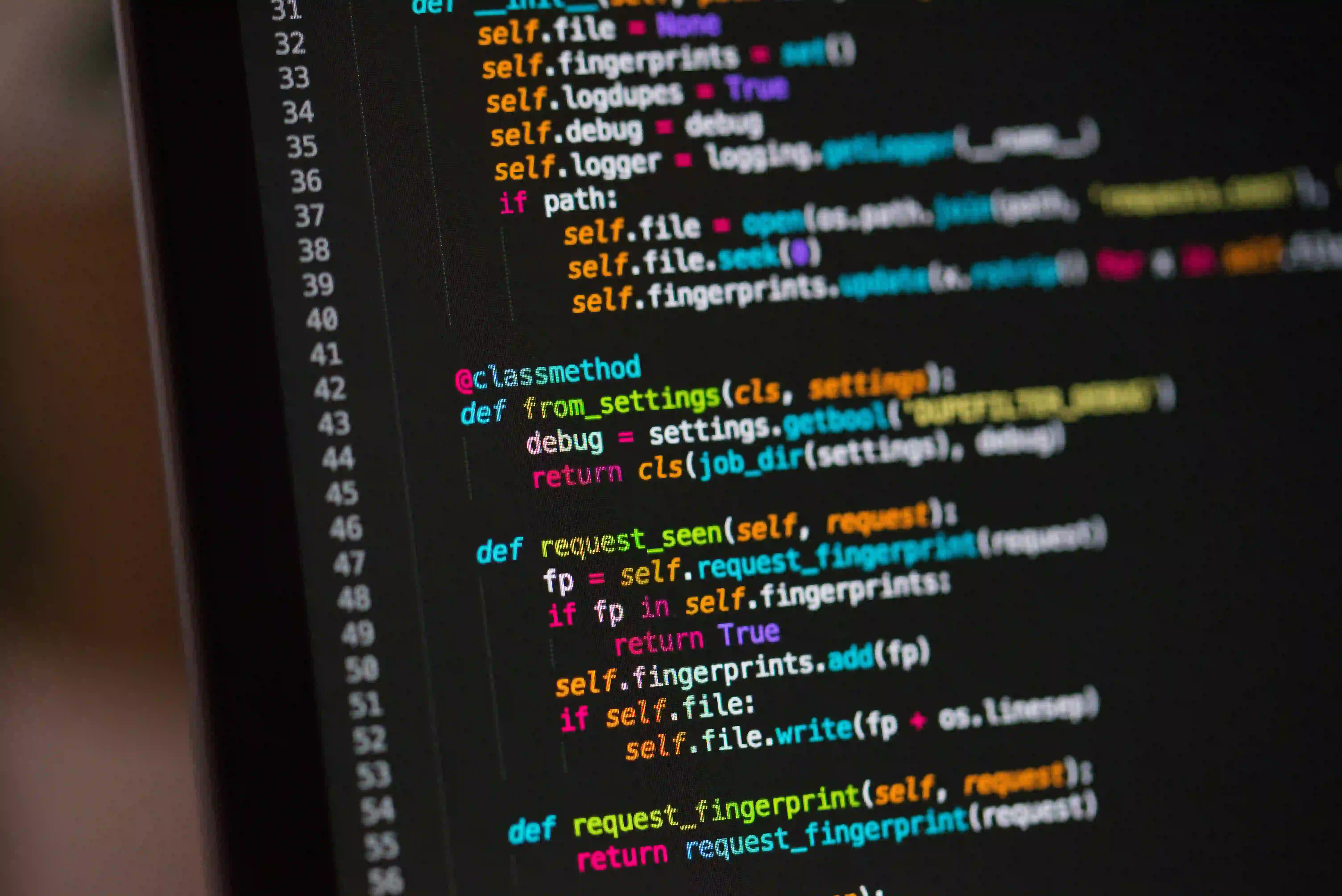
Mastering File Uploads & Downloads in Java Servlets
Handling file uploads and downloads is a crucial aspect of web application development. In this post, we will explore how to effectively manage file uploads and downloads in Java Servlets. We will cover everything from handling multipart file uploads to serving files for download, all while maintaining best practices for security and performance.
Uploading Files with Java Servlets
Handling File Upload Form
When working with file uploads in Java Servlets, you often deal with multipart form data. The HttpServletRequest
object provides APIs for parsing multipart request bodies. You can use a library like Apache Commons FileUpload for a more convenient approach.
// Handling file upload in doPost method of a Servlet
protected void doPost(HttpServletRequest request, HttpServletResponse response) {
boolean isMultipart = ServletFileUpload.isMultipartContent(request);
if (isMultipart) {
// Create a FileItemFactory
FileItemFactory factory = new DiskFileItemFactory();
// Create a new file upload handler
ServletFileUpload upload = new ServletFileUpload(factory);
try {
List<FileItem> items = upload.parseRequest(request);
for (FileItem item : items) {
if (!item.isFormField()) {
// Process file content here
String fileName = FilenameUtils.getName(item.getName());
// Save file to disk or perform other operations
}
}
} catch (FileUploadException e) {
// Handle file upload exception
}
}
}
Validating File Uploads
Validation is crucial for file uploads to ensure that the uploaded files meet the required criteria, such as file type, size, and content. You can perform validation before processing the uploaded file.
// Example file type validation
if (!item.getContentType().equals("image/jpeg") && !item.getContentType().equals("image/png")) {
// Handle invalid file type
}
Downloading Files with Java Servlets
Serving Files for Download
To serve files for download in a Java Servlet, you can set the appropriate HTTP headers and use the ServletOutputStream
to write the file content to the response.
// Setting headers for file download
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=\"example.txt\"");
// Writing file content to response
ServletOutputStream outputStream = response.getOutputStream();
File file = new File("/path/to/example.txt");
FileInputStream fileInputStream = new FileInputStream(file);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
fileInputStream.close();
outputStream.close();
Handling Large File Downloads
When dealing with large file downloads, it is important to consider streaming the file content instead of loading the entire file into memory. This approach can significantly improve performance and reduce memory consumption.
// Streaming large file for download
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=\"largefile.zip\"");
File file = new File("/path/to/largefile.zip");
FileInputStream fileInputStream = new FileInputStream(file);
ServletOutputStream outputStream = response.getOutputStream();
byte[] buffer = new byte[8192];
int bytesRead;
while ((bytesRead = fileInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
fileInputStream.close();
outputStream.close();
Security Considerations
Preventing File Upload Vulnerabilities
When handling file uploads, it is crucial to implement security measures to prevent common vulnerabilities such as directory traversal, executable file uploads, and content type spoofing.
Use file extension validation, content type validation, and store uploaded files outside the web root directory to mitigate these security risks.
Avoiding Path Traversal Attacks
Path traversal attacks can occur if proper precautions are not taken. Always sanitize and validate file paths to ensure that uploaded files are stored in the intended directory.
// Sanitize and validate file paths
String uploadDirectory = "/path/to/uploads/";
String sanitizedFileName = FilenameUtils.getName(item.getName());
File uploadedFile = new File(uploadDirectory + sanitizedFileName);
The Bottom Line
Mastering file uploads and downloads in Java Servlets is essential for building robust web applications. By understanding the intricacies of handling multipart form data, validating uploads, and serving files for download, you can ensure secure and efficient file management in your Java web applications.
By implementing best practices for security and performance, you can mitigate common vulnerabilities and provide a seamless file management experience for your users.
With the knowledge gained from this post, you are well-equipped to tackle the challenges of handling file uploads and downloads in Java Servlets with confidence and proficiency.
Whether you are developing a content management system, a file-sharing platform, or any web application that involves file manipulation, mastering these techniques will elevate the quality and reliability of your software.
For further reading, you can explore the official Java Servlet API documentation to delve deeper into the intricacies of file uploads and downloads in the context of web development.
Here's where you can find help: