Overcoming JAX-RS and Jersey Integration Issues
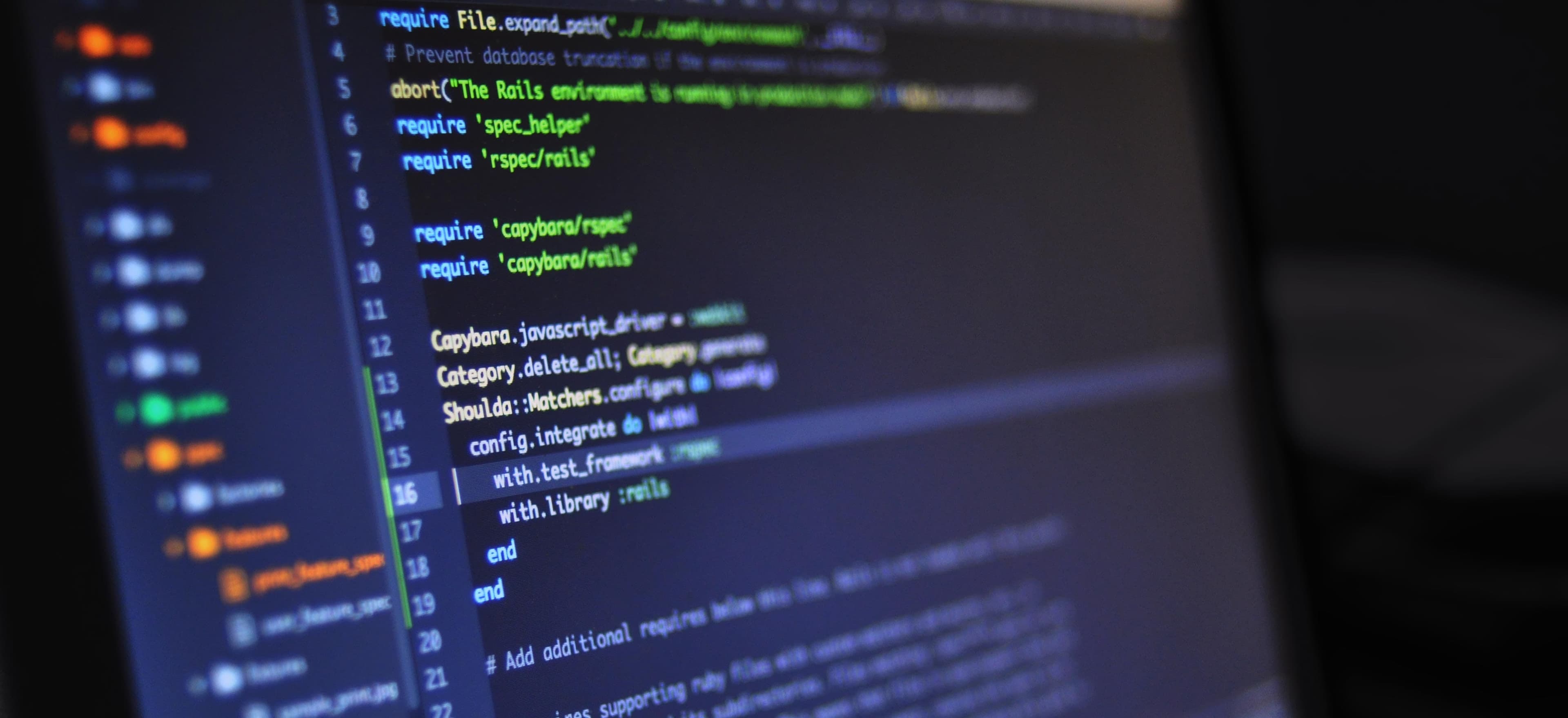
- Published on
Overcoming JAX-RS and Jersey Integration Issues
When developing a Java web application, integrating JAX-RS (Java API for RESTful Web Services) with Jersey is a common and powerful choice. However, with great power comes great responsibility, and integrating these technologies may encounter some issues. In this article, we'll discuss common integration issues between JAX-RS and Jersey and how to overcome them, ensuring a smooth and efficient development process.
Understanding JAX-RS and Jersey
Before diving into the integration issues, let's briefly understand what JAX-RS and Jersey are.
-
JAX-RS: It's a set of APIs for creating web services using the REST architecture. JAX-RS provides a convenient way to map Java methods to HTTP methods and build RESTful web services.
-
Jersey: It's an open-source, production-quality framework for building RESTful web services in Java. Jersey provides an implementation of JAX-RS APIs and extends it with additional features and utilities.
Now that we have an understanding of JAX-RS and Jersey, let's explore some common integration issues and their solutions.
Common Integration Issues and Solutions
Issue 1: ClassNotFoundException or NoClassDefFoundError
One of the most common issues developers encounter when integrating JAX-RS and Jersey is related to runtime class loading. This issue occurs when the required JAR files or dependencies are not included in the application's classpath.
Solution:
Ensure that the necessary Jersey JAR files are included in the project's classpath. You can use tools like Maven or Gradle to manage dependencies effectively. Here's an example of how to add Jersey dependencies in a Maven pom.xml
file:
<dependencies>
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet</artifactId>
<version>2.3.1</version>
</dependency>
<!-- Add other necessary dependencies -->
</dependencies>
By including the required dependencies, you can resolve the ClassNotFoundException
or NoClassDefFoundError
issues.
Issue 2: Conflicting JAX-RS Implementations
Another common issue arises when there are conflicts between multiple JAX-RS implementations. For example, an application may have multiple libraries or frameworks that include their own JAX-RS implementations, leading to conflicts and runtime errors.
Solution:
Exclude any additional JAX-RS implementations from the dependencies and ensure that only the required Jersey implementation is used. You can use the exclusions
tag in Maven to exclude specific dependencies. Here's an example:
<dependency>
<groupId>some.group</groupId>
<artifactId>conflicting-jaxrs-library</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>javax.ws.rs</groupId>
<artifactId>javax.ws.rs-api</artifactId>
</exclusion>
</exclusions>
</dependency>
By excluding conflicting JAX-RS implementations, you can avoid runtime conflicts and ensure the seamless integration of Jersey with JAX-RS.
Issue 3: Incorrect Configuration in web.xml
Sometimes, integration issues stem from incorrectly configured web.xml
files. This can lead to Jersey not being properly initialized or mapped to the application, resulting in 404 errors when accessing the REST resources.
Solution:
Ensure that the web.xml
file contains the necessary configuration to initialize Jersey servlet and map the REST resources correctly. Here's an example of the web.xml
configuration for Jersey:
<servlet>
<servlet-name>JerseyServlet</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.example.resources</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JerseyServlet</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
By ensuring correct configuration in the web.xml
file, Jersey can be properly initialized and the REST resources can be accessed without any 404 errors.
Issue 4: Resource Endpoint Not Found
Another common issue is when the resource endpoints are not found or the resource methods are not invoked as expected. This can occur due to incorrect package scanning or resource registration.
Solution:
Ensure that the resource packages are scanned and registered correctly with Jersey. You can use the jersey.config.server.provider.packages
parameter in the web.xml
configuration to specify the packages to be scanned for resources. Additionally, you can annotate your resource classes with @Path
to explicitly define the resource endpoints.
@Path("/users")
public class UserResource {
@GET
@Path("/{id}")
public Response getUserById(@PathParam("id") String userId) {
// Implementation
}
}
By correctly specifying the resource packages and annotating the resource classes, you can ensure that the resource endpoints are found and the methods are invoked as expected.
Issue 5: Dependency Injection Not Working
In some cases, dependency injection may not work as expected within the JAX-RS resource classes. This can happen due to incorrect configuration or missing dependency injection annotations.
Solution:
Ensure that the dependency injection framework, such as HK2 (Hypersonic 2), is properly configured and enabled for JAX-RS resources. Additionally, make sure to use annotations like @Inject
to inject dependencies into the resource classes.
public class UserResource {
@Inject
private UserService userService;
// Resource methods using userService
}
By correctly configuring the dependency injection framework and using the @Inject
annotation, you can enable dependency injection within the JAX-RS resource classes.
The Bottom Line
In this article, we've explored common integration issues between JAX-RS and Jersey and provided solutions to overcome these issues. By addressing issues related to class loading, conflicting implementations, configuration, resource endpoints, and dependency injection, developers can ensure a smooth integration of JAX-RS with Jersey in their Java web applications.
Effective management of dependencies, proper configuration, and adherence to best practices play a vital role in overcoming integration issues and building robust and efficient RESTful web services using JAX-RS and Jersey.
By understanding and addressing these integration challenges, developers can harness the full potential of JAX-RS and Jersey to create high-performance, scalable, and maintainable web applications in the Java ecosystem.
Remember, mastering the integration of JAX-RS and Jersey not only leads to efficient RESTful web services but also to a deeper understanding of the Java web development landscape.