Unwrapping Scala's Cake Pattern: A Slice of Pros & Cons
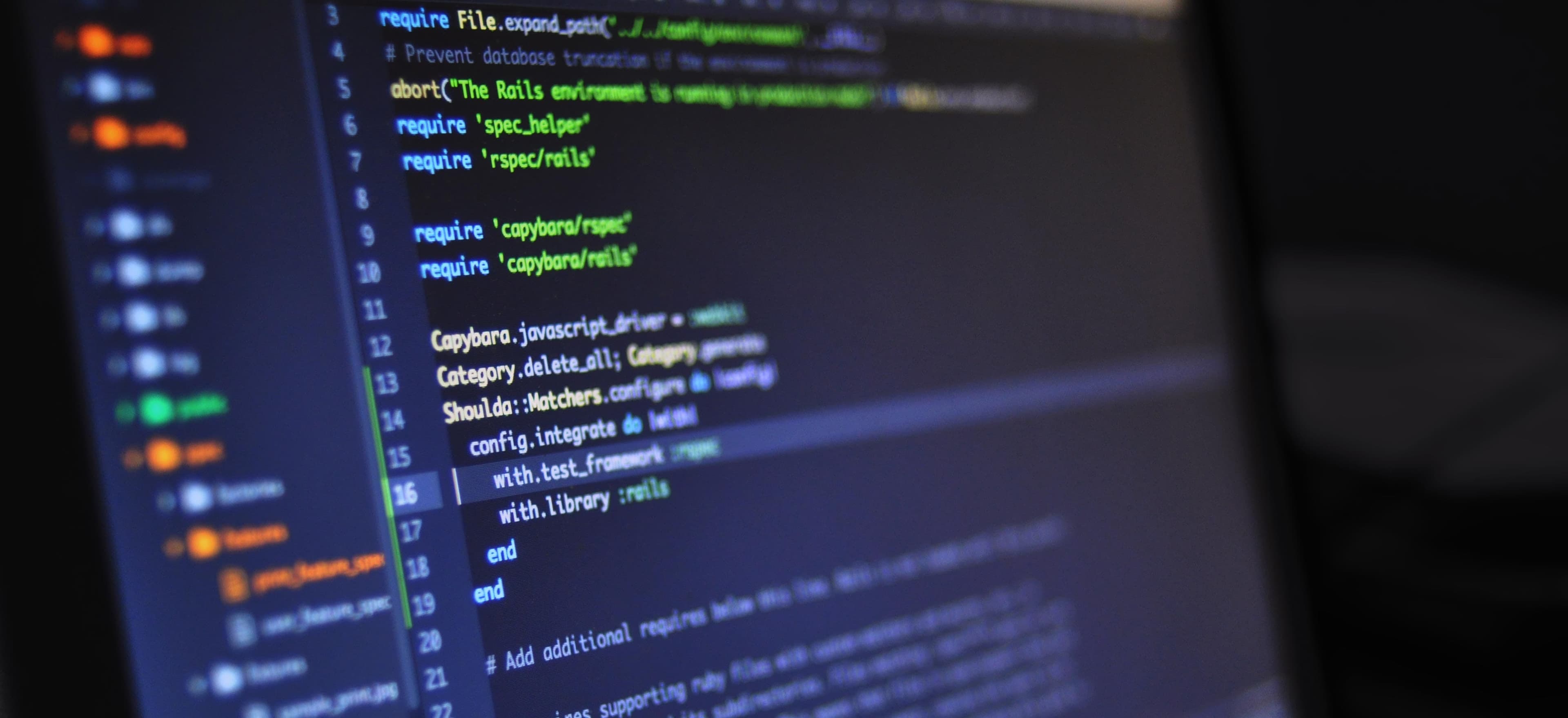
- Published on
Unwrapping Scala's Cake Pattern: A Slice of Pros & Cons
Scala, a powerful and expressive programming language, seamlessly blends object-oriented and functional programming paradigms. This fusion enables developers to create robust, scalable, and maintainable applications. One design pattern that stands out in the Scala ecosystem is the Cake Pattern, which provides an elegant solution to common problems related to dependency management and modularity. In this blog post, we will explore the advantages and disadvantages of the Cake Pattern in the realm of Scala development.
What is the Cake Pattern?
The Cake Pattern, often associated with Scala, serves as a solution to the challenges of dependency injection and service layer abstraction in large applications. By utilizing Scala's traits and mixin composition, the Cake Pattern promotes the creation of loosely coupled and easily testable components. This approach results in a highly modular and flexible codebase, allowing for scalable and maintainable applications.
Why Use the Cake Pattern?
Given the nature of the Cake Pattern, its usage in Scala development brings several notable benefits:
-
Enhanced Modularity: The Cake Pattern encourages the division of application components into distinct traits, fostering a modular and organized codebase. This modularity facilitates easier maintenance and facilitates the extension of the application's functionality.
-
Improved Testability: The Cake Pattern simplifies the process of writing unit tests by employing dependency injection. It allows for easy mock implementation of components, thereby enhancing the testability of the application.
-
Flexible Configuration: Developers can easily switch between different implementations of components through the Cake Pattern, allowing for more flexible application configuration and development.
In the following sections, we will delve into a practical example to illustrate the implementation of the Cake Pattern in Scala, uncover its inner workings, and assess its pros and cons in detail.
The Cake Pattern in Action: A Practical Example
Scenario Description
Imagine a scenario where we are developing an application that manages user information. This application requires services like UserRepository
and EmailService
for the storage and communication of user data, and we will use the Cake Pattern to manage these dependencies.
Code Implementation
Let's take a look at how the Cake Pattern can be implemented in the context of the User Service Application scenario.
// Define the UserServiceComponent trait
trait UserServiceComponent {
val userRepository: UserRepository
// Define the UserRepository trait
trait UserRepository {
def getUserById(id: Int): Option[User]
def addUser(user: User): Boolean
// Other repository operations
}
}
// Define the EmailServiceComponent trait
trait EmailServiceComponent {
val emailService: EmailService
// Define the EmailService trait
trait EmailService {
def sendEmail(to: String, subject: String, body: String): Boolean
// Other email service operations
}
}
// Compose the application module by mixing in the required components
trait ApplicationComponent
extends UserServiceComponent
with EmailServiceComponent
{
val userRepository: UserRepository
val emailService: EmailService
}
In this example, we have defined traits for UserService
, UserRepository
, EmailService
, and composed them using the ApplicationComponent
trait.
Deep Dive: Understanding the Implementation
The aforementioned code snippet illustrates the core components of the Cake Pattern implementation.
The UserServiceComponent
and EmailServiceComponent
traits represent the service abstractions, each defining the required dependencies for their respective services. These traits encapsulate the dependencies within their self-type annotations, fostering the inversion of control and allowing for flexible integration of components.
The ApplicationComponent
trait combines the individual service components via mixin composition. This results in a fully integrated application module with access to all the required services. Self-type annotations facilitate the declaration of dependencies, allowing components to reference each other seamlessly while preserving loose coupling.
Weighing the Cake: Pros & Cons
Pros
The Cake Pattern in Scala brings forth several advantages for application development:
-
Modularity and Testability: The pattern's emphasis on trait-based componentization enhances the modularity and testability of the application. By promoting the encapsulation of dependencies within traits, the Cake Pattern facilitates easier management and testing of individual components.
-
Flexibility in Configuration: Through the use of self-type annotations and trait composition, the Cake Pattern allows for the flexible configuration of the application. This enables developers to seamlessly replace or upgrade components without necessitating major overhauls within the codebase.
Cons
Despite its benefits, the Cake Pattern also comes with certain considerations and potential downsides:
-
Complexity: The Cake Pattern, especially for newcomers to Scala or those unfamiliar with the pattern, can introduce complexity into the codebase. Understanding and effectively utilizing the pattern's features may require a learning curve for developers.
-
Boilerplate Code: Implementing the Cake Pattern might necessitate additional boilerplate code, especially when managing a large number of interconnected components. This can lead to increased verbosity and code maintenance overhead.
-
Compile-Time Dependencies: The pattern might obfuscate compile-time dependencies within the code, potentially complicating debugging sessions and making it more challenging to track down issues related to dependency resolution.
In Conclusion, Here is What Matters
In conclusion, the Cake Pattern in Scala presents a powerful and elegant solution for managing dependencies and promoting modularity. Its emphasis on trait-based composition and self-type annotations enables developers to create highly testable, modular, and flexible applications. However, it is essential to consider the potential complexity and verbosity that the pattern introduces, especially in larger codebases. Developers should carefully assess the specific needs and complexity of their projects before adopting the Cake Pattern.
As with any design pattern, a thorough understanding of its intricacies and trade-offs is crucial for making informed architectural decisions. Scala's vibrant and supportive community, combined with detailed language documentation, provides a wealth of resources for diving deeper into the Cake Pattern and other dependency management techniques.
Further Reading
For further exploration of the Cake Pattern and related topics in Scala development, consider the following resources:
- Scala Documentation: The official Scala documentation offers comprehensive insights into the language's features, including detailed explanations of the Cake Pattern.
- Scala Community Blogs: Various Scala community blogs and forums often host in-depth discussions, practical examples, and best practices related to the Cake Pattern and other design patterns.
- Academic Papers on Software Design Patterns: Delve into academic research on software design patterns, where you can find detailed analyses and comparative studies of different dependency injection techniques in Scala.
With a firm grasp of the advantages and potential challenges of the Cake Pattern, developers can make informed decisions when designing Scala applications and harness the power of this elegant design pattern.
Checkout our other articles