eclipse, as a robust integrated development environment, offers a wide range of functionalities through its plugin architecture. However, developing plugins for Eclipse comes with its own set of challenges and potential pitfalls. In this article, we will explore some common pitfalls when developing Eclipse plugins in Java and how to avoid them.
1. Understanding the Plugin Lifecycle
One common pitfall when developing Eclipse plugins is not fully understanding the plugin lifecycle. Eclipse plugins follow a specific lifecycle that includes activation, deactivation, and destruction phases. It's crucial to understand when and how your plugin is activated and deactivated to ensure proper functionality and resource management.
Let's take a look at a simple plugin activation and deactivation to understand the lifecycle better:
public class MyPluginActivator implements BundleActivator {
@Override
public void start(BundleContext context) throws Exception {
// Plugin activation logic
}
@Override
public void stop(BundleContext context) throws Exception {
// Plugin deactivation logic
}
}
In the above code, start()
method is called when the plugin is activated, and stop()
method is called when the plugin is deactivated. Understanding and utilizing these lifecycle methods correctly can prevent various issues related to resource management and unexpected behavior.
2. Dealing with UI Thread Constraints
When developing Eclipse plugins that involve user interface interactions, it's essential to be mindful of the UI thread constraints. In Eclipse, any operations that manipulate the user interface must be executed on the UI thread to avoid concurrency issues and ensure smooth user experience.
Here's an example of how to execute a task on the UI thread using Display
class:
Display.getDefault().asyncExec(() -> {
// UI-related task
});
By using Display.asyncExec()
, you can safely execute UI-related tasks on the UI thread, thereby avoiding potential UI freezing and responsiveness issues.
3. Proper Resource Management
Another common pitfall in Eclipse plugin development is improper resource management. Failing to release resources such as file handles, database connections, or memory allocations can lead to memory leaks and degraded performance over time.
To address this, it's important to use try-with-resources for resource management wherever applicable. For example, when working with file I/O operations:
try (FileInputStream fis = new FileInputStream("example.txt")) {
// File processing logic
} catch (IOException e) {
// Exception handling
}
Using try-with-resources ensures that the associated system resources are properly closed after the execution, preventing resource leaks and potential system instability.
4. Handling Plugin Dependencies
Managing dependencies between Eclipse plugins is crucial for ensuring the stability and compatibility of the overall system. One common pitfall is not handling plugin dependencies correctly, which can result in runtime errors and unexpected behaviors.
When defining plugin dependencies in the MANIFEST.MF
file, it's essential to specify the required plugins and their versions accurately. Additionally, leveraging tools like Maven or Gradle for dependency management can streamline the process and reduce potential issues.
5. Testing and Debugging
Testing and debugging Eclipse plugins pose unique challenges due to the complex nature of the Eclipse platform. One common pitfall is not investing enough effort into testing and debugging, leading to unresolved issues and poor plugin quality.
Utilizing Eclipse's built-in tools such as JUnit for testing and the debugging capabilities for identifying and resolving issues is essential. Additionally, writing unit tests for plugin components and using the Eclipse plugin development environment for debugging can significantly improve the robustness of the plugin.
In Conclusion, Here is What Matters
Developing Eclipse plugins in Java offers a wide range of possibilities but also presents specific challenges that developers must be mindful of. By understanding the plugin lifecycle, handling UI thread constraints, practicing proper resource management, managing plugin dependencies, and emphasizing testing and debugging, developers can avoid common pitfalls and build high-quality Eclipse plugins that integrate seamlessly with the Eclipse platform.
Remember, mastering Eclipse plugin development takes time and practice, but the payoff is well worth the effort.
In conclusion, building Eclipse plugins in Java can be both challenging and rewarding. By understanding and addressing these common pitfalls, you can create high-quality plugins that enhance the Eclipse development experience.
Now that you've gained insights into avoiding common pitfalls, you can take your Eclipse plugin development to the next level with confidence. Have fun mastering Eclipse plugins!
Happy coding!
Here is an in-depth tutorial on Eclipse Plugin development to further enhance your understanding.
And don't forget to check out the Eclipse community's newsletter for the latest updates and tips on plugin development.