Overcoming Slow Boot Times in Scala Play for Startups
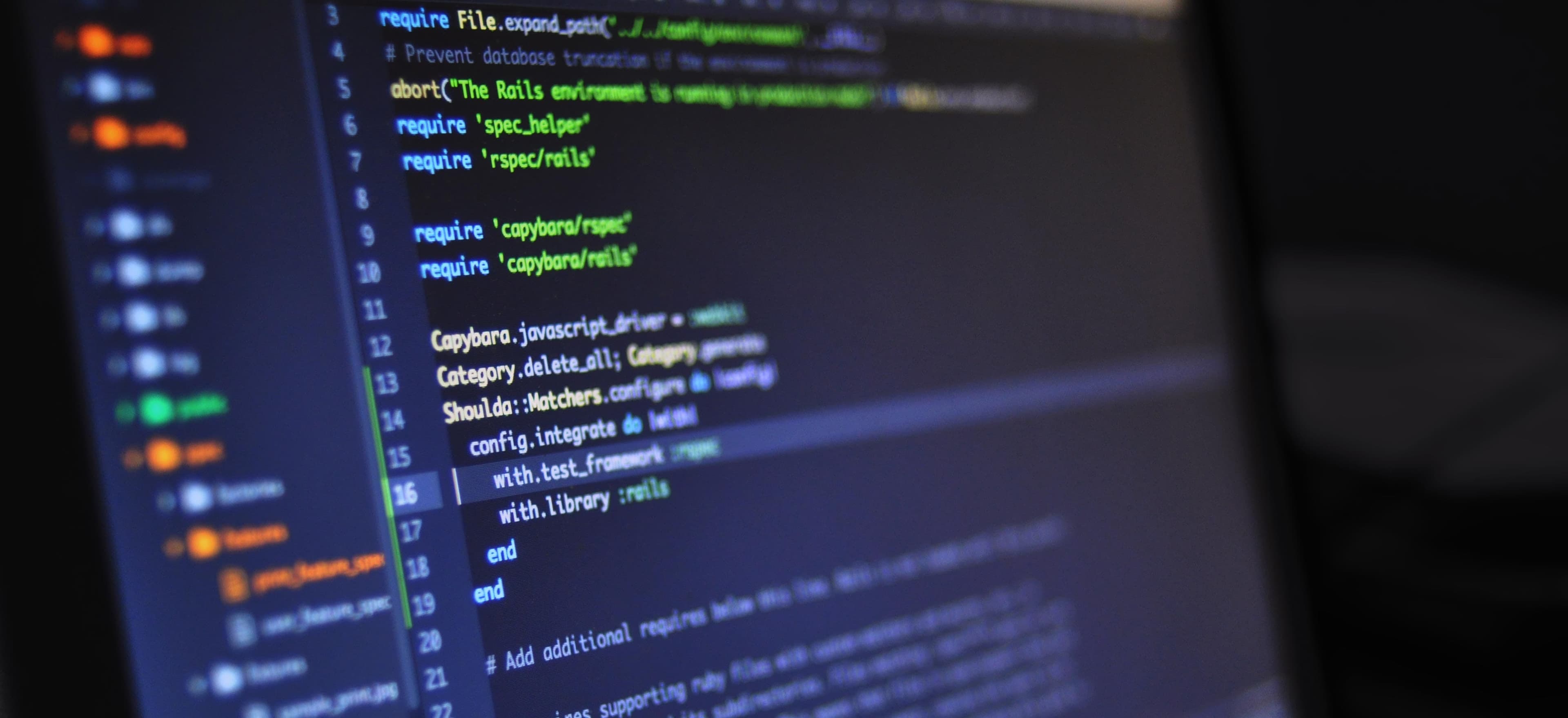
- Published on
Overcoming Slow Boot Times in Scala Play for Startups
As a startup founder or developer, slow boot times can be a significant hindrance to the productivity and usability of your Scala Play application. Slow boot times can frustrate both your team and your users, leading to a decrease in overall efficiency and satisfaction. In this article, we'll explore the potential causes of slow boot times in Scala Play applications for startups and discuss effective strategies to overcome this issue.
Understanding the Causes of Slow Boot Times
Before diving into solutions, it's crucial to understand what could be causing slow boot times in your Scala Play application. Some common factors that contribute to slow boot times include:
-
Heavy Application Initialization: If your Scala Play application requires a long time to initialize due to complex configurations or extensive dependencies, it can significantly slow down the boot time.
-
Inefficient Code: Poorly optimized code, including inefficient algorithms or resource-intensive operations, can lead to slow boot times as the application takes longer to initialize.
-
Large Number of Dependencies: A high volume of external dependencies, such as libraries or frameworks, can increase the boot time as the application needs to load and initialize these dependencies during startup.
Strategies to Overcome Slow Boot Times
1. Profile and Optimize Application Initialization
Problem: Heavy application initialization can cause slow boot times as the application takes time to set up various components.
Solution: Use profiling tools such as YourKit or JProfiler to identify bottlenecks in the initialization process. Once identified, focus on optimizing critical initialization steps, such as database connections, caching setups, or dependency injection. Additionally, consider lazy initialization for components that are not essential during startup, deferring their setup to a later stage in the application lifecycle.
2. Focus on Code Optimization
Problem: Inefficient code can lead to slow boot times as the application struggles to execute resource-intensive operations during startup.
Solution: Conduct code reviews and performance profiling to identify areas of the codebase that can be optimized. Utilize effective algorithms and data structures to improve the efficiency of critical operations. Additionally, consider asynchronous and non-blocking patterns to parallelize tasks during initialization, reducing the overall boot time.
// Example of non-blocking initialization using Future in Scala
import scala.concurrent.Future
import scala.concurrent.ExecutionContext.Implicits.global
val initializationTask: Future[Unit] = Future {
// Perform resource-intensive initialization tasks
}
initializationTask.onComplete { _ =>
// Continue application startup after initialization is complete
}
In this example, the use of Future
allows resource-intensive tasks to run concurrently, speeding up the initialization process.
3. Dependency Optimization
Problem: A large number of dependencies can contribute to slow boot times as the application needs to load and initialize these dependencies during startup.
Solution: Evaluate the necessity of each dependency and minimize the number of non-essential libraries or frameworks. Utilize tools such as sbt's dependency graph to visualize and understand the dependency hierarchy. Additionally, consider using modularization to load dependencies on demand, rather than all at once during application startup.
Embracing Reactive Programming
Reactive programming can be a game-changer in overcoming slow boot times in Scala Play applications. By embracing reactive principles, such as asynchronous and non-blocking operations, startups can significantly improve the responsiveness and boot times of their applications.
Scala Play provides excellent support for reactive programming through libraries like Akka and Play JSON. Leveraging these libraries and adopting reactive design patterns can streamline the application's startup process, resulting in faster boot times and improved overall performance.
// Example of non-blocking database access using Akka in Scala Play
import akka.pattern.ask
import akka.util.Timeout
import scala.concurrent.duration._
import akka.actor.ActorSystem
import play.api.libs.concurrent.Akka
import play.api.Play.current
implicit val system: ActorSystem = Akka.system
implicit val timeout: Timeout = Timeout(5.seconds)
val databaseActor = // Obtain a reference to the database actor
val query = // Define the database query
val resultFuture = databaseActor ? query // Non-blocking database query using ask pattern
resultFuture.map { result =>
// Process the database query result
}
In this example, the use of Akka enables non-blocking database access, allowing the application to continue initialization while awaiting the query result.
Lessons Learned
Slow boot times in Scala Play applications can be a significant hurdle for startups, impacting both developer productivity and user experience. By addressing the causes of slow boot times and implementing strategies such as application profiling, code optimization, and embracing reactive programming, startups can effectively overcome this challenge and create responsive, high-performance Scala Play applications.
By applying these strategies and leveraging the principles of reactive programming, startups can streamline the boot time of their Scala Play applications, ensuring a seamless and efficient user experience.
Remember, addressing slow boot times is a continuous process, and ongoing optimization and refinement are essential to maintain optimal application startup performance.
For additional insights and best practices regarding Scala Play applications and reactive programming, be sure to check out the Lightbend website.
Start optimizing your Scala Play application's boot time today and pave the way for a faster, more responsive user experience!