Unlock the Mystery: Solving Deadlocks with Explicit Locking!
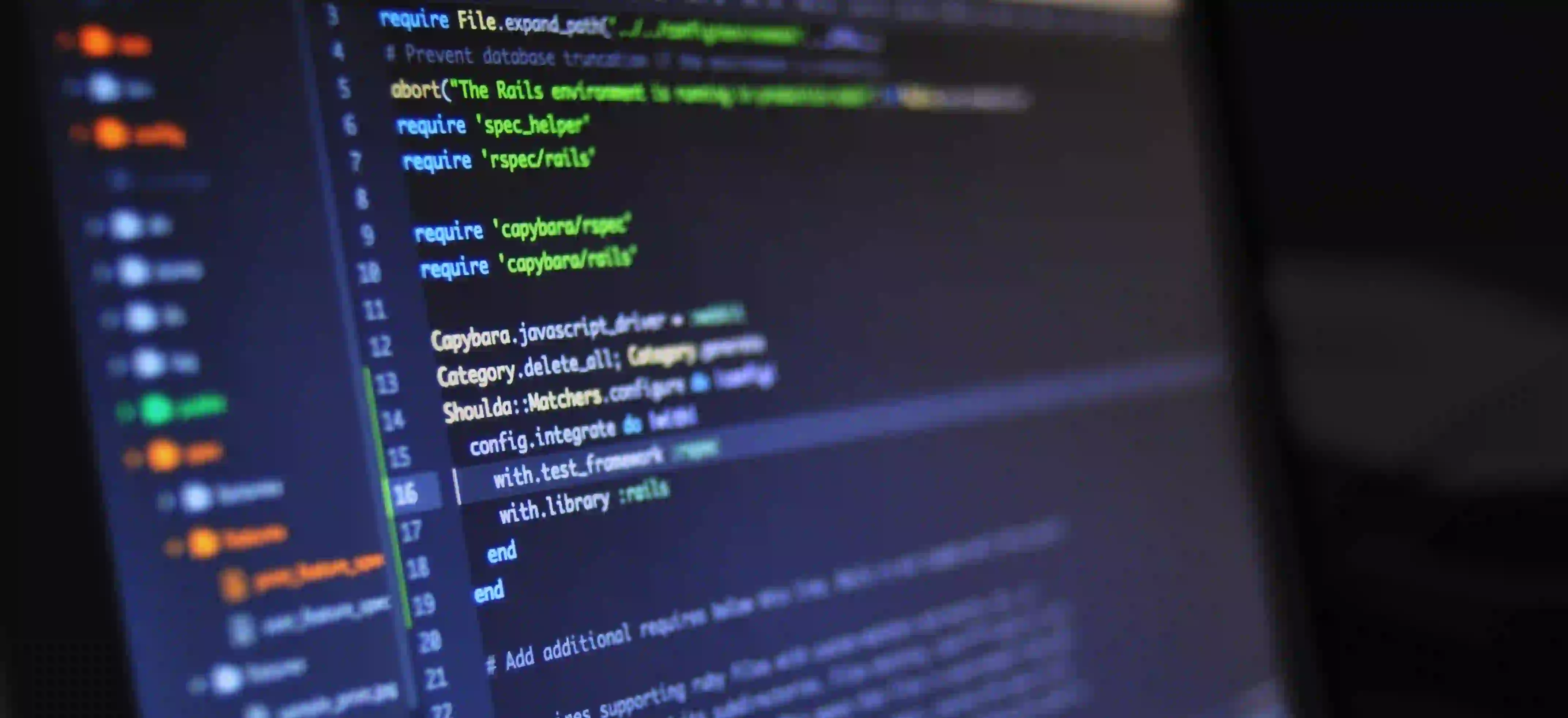
Unlock the Mystery: Solving Deadlocks with Explicit Locking in Java
Deadlocks in Java can turn your smooth-running application into a stalled nightmare. It's like finding yourself in a horror movie where your threads are the protagonists, and the deadlock is the monster lurking in the shadows. But fear not! Explicit Locking in Java provides a powerful flashlight to cut through the darkness. This blog post will guide you through understanding deadlocks and how explicit locking can be your key to solving and preventing them.
What is a Deadlock?
Imagine you're in a room with two locks that need keys from each other's lockboxes. You need both keys to exit, but you can only open one lockbox at a time. If someone else has the same idea and grabs the other lockbox, both of you are stuck indefinitely. That's deadlock in a nutshell – a situation in programming where two or more threads are waiting on each other to release resources, creating a cycle of dependencies that puts them into eternal waiting.
In technical terms, a deadlock requires four conditions to happen simultaneously:
- Mutual Exclusion: Limited resources can be used by only one thread at a time.
- Hold and Wait: Threads hold on to resources while waiting for others.
- No Preemption: Resources cannot be forcibly taken away from threads.
- Circular Wait: A closed chain of threads exists, where each thread holds a resource needed by the next.
Understanding Deadlocks with an Example
Let's dive into a simple example to see a deadlock in action:
public class DeadlockDemo {
private static Object Lock1 = new Object();
private static Object Lock2 = new Object();
private static Thread firstThread = new Thread(() -> {
synchronized (Lock1) {
System.out.println("Thread 1: Holding lock 1...");
try { Thread.sleep(10); } catch (InterruptedException e) {}
System.out.println("Thread 1: Waiting for lock 2...");
synchronized (Lock2) {
System.out.println("Thread 1: Holding lock 1 & 2...");
}
}
});
private static Thread secondThread = new Thread(() -> {
synchronized (Lock2) {
System.out.println("Thread 2: Holding lock 2...");
try { Thread.sleep(10); } catch (InterruptedException e) {}
System.out.println("Thread 2: Waiting for lock 1...");
synchronized (Lock1) {
System.out.println("Thread 2: Holding lock 2 & 1...");
}
}
});
public static void main(String[] args) {
firstThread.start();
secondThread.start();
}
}
In this code snippet, firstThread
and secondThread
are both trying to obtain Lock1
and Lock2
. However, they attempt to do so in reverse order, creating the perfect conditions for a deadlock. They each grab a lock and then pause (Thread.sleep(10)
), simulating some operation. During the pause, the other thread grabs the remaining lock. Then, they both wait forever for the other lock to be released.
Breaking Free: Enter Explicit Locking
Java provides a more flexible and sophisticated way to handle locks through the java.util.concurrent.locks.Lock
interface and its implementations, such as ReentrantLock
. This approach provides methods to try to acquire a lock without blocking indefinitely, thus offering a solution to avoid deadlocks.
Why Use Explicit Locking?
Explicit locking gives you more control over lock acquisition and release, plus additional features:
- Try Lock: Attempt to acquire a lock without waiting forever.
- Lock Interruptibly: Acquire a lock unless the thread is interrupted.
- Fairness: Optionally, make the lock fair so that the longest-waiting thread gets the lock next.
Solving Our Deadlock with ReentrantLock
Let's solve the earlier deadlock issue using ReentrantLock
:
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class DeadlockSolution {
private static Lock lock1 = new ReentrantLock();
private static Lock lock2 = new ReentrantLock();
private static void acquireLocks(Lock firstLock, Lock secondLock) throws InterruptedException {
while (true) {
// Acquire locks without waiting indefinitely
boolean gotFirstLock = false;
boolean gotSecondLock = false;
try {
gotFirstLock = firstLock.tryLock();
gotSecondLock = secondLock.tryLock();
}
finally {
if (gotFirstLock && gotSecondLock) {
return;
}
if (gotFirstLock) {
firstLock.unlock();
}
if (gotSecondLock) {
secondLock.unlock();
}
}
// Locks not acquired, waiting a bit before retrying
Thread.sleep(1);
}
}
public static void main(String[] args) {
Thread t1 = new Thread(() -> {
try {
acquireLocks(lock1, lock2);
System.out.println("Thread 1: Holding both locks");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock1.unlock();
lock2.unlock();
System.out.println("Thread 1: Released locks");
}
});
Thread t2 = new Thread(() -> {
try {
acquireLocks(lock2, lock1);
System.out.println("Thread 2: Holding both locks");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
lock1.unlock();
lock2.unlock();
System.out.println("Thread 2: Released locks");
}
});
t1.start();
t2.start();
}
}
In this improved version, the acquireLocks
method attempts to acquire both locks using tryLock()
. If it can't get both, it releases any lock it did get and tries again. This approach eliminates the deadlock risk because it ensures that both locks must be acquired for the operation to proceed; if not, it backs off and retries. This type of lock handling is not directly possible with intrinsic locks obtained through synchronized
blocks or methods.
Best Practices for Avoiding Deadlocks
While explicit locking provides tools to prevent deadlocks, following best practices is crucial in designing deadlock-free applications:
- Lock Ordering: Always acquire locks in a consistent global order.
- Lock Timeout: Use
tryLock
with a timeout instead of blocking indefinitely. - Lock Minimization: Hold locks for the shortest time possible.
- Deadlock Detection: Implement detection and recovery mechanisms.
To dive deeper into concurrency in Java and gain more insights into mechanisms like explicit locks, check out the official concurrency tutorial available on the Oracle website.
Moreover, for advanced readers looking to master the intricacies of Java concurrency and thread management, the book "Java Concurrency in Practice" by Brian Goetz is an invaluable resource. It's a deep dive into understanding and solving concurrency issues in Java applications.
To Wrap Things Up
Deadlocks can severely impact the responsiveness and reliability of your Java applications. However, by understanding the conditions that lead to deadlocks and applying explicit locking strategies as discussed, developers can design robust, deadlock-free applications. Remember, preventing deadlocks is not just about using the right tools; it's about thoughtful design and following best practices in concurrency. Equip yourself with these strategies, and you'll be well on your way to mastering the art of concurrency in Java.
Solving deadlock issues is akin to unlocking a mystery. With the right knowledge and tools, what once seemed like an insurmountable challenge can be systematically understood and resolved. As you continue to develop and refine your Java applications, keep the lessons of explicit locking and concurrency best practices as key tools in your developer toolkit.