Balancing Act: Iterative vs Incremental Development
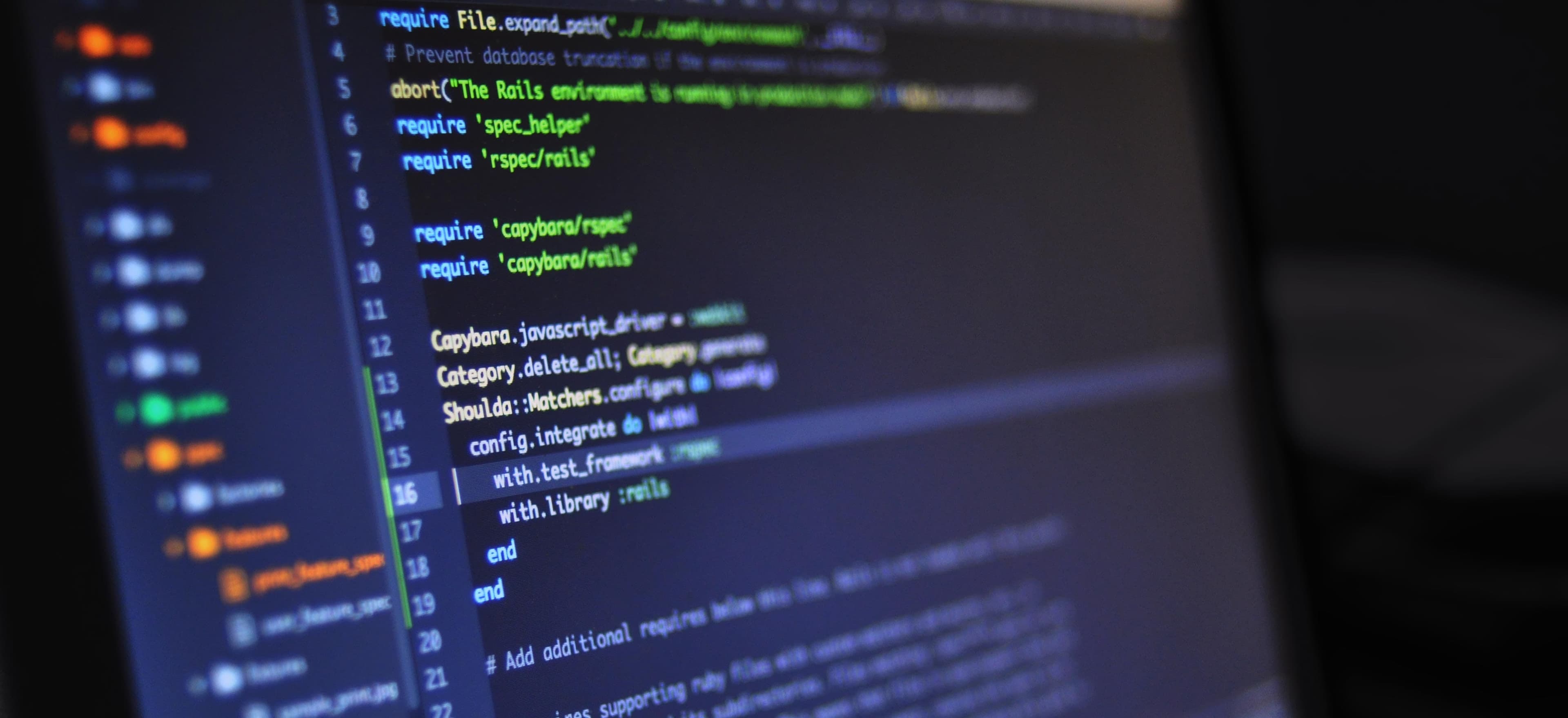
- Published on
Balancing Act: Iterative vs Incremental Development in Java
In the realm of software development, choosing the right methodology is crucial for the success of a project. Two popular approaches, iterative development and incremental development, offer distinct methodologies for Java projects. In this article, we'll delve into these two methodologies, explore their characteristics, provide examples in Java, and discuss their implications for Java development projects.
Understanding Iterative Development
Iterative development is a software development methodology where the project is broken down into small parts, and each part goes through the whole development process, including planning, requirements, design, coding, and testing. This approach allows for repeated cycles, each resulting in an improved version of the software. Iterative development is characterized by its flexibility, adaptability to changes, and customer involvement throughout the development process.
In Java development, the iterative approach can be advantageous, particularly for complex projects where requirements may evolve, or when the overall scope might not be fully understood at the outset. By breaking the project into manageable iterations, developers can ensure that the software aligns with the changing needs of the stakeholders.
Example: Iterative Development in Java
Let's consider a simple example to illustrate an iterative approach in Java. Suppose we are developing a program to generate Fibonacci series. We start with a simple implementation and then iteratively add features to optimize and enhance the functionality.
public class FibonacciSeries {
public static void main(String[] args) {
int n = 10, firstTerm = 0, secondTerm = 1;
System.out.println("Fibonacci Series:");
for (int i = 1; i <= n; ++i) {
System.out.print(firstTerm + ", ");
int nextTerm = firstTerm + secondTerm;
firstTerm = secondTerm;
secondTerm = nextTerm;
}
}
}
In this iterative example, we start by simply generating the first 10 numbers in the Fibonacci series. Then, in successive iterations, we can enhance the program by allowing the user to input the number of terms to generate, implementing error handling, and even optimizing the performance through better algorithms. This iterative process ensures that each cycle delivers a more robust and effective solution.
Understanding Incremental Development
Incremental development is a method where the systems are developed and delivered in small portions over time. Each increment builds on the previous functionality, gradually adding new features. This approach allows for partial delivery of the system to the stakeholders after each increment, providing early feedback and the opportunity to adjust requirements based on the delivered functionality.
In Java projects, incremental development can offer several benefits, especially when stakeholders require visibility into the project's progress and want early access to functional parts of the system. Additionally, it can help mitigate risks by providing an early opportunity to identify and rectify any deviations from the project's objectives.
Example: Incremental Development in Java
To demonstrate incremental development in Java, let's consider a hypothetical project to build a simple e-commerce website. We can start with a basic product catalog and then incrementally add features such as shopping cart functionality, user authentication, payment processing, and order management.
// Increment 1: Basic Product Catalog
public class Product {
private String name;
private double price;
// Constructors, getters, and setters
// ...
}
public class ProductCatalog {
private List<Product> products;
// Methods to manage and display products
// ...
}
// Increment 2: Shopping Cart Functionality
public class ShoppingCart {
private List<Product> items;
// Methods to add, remove, and display items
// ...
}
// Increment 3: User Authentication
// ...
// Increment 4: Payment Processing
// ...
// Increment 5: Order Management
// ...
In this example, each increment adds new functionality to the e-commerce website, enhancing its capabilities over time. This incremental approach allows for a phased rollout of the website's features, catering to the evolving needs of both the developers and the stakeholders.
Comparing Iterative and Incremental Development
While both iterative and incremental development share the goal of delivering high-quality software in a flexible and adaptable manner, they differ in their approach.
Characteristics
-
Iterative Development:
- Emphasizes delivering a complete, tested, and integrated system in small increments.
- Allows for changes in requirements and features at any point in the development process.
- Involves multiple cycles of development, each delivering an improved version of the software.
- Enables continuous refinement and improvement of the project.
-
Incremental Development:
- Emphasizes delivering working subsets of the system in increments.
- Enables early and partial delivery of the system for feedback and evaluation.
- Adds new features in a phased manner, building on the existing functionality.
- Provides visibility into the project's progress and fosters stakeholder involvement throughout the development process.
Context within Java Development
In the context of Java development, both methodologies offer unique advantages. Iterative development is well-suited for projects with evolving requirements or where the overall scope of the project is not fully defined from the beginning. On the other hand, incremental development can be beneficial when stakeholders require early access to functional components of the system to provide feedback or when there is a need to manage risks through phased delivery.
Best Practices for Combining Iterative and Incremental Development in Java
While these methodologies have their strengths when used independently, combining iterative and incremental development can offer a comprehensive solution for Java projects with diverse needs. A hybrid approach can provide the flexibility to adapt to changing requirements while also delivering functional subsets of the system at regular intervals.
Case Study: Java Project Utilizing Both Approaches
Let's consider a real-world scenario where a team is developing a Java-based financial management application. In this case, the team decides to adopt a hybrid approach, leveraging both iterative and incremental methodologies.
During the iterative phase, the team focuses on building core functionalities such as user authentication, transaction processing, and reporting. Each iteration involves refining existing features and incorporating new requirements based on user feedback and emerging business needs.
In parallel, the incremental approach is employed to deliver incremental versions of the application to the stakeholders. The first increment may focus on basic account management, followed by subsequent increments that introduce features for investment tracking, budgeting, and forecasting. This phased delivery allows stakeholders to evaluate the application's functionality early and provide feedback for further iterations.
By combining these methodologies, the team achieves the dual advantages of adaptability to changing requirements and early delivery of core functionalities, resulting in a well-rounded and responsive financial management application.
In Conclusion, Here is What Matters
In the dynamic landscape of software development, the choice between iterative and incremental development can significantly impact the success of a Java project. While each methodology offers distinct advantages, the best outcomes often stem from combining these approaches to form a hybrid methodology tailored to the specific needs of the project.
By understanding the characteristics and implications of iterative and incremental development, Java developers can make informed decisions when selecting the most suitable approach for their projects. Furthermore, experimentation with a hybrid methodology can unlock new possibilities for delivering high-quality, adaptive, and stakeholder-centric software solutions.
We encourage you to share your experiences and thoughts on utilizing iterative and incremental development in Java projects. How has your team found success in balancing these methodologies? Share your insights in the comments below!