Mastering CXF Services: Handling Multiple Query Params
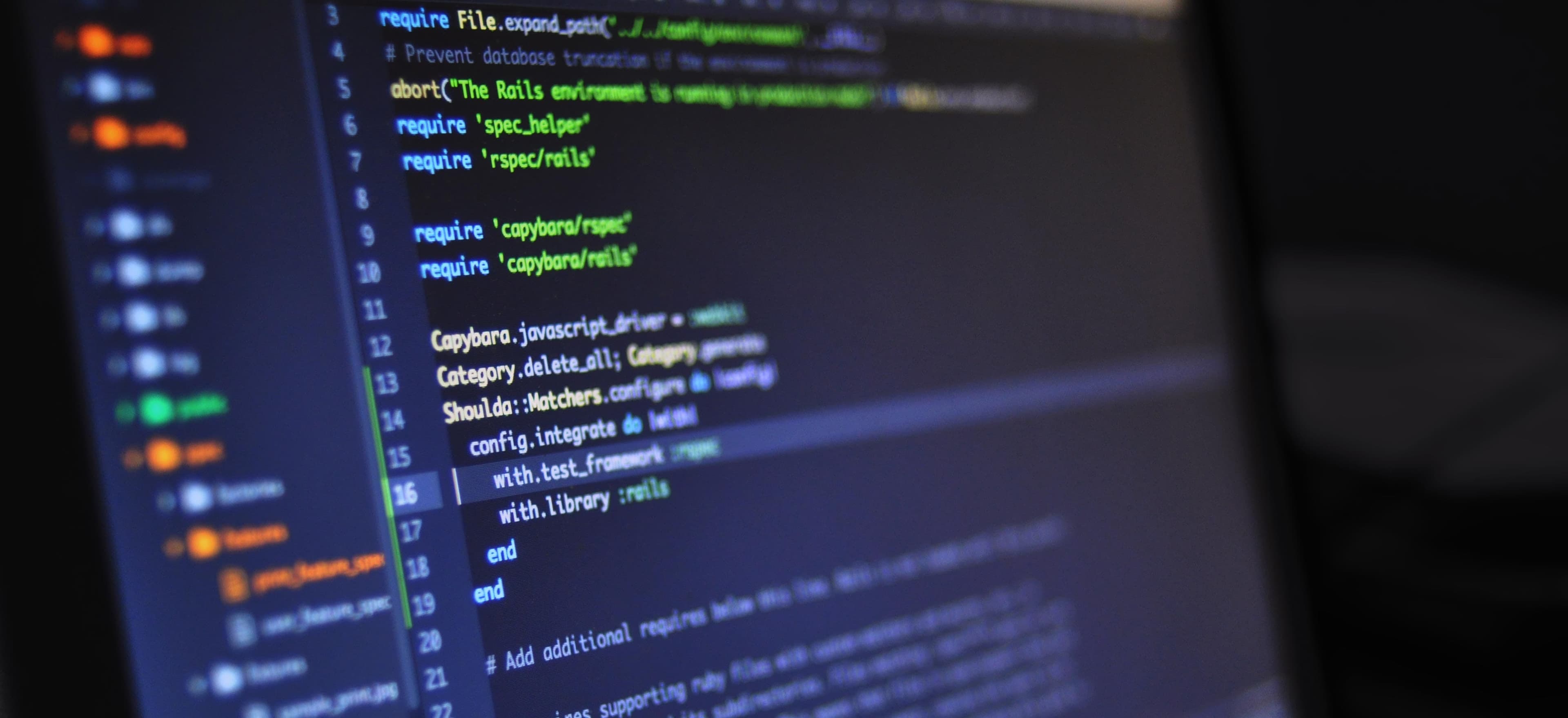
- Published on
Mastering CXF Services: Handling Multiple Query Params
When building robust and scalable web services using Java, Apache CXF is a powerful and widely-used framework. In this post, we'll delve into the key concepts of handling multiple query parameters in CXF services with practical examples and best practices.
Understanding Query Parameters
Query parameters are a fundamental part of RESTful web services. They provide a way to pass data to the server in the URL. In Apache CXF, handling multiple query parameters efficiently is essential for seamless communication between clients and services.
The Basics: Defining CXF Service
Let's start by creating a simple CXF service that handles multiple query parameters. First, we define the service interface, for example:
public interface BookService {
List<Book> searchBooks(String title, String author);
}
Here, the searchBooks
method takes two query parameters: title
and author
. Now, let's implement this service using CXF.
Implementing CXF Service
We can implement the BookService
interface as follows:
public class BookServiceImpl implements BookService {
@Override
public List<Book> searchBooks(String title, String author) {
// Implementation logic to search books
}
}
Handling Multiple Query Parameters
In CXF, handling multiple query parameters is straightforward. We can use JAX-RS annotations to extract query parameters from the incoming HTTP request. Here's how we can modify the service implementation to handle multiple query parameters:
import javax.ws.rs.QueryParam;
public class BookServiceImpl implements BookService {
@Override
public List<Book> searchBooks(@QueryParam("title") String title, @QueryParam("author") String author) {
// Implementation logic to search books
}
}
In this modified implementation, we use the @QueryParam
annotation to bind the query parameters to the method parameters.
Best Practices
While handling multiple query parameters in CXF services, it's important to adhere to best practices to ensure maintainability and readability of the codebase.
-
Use descriptive parameter names: When defining method parameters, use descriptive names that convey the purpose of each query parameter. This enhances the readability of the code and makes it easier for other developers to understand the intent of each parameter.
-
Validate query parameters: Always validate query parameters to ensure they meet the expected criteria. This helps prevent invalid data from being processed and enhances the overall robustness of the service.
Handling Optional Query Parameters
In some scenarios, query parameters may be optional. In CXF, we can handle optional query parameters by setting default values for method parameters. Here's an example:
public class BookServiceImpl implements BookService {
@Override
public List<Book> searchBooks(@QueryParam("title") String title, @QueryParam("author") String author, @DefaultValue("10") @QueryParam("limit") int limit) {
// Implementation logic to search books
}
}
In this example, the limit
query parameter is optional, and we've set a default value of 10 using the @DefaultValue
annotation. This ensures that the service can handle requests with or without the limit
parameter.
The Last Word
In this post, we've explored the essentials of handling multiple query parameters in Apache CXF services. We've covered the basics of defining and implementing a CXF service, as well as best practices for handling query parameters. By following these guidelines and leveraging the capabilities of CXF, you can build efficient and robust web services that cater to diverse client requirements.
For further insights into Apache CXF, you can refer to the official documentation.
Happy coding!