Overcoming Common System Observability Challenges in Production
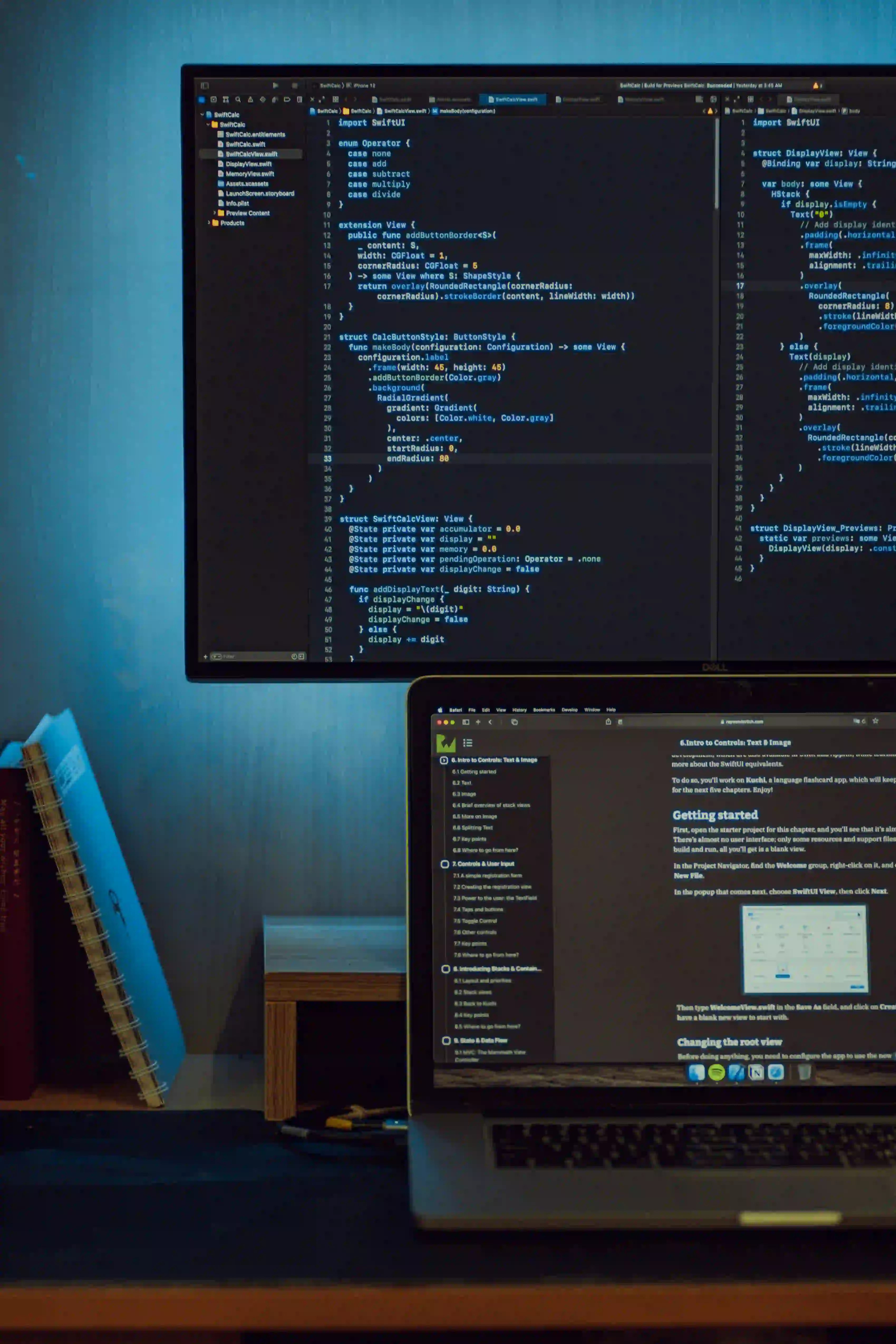
Overcoming Common System Observability Challenges in Production
Observability is critical in modern distributed systems. As organizations adopt microservices architectures and cloud-based strategies, the complexities of applications increase significantly. Along with these complexities come various challenges that developers and operations teams must address to ensure that systems remain healthy, performant, and reliable in production.
In this post, we will explore common system observability challenges and discuss practical approaches to overcome them, ensuring that you can maintain exceptional performance and minimize downtimes in your production environments.
1. Challenge: Lack of Clear Metrics
The Problem
One of the most significant observations challenges is the absence of clear, actionable metrics. Developers often get caught up in the volume of data generated by applications and overlook relevant metrics for their specific environment.
The Solution
To combat this challenge, follow a systematic approach:
-
Define Key Performance Indicators (KPIs): Identify the metrics critical to your applications' performance. Often, these include latency, error rates, and resource consumption.
-
Use Standard Metrics: Leverage frameworks that provide standard observability metrics. Prometheus is widely adopted for Kubernetes environments, making it easier to track, alert, and visualize metrics.
Code Example: Collecting Metrics with Prometheus
Here is a basic example of how to integrate Prometheus with a Java application using Spring Boot.
import io.prometheus.client.Counter;
import io.prometheus.client.spring.web.PrometheusMvcInterceptor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class PrometheusConfig implements WebMvcConfigurer {
private static final Counter requestCounter = Counter.build()
.name("request_total").help("Total requests.").register();
public void incrementCounter() {
requestCounter.inc();
}
@Bean
public PrometheusMvcInterceptor prometheusMvcInterceptor() {
return new PrometheusMvcInterceptor();
}
}
Why This Code Matters: Here, we define a metrics counter for total requests handled by the application. Each time a request is made, the incrementCounter
method will increment the counter, allowing us to monitor requests effectively.
2. Challenge: Data Overload
The Problem
With so much data generated by modern applications, filtering out noise and focusing on useful insights can be incredibly challenging.
The Solution
Implement logging and monitoring tools that can aggregate and analyze data without causing information overload. Consider the following practices:
-
Sampling and Rate Limiting: Implement data sampling techniques to limit the volume of logs sent to your monitoring tools.
-
Structured Logging: Adopt structured logging on your application side. Use formats like JSON which allow greater flexibility in analyzing and filtering logs.
Code Example: Using Structured Logging in Spring Boot
Utilizing SLF4J with JSON formatting for logs can significantly improve log clarity:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyService {
private static final Logger logger = LoggerFactory.getLogger(MyService.class);
public void performTask() {
logger.info("{\"event\":\"task_start\", \"timestamp\":\"{}\"}", System.currentTimeMillis());
// Task execution logic
logger.info("{\"event\":\"task_end\", \"timestamp\":\"{}\"}", System.currentTimeMillis());
}
}
Why This Code Matters: This example demonstrates how to log structured JSON messages during the execution of a task within an application. It helps you capture event data in a way conducive to automation and analysis.
3. Challenge: Identifying the Root Cause of Issues
The Problem
Even with excellent metrics and logging in place, identifying the root cause of an issue can still be a daunting task. Often, issues are more abstract, hidden in the correlation of various metrics.
The Solution
-
Tracing: Use distributed tracing to visualize the flow of requests across services. Tools like OpenTracing and Jaeger can offer valuable insights.
-
Correlation IDs: Implement correlation IDs across your microservices. This practice enables you to trace a request as it passes through various services.
Code Example: Injecting Correlation ID in a Spring Boot Application
You can manage correlation IDs in a Spring application using filters. Here’s how:
import javax.servlet.*;
import javax.servlet.http.HttpServletRequest;
import java.io.IOException;
public class CorrelationIdFilter implements Filter {
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest httpServletRequest = (HttpServletRequest) request;
String correlationId = httpServletRequest.getHeader("X-Correlation-ID");
if (correlationId == null) {
correlationId = generateNewCorrelationId(); // Implement this method
}
// Here you would add the correlation ID to your logging context
MDC.put("correlationId", correlationId);
try {
chain.doFilter(request, response);
} finally {
MDC.clear(); // Clean up after finishing the request
}
}
}
Why This Code Matters: This filter captures incoming requests, retrieves or generates a correlation ID, and sets it in the logging context. This will enable tracing through your logs as correlated requests are logged.
4. Challenge: Managing Multiple Tools
The Problem
As companies scale their observability solutions, they often fall into the trap of managing multiple disparate tools. Pulling insights from these various sources can become a full-time job in itself.
The Solution
Consider using full-stack observability platforms that encompass various elements like logs, metrics, and traces in a single interface. Some recommended tools include Elastic Stack and Grafana.
Integration Example: Combining Tools
Many organizations prefer to use a combination of tools like Prometheus for metrics and ELK Stack for logs. Each metric can be visualized directly in Grafana, while logs can be searched in Kibana, providing holistic insights.
5. Challenge: Alert Fatigue
The Problem
Frequent irrelevant alerts can lead teams to grow desensitized to notifications, potentially overlooking critical issues that actually require attention.
The Solution
Set up alerting based on clearly defined thresholds and incorporate anomaly detection. The following practices can help:
-
Dynamic Thresholds: Implement dynamic thresholds based on historical performance rather than static limits.
-
Alert Scoping: Use aggregation methods to group similar events before alerting. This helps in reducing the number of notifications generated at once.
Code Concept: Defining Alert Rules in Prometheus
Use Prometheus AlertManager for setting alerting rules:
groups:
- name: example-alert
rules:
- alert: HighRequestLatency
expr: histogram_quantile(0.95, sum(rate(http_request_duration_seconds_bucket[5m])) by (le)) > 0.5
for: 10m
labels:
severity: warning
annotations:
summary: "Request latency is too high"
description: "Request latency is above 0.5s for more than 10 minutes."
Why This Code Matters: The code defines an alert rule that triggers when the 95th percentile of request latency exceeds 0.5 seconds for 10 minutes. This ensures that alerts are relevant and based on calculated performance thresholds.
The Last Word
Observability in production systems is an ongoing process that requires continuous refinement. By implementing effective practices, such as defining key metrics, utilizing distributed tracing, and managing alerts intelligently, you can vastly improve your system's observability.
Investing time in these aspects pays off in reduced downtime, quicker incident responses, and overall enhanced performance of your applications. With the right tools and strategies, your observability challenges can turn into opportunities for better insight and continuous delivery.
For further readings on observability, check out Google's Site Reliability Engineering or dive into the resources provided by OpenTelemetry.
Whether you're just getting started or looking to refine your observability practices, the best time to act is now.