Emulating Kent Beck: Solving Complex Code with Simplicity
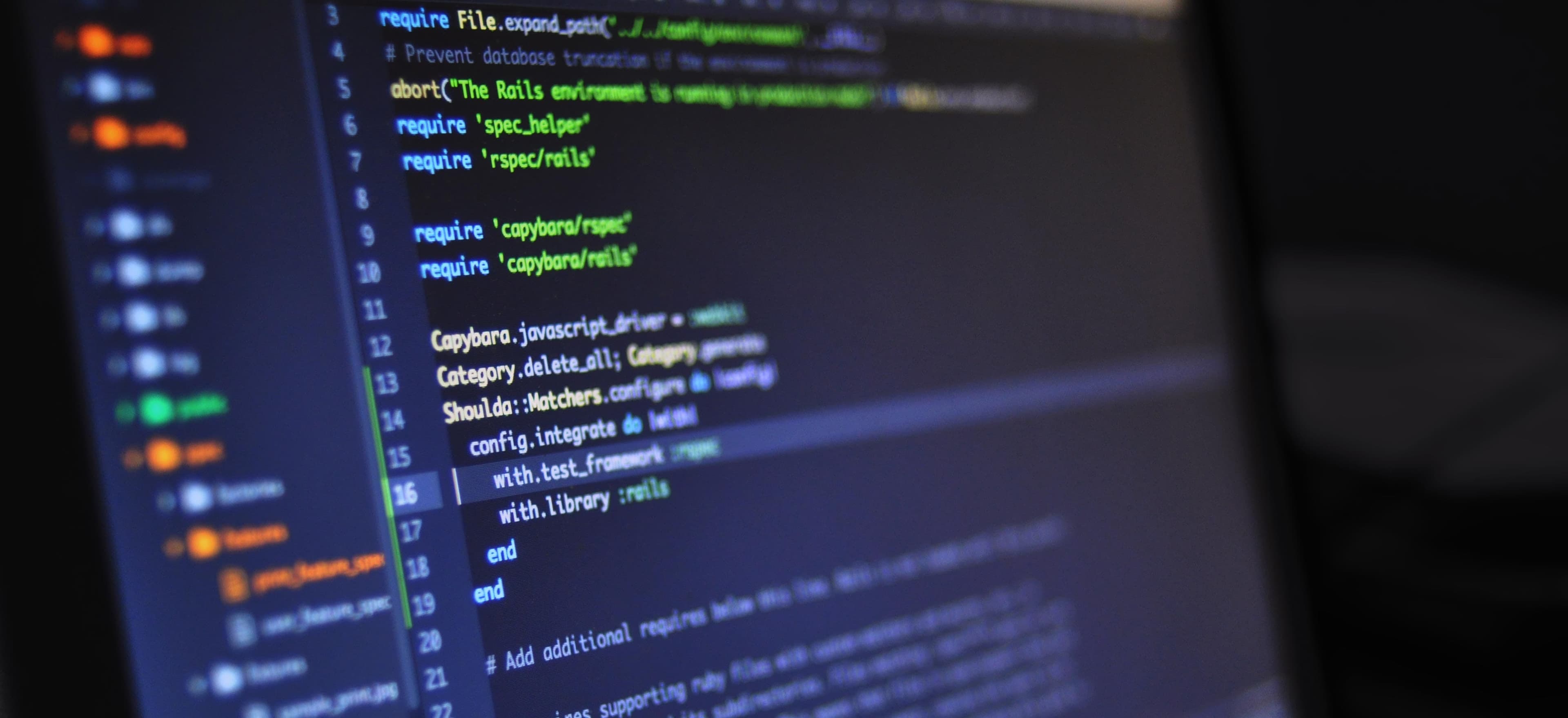
- Published on
Emulating Kent Beck: Solving Complex Java Code with Simplicity
Kent Beck, the creator of Extreme Programming (XP) and a significant contributor to agile software development, advocates for the simplicity over complexity principle. In the context of Java programming, simplicity not only enhances the maintainability and readability of the code but also contributes to improved performance. This article delves into the concept of simplicity in Java programming, and how to solve complex problems using simple, elegant solutions.
The Simplicity Principle in Java
Simplicity in Java programming is about writing clean, understandable, and concise code that achieves its purpose while being easy to maintain and extend. When dealing with complex problems, it's tempting to craft elaborate, intricate solutions. However, embracing simplicity often leads to more robust and effective code. Kent Beck's approach to simplicity involves favoring the straightforward, minimalistic, and direct way of implementing functionality.
Using Simple Data Structures
In Java, using simple data structures is a fundamental way to promote simplicity. It's common to encounter situations where complex data structures are used when simpler ones would suffice. Let's consider an example of using a HashMap
to store key-value pairs.
Instead of:
HashMap<String, String> employeeDetails = new HashMap<>();
employeeDetails.put("John", "Developer");
employeeDetails.put("Emily", "Designer");
Use a Map
interface for improved flexibility:
Map<String, String> employeeDetails = new HashMap<>();
employeeDetails.put("John", "Developer");
employeeDetails.put("Emily", "Designer");
Using the Map
interface promotes simplicity as it allows for easy switching between different implementations such as HashMap
, TreeMap
, or LinkedHashMap
without affecting the code that uses this interface. It emphasizes the "program to an interface, not an implementation" principle, which is central to the simplicity paradigm.
Leveraging Lambda Expressions
Java 8 introduced lambda expressions, a feature that enables functional programming constructs. Lambda expressions promote simplicity by allowing developers to write more concise and readable code, especially when working with collections.
Consider the following example, where a list of employee names needs to be printed:
List<String> employees = Arrays.asList("John", "Emily", "Mike");
for (String employee : employees) {
System.out.println(employee);
}
Using lambda expressions, the code can be simplified as follows:
List<String> employees = Arrays.asList("John", "Emily", "Mike");
employees.forEach(System.out::println);
The use of lambda expressions reduces the ceremonial and boilerplate code, leading to more elegant and succinct solutions.
Solving Complex Problems with Simple Algorithms
When faced with complex problems, it's crucial to rely on simple algorithms and data structures before considering intricate solutions. For instance, when searching for an element in a collection, opting for the straightforward linear search reflects simplicity:
public static <T> int linearSearch(T[] array, T key) {
for (int i = 0; i < array.length; i++) {
if (array[i].equals(key)) {
return i;
}
}
return -1;
}
While more sophisticated search algorithms like binary search exist, choosing the appropriate algorithm based on the problem's requirements and complexity aligns with the simplicity principle.
Embracing Test-Driven Development (TDD)
Kent Beck is a fervent advocate of Test-Driven Development (TDD). TDD promotes simplicity by encouraging developers to focus on writing the simplest possible code to pass the failing test. Writing tests before the actual code promotes clear and concise design, as the code is tailored to satisfy the test cases.
Here's an example of a simple test case using JUnit:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class SimpleCalculatorTest {
@Test
public void testAddition() {
SimpleCalculator calculator = new SimpleCalculator();
assertEquals(5, calculator.add(2, 3));
}
}
By writing tests first, developers are compelled to keep the design simple and only implement what is necessary to fulfill the test scenarios. This approach promotes clean, minimalistic, and robust code.
Bringing It All Together
In the world of Java programming, simplicity is a guiding principle that can lead to more maintainable, readable, and efficient code. By employing simple data structures, leveraging lambda expressions, opting for straightforward algorithms, and embracing TDD, developers can solve complex problems with elegant, uncomplicated solutions. Kent Beck's philosophy of simplicity continues to inspire developers to approach code with minimalism and clarity, finding beauty in simplicity and elegance.
Emulating Kent Beck's approach to simplicity can lead to more robust and maintainable code, ultimately benefiting both developers and end-users.
Remember, simplicity isn't about writing less code, it's about solving complex problems with clear, concise, and elegant solutions. Happy coding!
Considering the significance of Kent Beck's influence, this article is tailored to serve as a resource for Java developers aiming to harness the power of simplicity in their code. The emphasis on leveraging simple data structures, lambda expressions, and TDD aligns with contemporary best practices in Java programming. By incorporating these principles, developers can produce elegant, efficient, and maintainable code.