Boost Speed: Mastering Performance Optimization's #1 Rule!
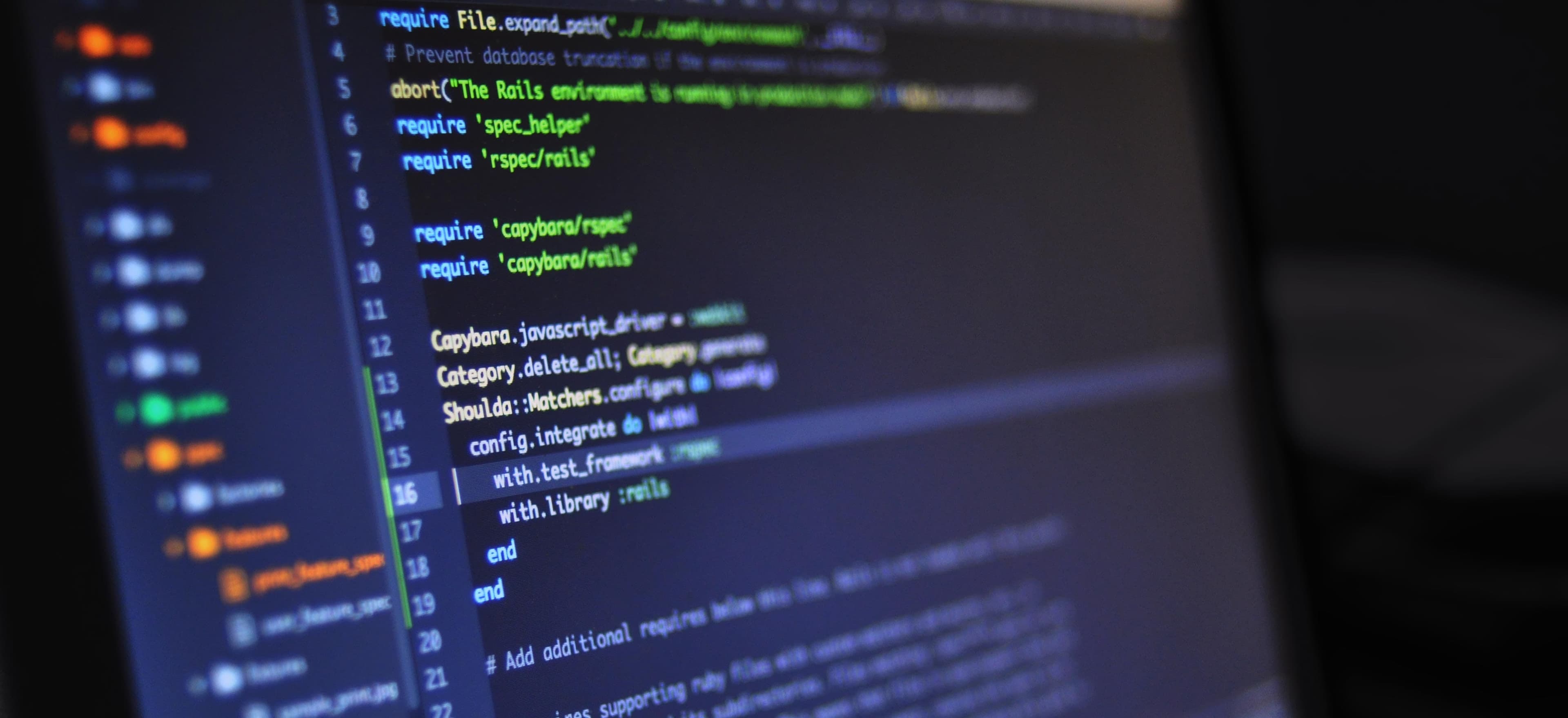
- Published on
The Ultimate Guide to Performance Optimization in Java
Performance optimization is a crucial aspect of Java development. Whether you're working on a small project or a large-scale application, optimizing your code can make a significant difference in terms of speed, efficiency, and resource utilization. In this comprehensive guide, we'll delve into the best practices for optimizing the performance of your Java applications.
Understanding the Importance of Performance Optimization
Performance optimization is not merely about making your code run faster; it's about maximizing the efficiency of your application. When your code is optimized, it consumes fewer resources, responds more quickly, and provides a better user experience. In today's highly competitive digital landscape, where user expectations are continually increasing, optimizing the performance of your Java applications is non-negotiable.
The #1 Rule of Performance Optimization: Measure, Don't Guess!
Before diving into the intricate techniques and tools for performance optimization, it's essential to understand the cardinal rule: Measure, Don't Guess! Without accurate measurements, you won't be able to identify performance bottlenecks or validate the effectiveness of your optimizations.
So, how do you measure the performance of your Java application? The answer lies in utilizing profiling tools. Profilers such as VisualVM and YourKit enable you to analyze various aspects of your application's performance, including CPU and memory usage, method hotspots, and object allocation. By identifying areas of code that consume the most resources, you can focus your optimization efforts where they'll have the most impact.
Optimizing Java Code for Maximum Performance
1. Efficient Data Structures and Algorithms
The choice of data structures and algorithms can have a significant impact on the performance of your Java application. For instance, using a HashMap instead of an ArrayList for large datasets can dramatically improve lookup and insertion times. Similarly, employing efficient sorting algorithms like QuickSort or MergeSort can enhance the performance of sorting operations.
Let's take a look at an example of how choosing the right data structure can improve performance:
// Inefficient approach using ArrayList
List<String> names = new ArrayList<>();
names.add("Alice");
names.add("Bob");
names.add("Charlie");
// Efficient approach using HashMap for quick lookup
Map<String, Integer> ages = new HashMap<>();
ages.put("Alice", 30);
ages.put("Bob", 28);
ages.put("Charlie", 35);
In this example, using a HashMap for storing name-age pairs allows for constant-time lookup, resulting in improved performance compared to an ArrayList.
2. String Concatenation and StringBuilder
In Java, string concatenation using the + operator can be inefficient, especially when dealing with a large number of concatenations. The String class in Java is immutable, meaning that every concatenation operation creates a new string object. To address this, the StringBuilder class should be used for intensive string manipulations to minimize the creation of additional string objects.
Consider the following example:
String result = "";
for (int i = 0; i < 1000; i++) {
result += "hello";
}
In the above code, using + for string concatenation within the loop will create a new string object in each iteration, leading to poor performance. Let's refactor it to use StringBuilder:
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 1000; i++) {
sb.append("hello");
}
String result = sb.toString();
By using StringBuilder, we eliminate the creation of unnecessary string objects, resulting in a more efficient and performant solution.
3. Proper Usage of Java Collections
When working with Java collections, it's essential to understand the proper usage of interfaces such as List, Set, and Map, along with their respective implementations (ArrayList, LinkedList, HashSet, TreeMap, etc.). Selecting the appropriate collection type based on the specific requirements of your application can significantly impact performance.
Let's consider an example where choosing the right collection type can yield performance benefits:
// Inefficient iteration using ArrayList
List<Integer> numbers = new ArrayList<>();
numbers.add(1);
numbers.add(2);
numbers.add(3);
for (Integer number : numbers) {
System.out.println(number);
}
// Efficient iteration using HashSet for better performance
Set<Integer> uniqueNumbers = new HashSet<>();
uniqueNumbers.add(1);
uniqueNumbers.add(2);
uniqueNumbers.add(3);
for (Integer number : uniqueNumbers) {
System.out.println(number);
}
In this example, using a HashSet removes the need for maintaining an ordered collection, resulting in faster iteration, especially when dealing with a large number of elements.
Leveraging Multithreading for Performance Gains
Java's support for multithreading enables developers to take advantage of parallelism, thereby improving overall application performance. By efficiently utilizing multiple threads, tasks can be executed concurrently, leading to enhanced throughput and responsiveness.
However, it's crucial to approach multithreading with caution, as improper handling can introduce synchronization issues and degrade performance. The java.util.concurrent package provides a rich set of classes and interfaces for building concurrent and multithreaded applications, including thread pools, concurrent collections, and synchronization utilities.
Let's consider an example of leveraging multithreading to improve performance:
// Creating a thread pool to perform concurrent tasks
ExecutorService threadPool = Executors.newFixedThreadPool(4);
List<Future<String>> results = new ArrayList<>();
for (int i = 0; i < 10; i++) {
Future<String> result = threadPool.submit(() -> {
// Perform a computationally intensive task
return "Task completed";
});
results.add(result);
}
// Processing the results once the tasks are completed
for (Future<String> result : results) {
String taskResult = result.get();
System.out.println(taskResult);
}
// Shutting down the thread pool
threadPool.shutdown();
In this example, a thread pool is created to execute multiple tasks concurrently, leveraging the available resources for improved performance.
Utilizing Just-In-Time (JIT) Compilation for Runtime Optimization
Java's Just-In-Time (JIT) compilation plays a vital role in optimizing the performance of Java applications at runtime. JIT compilation translates Java bytecode into native machine code, allowing for efficient execution of frequently accessed methods.
By default, Java HotSpot, one of the most widely used Java Virtual Machine implementations, employs sophisticated JIT compilation techniques to dynamically optimize and recompile hotspots in the code. Additionally, modern JVMs provide various options to tune the behavior of the JIT compiler based on the specific workload and performance requirements of the application.
A Final Look
In conclusion, performance optimization is an indispensable aspect of Java development, and mastering it is paramount to delivering high-performing and efficient applications. By adopting best practices, leveraging the right tools, and understanding the nuances of performance tuning, developers can unlock the full potential of their Java applications.
Remember, the #1 rule of performance optimization remains: Measure, Don't Guess! Continuously monitor and profile your application to identify performance bottlenecks and validate the impact of your optimizations.
Optimizing the performance of your Java applications is an ongoing process, and staying abreast of the latest developments in Java performance tuning is essential for delivering exceptional user experiences and maintaining a competitive edge in the ever-evolving landscape of software development.
So, go ahead, apply these optimization techniques, and embark on the journey to supercharge the performance of your Java applications!
Remember, as much as Java performance optimization is an art, it's also a science. Delve into the intricacies, measure meticulously, and watch your Java applications soar to new performance heights!
Checkout our other articles