Solving Jenkins Pipeline Woes: A Field Note Guide
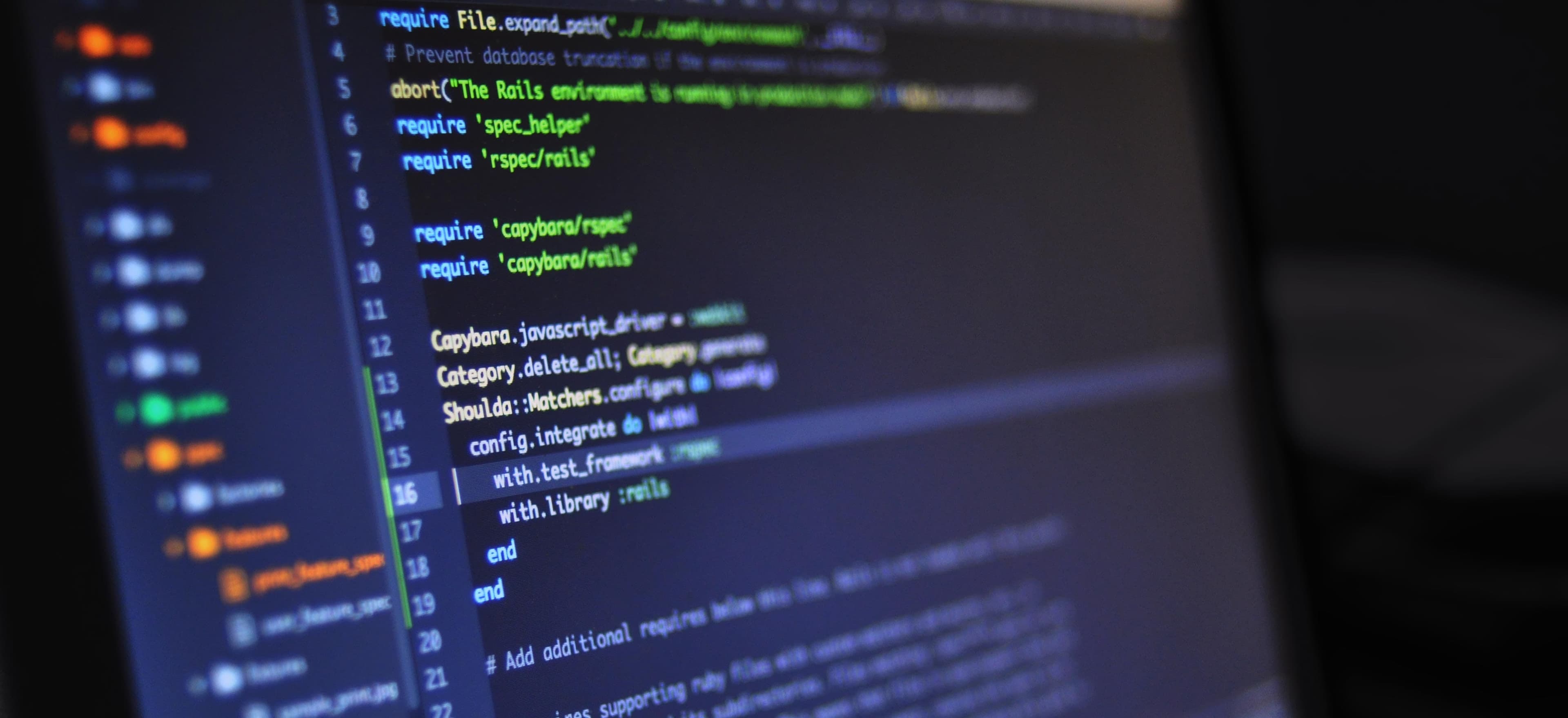
- Published on
Solving Jenkins Pipeline Woes: A Field Note Guide
When it comes to continuous integration and continuous deployment, Jenkins is one of the most popular tools out there. With its powerful capabilities for automating tasks, Jenkins has become a staple in the software development industry. However, even with its robust features, setting up and maintaining Jenkins pipelines can sometimes be a challenging and frustrating task.
In this blog post, we'll explore some common issues that developers encounter when working with Jenkins pipelines and provide practical solutions to address these challenges. From dealing with pipeline failures to optimizing pipeline performance, we'll cover a range of scenarios to help you streamline your CI/CD process with Jenkins.
Dealing with Pipeline Failures
One of the most common issues with Jenkins pipelines is dealing with pipeline failures. When a pipeline fails, it can be challenging to pinpoint the root cause of the failure, especially in complex pipeline setups.
Example of a Pipeline Failure:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
In the example above, if the mvn test
command fails during the Test stage, the entire pipeline will fail. To address this, you can use the try-catch block to catch and handle specific exceptions:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Test') {
steps {
try {
sh 'mvn test'
} catch (Exception e) {
// Handle the test failure
echo "Tests failed: ${e.message}"
}
}
}
}
}
In this modified pipeline, the try-catch
block catches the exception thrown by the mvn test
command and allows you to handle the failure gracefully. You can customize the error handling based on your specific requirements, such as sending notifications or triggering alternative actions.
Optimizing Pipeline Performance
Another common challenge with Jenkins pipelines is optimizing pipeline performance, especially as pipelines grow in complexity and scale. Long-running pipelines can significantly impact development velocity and resource utilization.
To optimize pipeline performance, consider the following strategies:
Parallelizing Stages
pipeline {
agent any
stages {
stage('Build and Test') {
parallel {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Run Tests') {
steps {
sh 'mvn test'
}
}
}
}
}
}
In this example, the Build
and Run Tests
stages are executed in parallel, leveraging the available resources more efficiently and reducing the overall pipeline execution time.
Caching Dependencies
pipeline {
agent any
options {
// Cache Maven dependencies to improve build performance
skipDefaultCheckout(true)
caches {
maven 'm2repository'
}
}
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
}
}
By caching dependencies, such as Maven artifacts, you can reduce the build time by reusing previously downloaded dependencies, thereby improving the overall pipeline performance.
Integrating Automated Testing
Automated testing is a critical aspect of any CI/CD pipeline. However, configuring and managing automated tests within Jenkins pipelines can present its own set of challenges.
Example of Running Automated Tests:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Run Automated Tests') {
steps {
sh 'mvn verify'
}
}
}
}
To integrate automated testing effectively, consider utilizing plugins such as JUnit and TestNG for generating test reports and integrating them with Jenkins for comprehensive test result analysis.
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean package'
}
}
stage('Run Automated Tests') {
steps {
sh 'mvn verify'
}
post {
always {
junit 'target/surefire-reports/**/*.xml'
}
}
}
}
}
In this revised pipeline, the junit
step collects and archives the test results, allowing for detailed analysis within Jenkins. Additionally, integrating with quality gates or approval processes based on test results can further enhance the automated testing workflow.
Closing the Chapter
In this post, we've covered some common challenges encountered when working with Jenkins pipelines and provided practical solutions to address these issues. By leveraging error handling, optimizing performance, and integrating automated testing, you can enhance the stability, efficiency, and reliability of your CI/CD pipelines.
Remember, Jenkins offers a wide range of plugins and integrations that can further extend its capabilities, so don't hesitate to explore and experiment with additional tools to tailor your pipeline to your specific needs.
With these tips and best practices in mind, you can streamline your Jenkins pipeline and navigate through potential woes with confidence.
Happy automating!