Tricky Transition: Flawlessly Converting Map Back to Objects
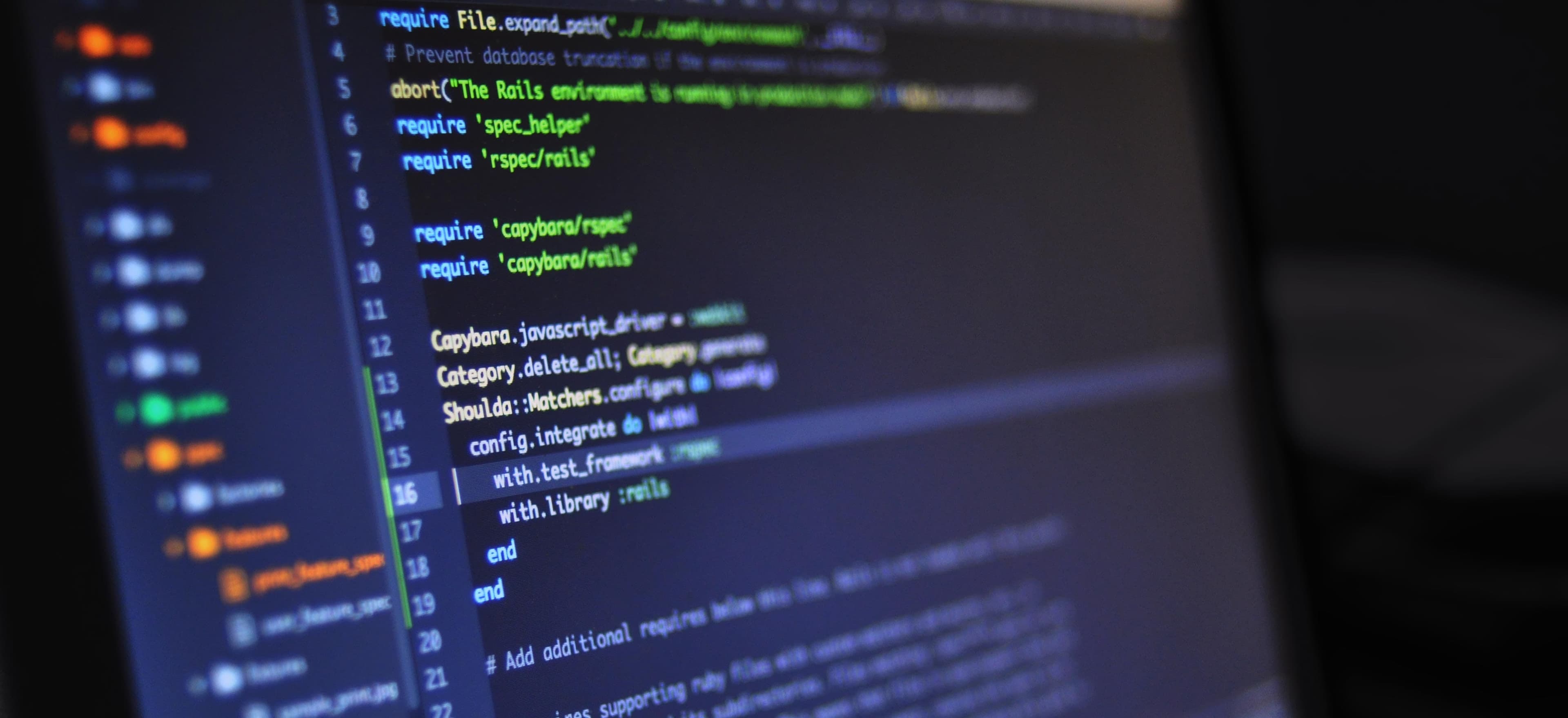
- Published on
Tricky Transition: Flawlessly Converting Map Back to Objects
When working with Java, you might often find yourself dealing with Map
data structures, such as HashMap
or LinkedHashMap
. These can be incredibly useful for storing key-value pairs, but there are times when you need to convert the map back to objects, and this process can be tricky to get right.
In this blog post, we'll explore the nuances of converting a Map
back to objects in Java. We'll discuss various approaches, highlight potential pitfalls, and provide best practices to ensure a smooth and effective transition. Let's dive in!
Understanding the Challenge
Consider a scenario where you retrieve data from a database or an API, and the result is delivered as a Map
where the keys correspond to the fields of an object. You want to convert this Map
back to an object to work with it more conveniently in your code.
Map<String, Object> data = fetchDataFromSource(); // Assume this method retrieves data as a Map
The challenge here is to seamlessly convert the Map
back to an object of a specific class, taking into account data types, error handling, and potential mismatches between the map keys and the object's fields.
Approach 1: Manual Conversion
One approach to convert a Map
back to an object is to manually iterate over the map entries and assign values to the corresponding fields of the object. Let's consider the following User
class as an example:
public class User {
private String name;
private int age;
// Getters and Setters omitted for brevity
// Constructor omitted for brevity
}
You could perform the conversion as follows:
User user = new User();
user.setName((String)data.get("name"));
user.setAge((int)data.get("age"));
While this manual approach may seem straightforward, it comes with significant drawbacks. It's error-prone, as a simple typo in a field name can lead to runtime errors. Additionally, it's not scalable; imagine handling a map with dozens of fields and a corresponding object with many properties.
Approach 2: Apache Commons BeanUtils
To simplify the conversion from a Map
to an object, you can leverage the BeanUtils
class from Apache Commons, which provides utility methods for populating JavaBeans properties from maps. Here's how you can use it:
User user = new User();
BeanUtils.populate(user, data);
This approach saves you from manually iterating over the map and assigning values to object fields. It automatically performs type conversion for basic data types, such as String
to int
, and provides better error handling. However, it's important to note that this method may not handle complex object graphs or custom type conversions seamlessly.
Approach 3: Jackson ObjectMapper
Another powerful tool for converting a Map
back to an object is the ObjectMapper
class from the Jackson library. Jackson is widely used for JSON processing, but it can also handle map-to-object conversions effortlessly.
Assuming the same User
class, the conversion can be achieved as follows:
ObjectMapper objectMapper = new ObjectMapper();
User user = objectMapper.convertValue(data, User.class);
By using Jackson's ObjectMapper
, you benefit from robust serialization and deserialization capabilities, advanced error handling, and support for complex mappings and custom data types. It's a versatile solution that covers a wide range of use cases.
Best Practices and Considerations
When engaging in the process of converting a Map
back to objects in Java, consider the following best practices and important points:
-
Error Handling: Always handle potential errors, such as type mismatches or missing keys in the map, to prevent unexpected exceptions.
-
Mapping Complexity: Be mindful of the complexity of the mapping. While simple cases can be handled with basic libraries or manual conversion, complex mappings and custom data types may require more sophisticated approaches such as Jackson's
ObjectMapper
. -
Unit Testing: Thoroughly test your conversion logic with various types of input data to ensure its correctness and resilience to edge cases.
-
Performance: Evaluate the performance implications of different conversion approaches, particularly when dealing with large datasets or frequent conversions.
-
Maintainability: Strive for maintainable and readable code. Consider how easy it would be for another developer to understand and modify the conversion logic.
-
Version Compatibility: Ensure that the libraries or frameworks you use for conversion are compatible with your project's Java version.
By adhering to these best practices and considerations, you can streamline the process of converting Map
data back to objects and ensure the robustness and reliability of your code.
My Closing Thoughts on the Matter
Converting a Map
back to objects in Java presents a common yet challenging task, especially when dealing with complex data structures and diverse use cases. While manual conversion is a straightforward but error-prone approach, leveraging libraries like Apache Commons BeanUtils and Jackson ObjectMapper can significantly simplify and enhance the conversion process.
As you navigate this often-tricky transition, be mindful of error handling, mapping complexity, performance, and maintainability to craft robust and efficient conversion logic.
With the insights and best practices shared in this article, you are now better equipped to flawlessly convert Map
data back to objects in Java, empowering your code with clarity, efficiency, and reliability.
Now, go forth and conquer those conversions with confidence!
Happy coding!
Checkout our other articles