Why You Can't Skip Learning Functional Programming in Java 8
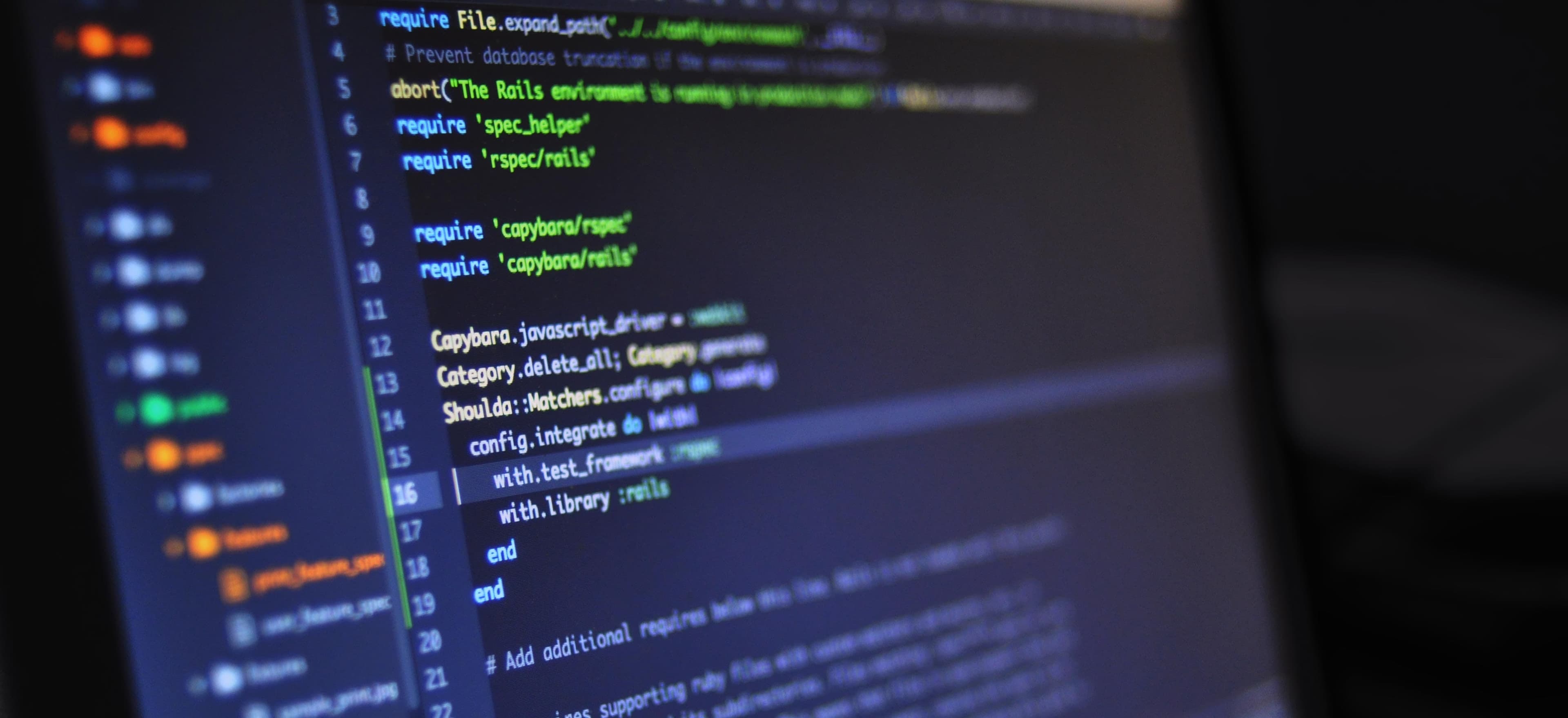
- Published on
Understanding the Significance of Functional Programming in Java 8
As a Java developer, you might have heard about functional programming and how it's been revolutionizing the way Java applications are developed. With the release of Java 8, functional programming capabilities were introduced, bringing a whole new paradigm to the language. In this article, we'll delve into why it is crucial for Java developers to embrace functional programming in Java 8, understand its significance, and explore its benefits.
Evolution of Functional Programming in Java
Java 8 marked a significant shift with the introduction of lambda expressions, functional interfaces, streams, and other functional programming features. These additions enable developers to write more concise, readable, and efficient code by incorporating functional programming concepts into their Java applications.
Lambda Expressions
Lambda expressions facilitate functional programming by allowing developers to write inline, concise implementations of functional interfaces. They provide a shorthand for implementing single-method interfaces, making the code more expressive and less verbose.
// Example of a Lambda Expression
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
names.forEach(name -> System.out.println("Hello, " + name));
The above code uses a lambda expression to iterate through the list of names and print a greeting for each name.
Streams
Streams enable developers to perform sequential or parallel operations on a collection of data. They provide a declarative approach to process data, allowing for more functional-style transformations, filtering, and aggregations.
// Example of Streams
List<String> languages = Arrays.asList("Java", "Python", "JavaScript", "Ruby", "Kotlin");
long count = languages.stream()
.filter(lang -> lang.length() > 4)
.count();
System.out.println("Number of languages with more than 4 characters: " + count);
In this example, a stream is used to filter the languages with more than 4 characters and count the remaining ones.
Embracing Functional Programming Principles
Functional programming brings about a shift in the way developers approach problem-solving and code design. By embracing functional programming principles, Java developers can leverage the following benefits:
Immutability
Functional programming promotes immutability, where once a variable is assigned a value, it cannot be changed. Immutability reduces complexity, avoids unintended side effects, and facilitates better concurrency control.
Higher-Order Functions
In functional programming, functions are treated as first-class citizens, meaning they can be passed as parameters to other functions, returned as values, and stored in data structures. This enables the use of higher-order functions, allowing for more flexible and modular code.
Avoiding Side Effects
Functional programming encourages writing pure functions that do not produce side effects. This leads to more predictable code and simplifies debugging and testing.
Parallelism
With the introduction of streams and parallel processing in Java 8, functional programming facilitates parallelism, enabling efficient utilization of multi-core processors for improved performance.
Leveraging Functional Interfaces
Functional interfaces play a pivotal role in Java's embrace of functional programming. A functional interface is an interface that contains only one abstract method, and it can be annotated with @FunctionalInterface
for compiler checks. By using these interfaces, Java developers can achieve better code organization and maintainability.
// Example of a Functional Interface
@FunctionalInterface
interface Calculator {
int calculate(int a, int b);
}
The Calculator
interface is a functional interface with a single abstract method, calculate
. It can be utilized for lambda expressions and method references.
Gaining Performance Benefits
Functional programming in Java 8 not only enhances code readability and maintainability but also brings performance benefits through efficient resource utilization and parallel processing capabilities. By leveraging streams and parallel streams, developers can significantly improve the performance of their applications.
The Road Ahead
As Java evolves, functional programming continues to play a crucial role in shaping the way developers write modern, efficient, and maintainable code. With the introduction of records, pattern matching, and other enhancements in Java, the functional programming paradigm is further solidifying its position in the Java ecosystem.
In conclusion, functional programming in Java 8 is not just a trend but a fundamental shift in the way Java developers approach software development. Embracing functional programming principles, leveraging lambda expressions, streams, and functional interfaces, and reaping the associated benefits are essential for staying competitive in the ever-changing world of Java development.
By understanding and mastering functional programming in Java 8, developers can write cleaner, more robust, and more efficient code that aligns with modern software development practices.
Are you ready to dive deeper into functional programming in Java 8 and explore its full potential?