Troubleshooting Spring MVC Error Handling
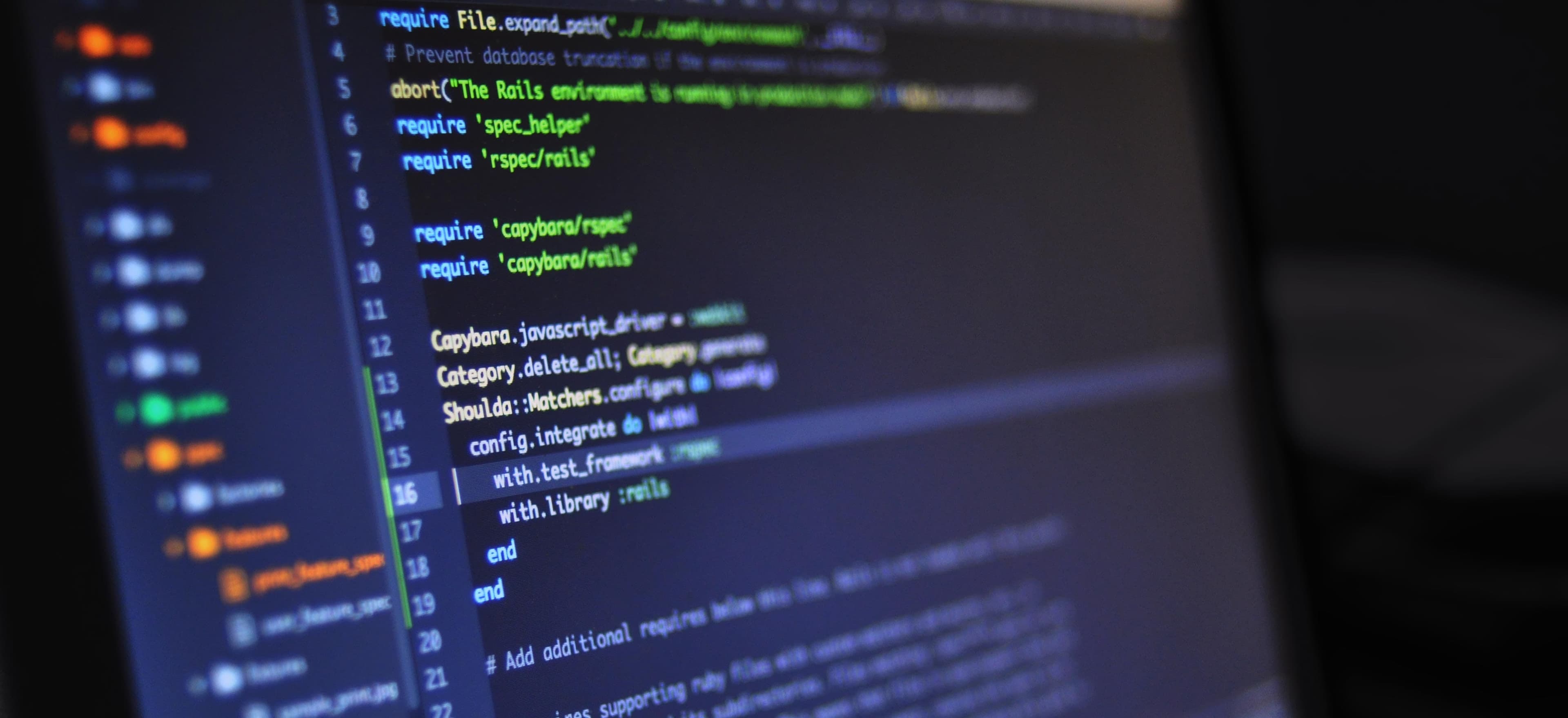
- Published on
Troubleshooting Spring MVC Error Handling
In a Spring MVC application, effective error handling is crucial for delivering a smooth user experience. When an error occurs, users should be presented with clear and meaningful error messages, while developers should be equipped with the necessary tools to diagnose and fix the issue.
Understanding the Basics of Error Handling in Spring MVC
In a Spring MVC application, error handling can be handled at various levels, including:
- Controller level: handling exceptions specific to a particular controller.
- Global level: handling exceptions that are not caught at the controller level.
Common Pitfalls in Error Handling
Overly Generic Error Messages
When error messages are too generic, they can frustrate users and hinder effective troubleshooting. It's important to provide relevant details in error messages to guide users and help them understand the issue.
Lack of Logging
Insufficient logging can make it challenging to diagnose and troubleshoot errors. Comprehensive logging can provide valuable insights into the root causes of errors.
Troubleshooting Steps
Step 1: Review the Controller Exception Handling
Examine the exception handling within the controllers. Are the exceptions being caught and handled appropriately? It's important to ensure that each controller handles exceptions specific to its operations.
@Controller
public class SampleController {
@ExceptionHandler(CustomException.class)
public ResponseEntity<String> handleCustomException(CustomException ex) {
// Handle the exception and return a meaningful error response
}
}
In the above code snippet, the @ExceptionHandler
annotation is used to handle a specific custom exception. This ensures that the controller can provide a tailored error response for that particular exception.
Step 2: Check Global Exception Handling
Review the global exception handling configuration. Are there specific error mappings defined to handle exceptions not caught at the controller level?
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<String> handleGlobalException(Exception ex) {
// Log the exception and return a generic error response
}
}
In the code above, the @ControllerAdvice
annotation is used to define global exception handling for all controllers. The @ExceptionHandler
method handles generic exceptions that may not be caught at the controller level.
Step 3: Verify Logging Configuration
Ensure that the application has robust logging configuration. Logging frameworks like SLF4J and Logback can be used to capture detailed information about exceptions and application events.
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
By incorporating SLF4J and Logback, developers can log relevant details about exceptions, such as stack traces and contextual information, to facilitate effective troubleshooting.
Best Practices for Effective Error Handling
-
Use Custom Exception Classes: Define custom exception classes to represent specific error scenarios within the application. This allows for fine-grained control over error handling and messaging.
-
Provide Clear Error Messages: Ensure that error messages are clear, concise, and provide actionable information for users. This can help mitigate user frustration and improve the overall user experience.
-
Comprehensive Logging: Implement comprehensive logging to capture detailed information about exceptions, including stack traces, input parameters, and contextual details. This can aid developers in diagnosing and addressing errors effectively.
-
Monitor Error Rates: Implement monitoring and alerting for error rates to proactively identify and address recurrent issues. Tools like Prometheus and Grafana can be valuable for monitoring error rates in a Spring MVC application.
Bringing It All Together
Effective error handling is paramount for the success of a Spring MVC application. By following best practices, reviewing and refining error handling strategies, and ensuring comprehensive logging, developers can troubleshoot and address errors effectively, leading to a more robust and user-friendly application.
By implementing these best practices and following the troubleshooting steps outlined in this article, developers can enhance the error handling capabilities of their Spring MVC applications, thereby delivering a more reliable and seamless user experience.
For further information on handling exceptions in Spring MVC, refer to the official Spring documentation.
Remember, proactive error handling not only benefits users but also contributes to the overall stability and performance of the application.