Boost Your App Speed: Micro-Caching & Memoization Magic!
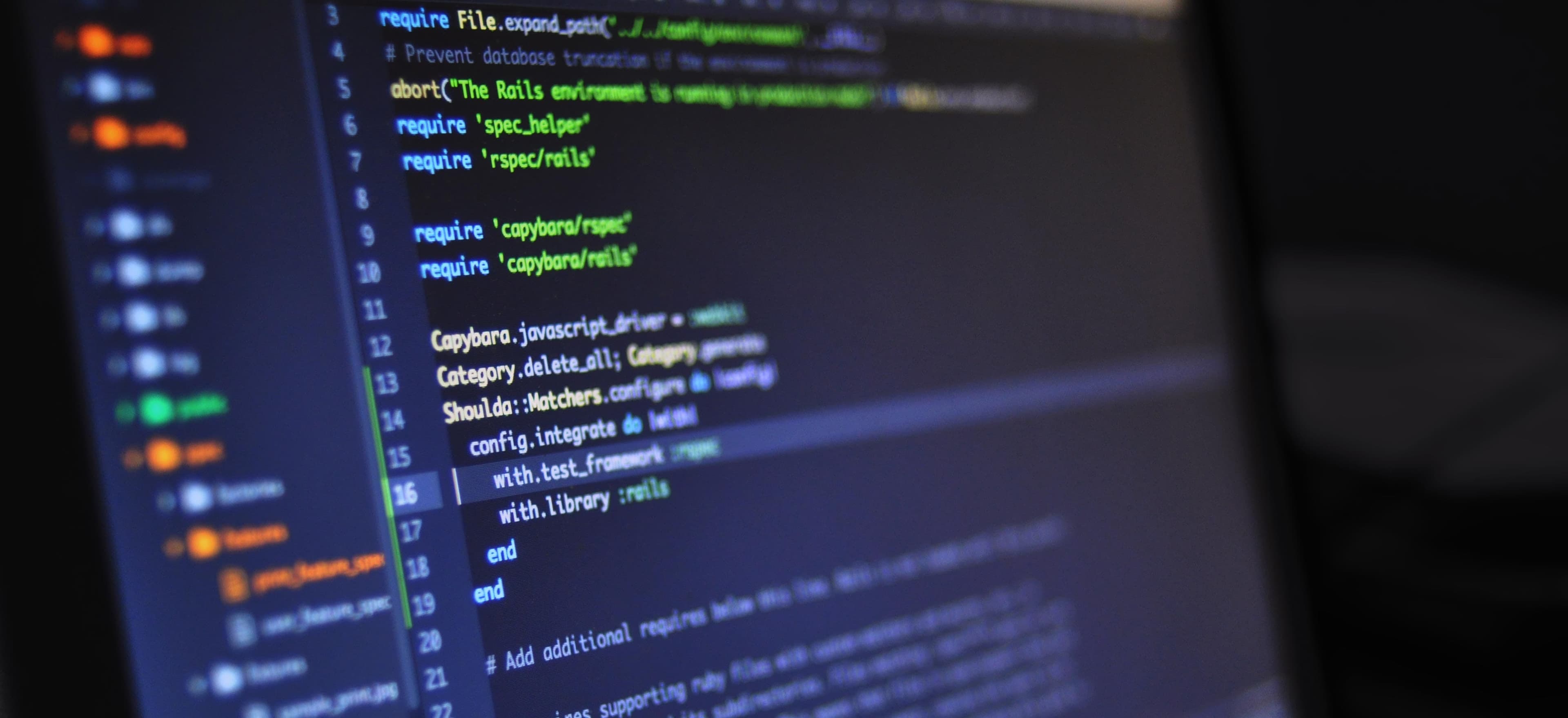
- Published on
Boost Your App Speed: Micro-Caching & Memoization Magic!
As a Java developer, you're constantly striving for better performance in your applications. One technique that can significantly boost the speed of your app is micro-caching and memoization. In this article, we'll delve into these concepts and see how they can work their magic to optimize your Java applications.
What is Micro-Caching?
Micro-caching involves caching the result of an expensive operation for a very short period of time. While traditional caching might hold data for minutes or hours, micro-caching involves caching data for just a few milliseconds. This can be exceptionally beneficial when dealing with operations that have high computational costs or are resource-intensive.
Implementing Micro-Caching in Java
Let's take a look at how micro-caching can be implemented in Java using the ConcurrentHashMap
class.
import java.util.concurrent.*;
public class MicroCache {
private ConcurrentHashMap<String, String> cache = new ConcurrentHashMap<>();
public String getResult(String input) {
return cache.computeIfAbsent(input, this::computeExpensiveOperation);
}
private String computeExpensiveOperation(String input) {
// Perform the expensive operation here
return "result"; // Result of the expensive operation
}
}
In this example, the getResult
method first checks the cache for the result. If it's not found, the computeExpensiveOperation
method is called to compute the result, which is then cached using computeIfAbsent
. Subsequent calls with the same input will retrieve the result from the cache, thus avoiding the expensive operation.
Understanding Memoization
Memoization is a specific form of caching that stores the result of expensive function calls and returns the cached result when the same inputs occur again. It's a powerful technique to optimize recursive and repetitive function calls by storing the results and avoiding redundant computations.
Applying Memoization in Java
Let's explore how memoization can be employed in Java using a recursive Fibonacci calculation as an example.
import java.util.HashMap;
import java.util.Map;
public class FibonacciMemoization {
private Map<Integer, Integer> cache = new HashMap<>();
public int calculateFibonacci(int n) {
if (cache.containsKey(n)) {
return cache.get(n);
}
if (n <= 1) {
return n;
}
int result = calculateFibonacci(n - 1) + calculateFibonacci(n - 2);
cache.put(n, result);
return result;
}
}
In this instance, the calculateFibonacci
method utilizes memoization to store the computed results in the cache. If the result for a specific input n
is found in the cache, it is immediately returned, preventing redundant calculations.
Advantages of Micro-Caching and Memoization
Performance Boost
Both micro-caching and memoization provide a significant performance boost by avoiding repetitive expensive computations. These techniques can lead to dramatic speed improvements, especially in scenarios with high computational complexity.
Reduced Resource Utilization
By storing and reusing results, micro-caching and memoization can reduce the utilization of CPU and memory resources. This improvement in resource efficiency can lead to smoother and more responsive application performance.
Scalability
The performance gains achieved through micro-caching and memoization contribute to the overall scalability of the application. As the workload increases, these techniques help in maintaining optimal performance without overburdening the system.
Closing the Chapter
Incorporating micro-caching and memoization into your Java applications can unlock significant performance enhancements. By intelligently caching the results of expensive operations and storing the outcomes of repetitive function calls, you can optimize your app's speed, resource utilization, and scalability.
To delve deeper into the world of caching in Java, check out this article on Java Caching System.
Optimize your applications today with micro-caching and memoization, and witness the magic of improved speed and efficiency!
Checkout our other articles