Master Any Language: Overcoming Practicality Hurdles!
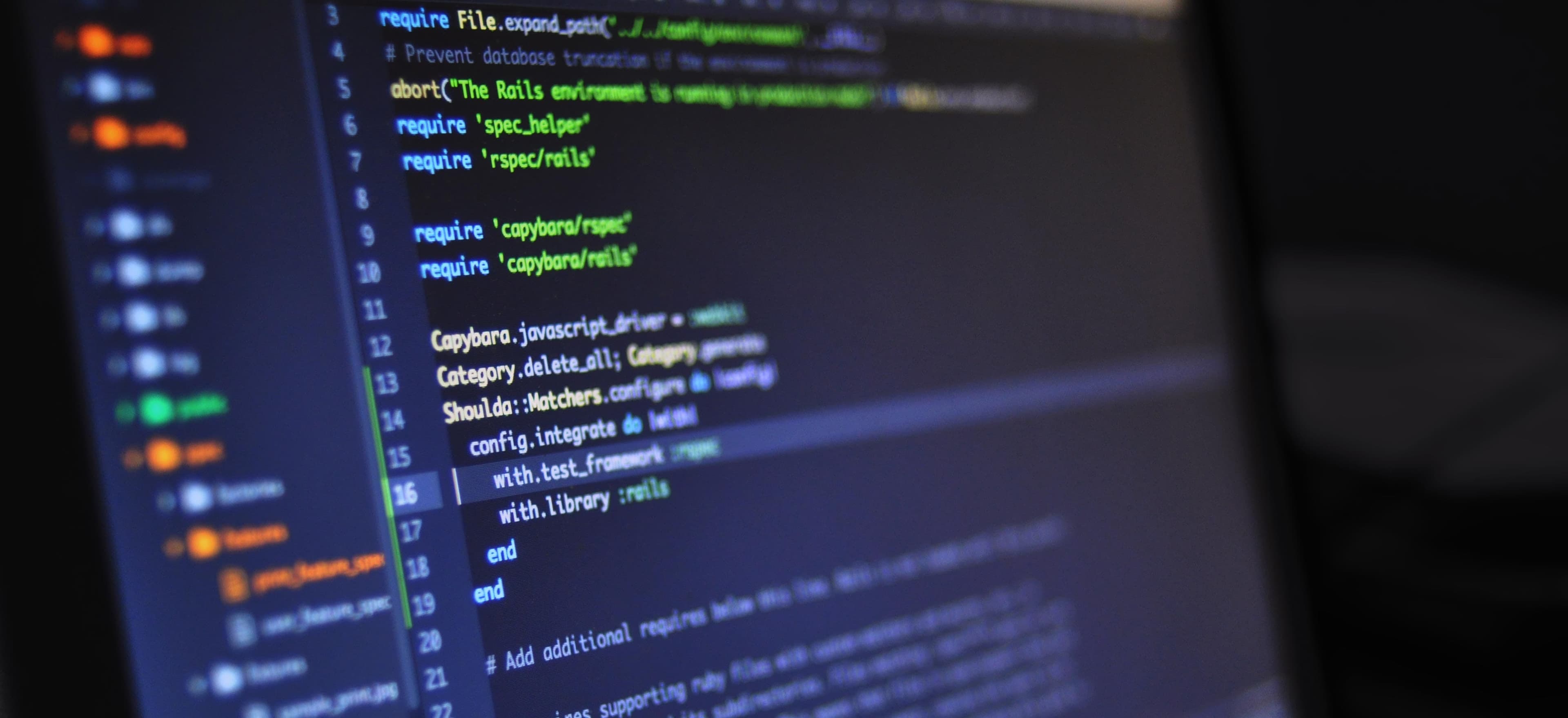
- Published on
Master Any Language: Overcoming Practicality Hurdles!
Establishing the Context
Programming languages are the building blocks of software development, and mastering a new language can significantly enhance a developer's skill set, broaden career opportunities, and improve adaptability to change. However, the journey toward mastering a new language often comes with practical challenges and hurdles, particularly when learning a versatile and widely used language like Java.
Understanding Java's Popularity and Challenges
The Popularity of Java
For decades, Java has been a popular choice among developers due to its versatility, portability, and strong community support. Its ability to run on diverse platforms, from desktops to mobile devices and large enterprise systems, has contributed to its widespread adoption. Additionally, the rich ecosystem of libraries and frameworks has made Java a go-to language for a wide range of application development.
Common Challenges When Learning Java
Despite its popularity, mastering Java comes with its own set of challenges. Beginners often face hurdles when grasping essential object-oriented programming concepts, handling memory management, and navigating Java's syntax and extensive libraries. Overcoming these challenges requires a structured approach and practical strategies.
Overcoming Practical Hurdles
Setting Up a Productive Development Environment
The first step in mastering Java is setting up a productive development environment. Integrated Development Environments (IDEs) like Eclipse and IntelliJ IDEA can streamline the development process. In IntelliJ IDEA, creating a new Java project can be done by following these steps:
Exemplary Code Snippet 1:
// Setting up a simple Java project in IntelliJ IDEA
1. Open IntelliJ IDEA and select "Create New Project"
2. Choose "Java" from the list of project types
3. Specify project name and location, then click "Finish"
4. Write your Java code in the source folder within the project
5. Run the code using the built-in run configurations
Grasping the Basics with Hands-On Practice
Once the environment is set up, it's essential to gain practical experience by writing and executing Java code. Starting with simple projects, like creating a "Hello World!" program or a basic calculator, can help in understanding Java's fundamental syntax and data types.
Exemplary Code Snippet 2:
// Writing a simple "Hello World!" program in Java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
In the above code, we define a class named HelloWorld
with a main
method, which is the entry point of the program. The println
statement is used to display the message "Hello, World!" to the console.
Understanding Object-Oriented Programming (OOP) in Java
Java is an object-oriented programming language, and understanding its key principles, such as inheritance, encapsulation, polymorphism, and abstraction, is crucial. Let's explore an example that demonstrates inheritance, one of the core principles of OOP.
Exemplary Code Snippet 3:
// Demonstrating inheritance in Java
// Base class
class Shape {
void draw() {
System.out.println("Drawing a shape");
}
}
// Derived class
class Circle extends Shape {
void draw() {
System.out.println("Drawing a circle");
}
}
public class Main {
public static void main(String[] args) {
Shape s = new Circle();
s.draw();
}
}
In the above example, we have a base class Shape
with a method draw
, and a derived class Circle
that inherits from the Shape
class. When the draw
method is invoked on an instance of Circle
, it overrides the method defined in the base class, demonstrating the concept of inheritance.
Utilizing Online Resources and Communities
Leveraging online resources and communities can provide invaluable support and knowledge sharing opportunities. Platforms like Stack Overflow, GitHub, and reputable Java forums offer a wealth of information, code snippets, and solutions to common challenges. Engaging with these communities can enhance the learning journey and provide insights into best practices from experienced Java developers.
Building Real-World Projects
Applying theoretical knowledge to real-world projects is a pivotal step in the learning process. Building practical applications, such as a simple e-commerce website or a basic data management system, can solidify understanding and provide hands-on experience with Java's features and capabilities.
Debugging and Problem-Solving Strategies
Debugging is a critical skill in software development. Learning to effectively debug Java programs using IDE tools and interpreting exception details can significantly improve problem-solving abilities. By practicing debugging techniques and analyzing errors, developers can gain insights into program behavior and identify and resolve issues efficiently.
Staying Motivated and Persistent
In the face of challenges, setting realistic goals, celebrating small victories, and maintaining persistence are key to staying motivated in the learning process. Learning a new language, especially one as versatile as Java, is a journey that requires determination and a growth mindset. Embracing the learning curve and continuously seeking knowledge is essential for long-term success.
Advanced Topics and Further Learning
As developers progress in their Java journey, they can explore advanced topics such as concurrency, multithreading, frameworks like Spring and Hibernate, and Java's role in Android app development. Resources like official documentation, online courses, and advanced Java programming books can offer comprehensive insights into these advanced concepts and further expand skills in Java development.
In Conclusion, Here is What Matters
In conclusion, mastering a new programming language like Java is a challenging yet rewarding endeavor. By embracing practical strategies such as hands-on practice, leveraging resources and communities, and building real-world projects, developers can overcome hurdles and elevate their Java skills. While the journey may present obstacles, persistence and continuous learning are the keys to mastering Java and thriving in the ever-evolving landscape of software development.
Call to Action
If you've been considering diving into Java development or are already on the learning path, take action today. Start a simple Java project using the tips and resources provided in this article. Share your progress, experiences, and any additional resources you find helpful in the comments below. Remember, every step taken in the journey of mastering Java brings you closer to realizing your full potential as a developer. Happy coding!