Enhancing Cybersecurity with Open Source Tools
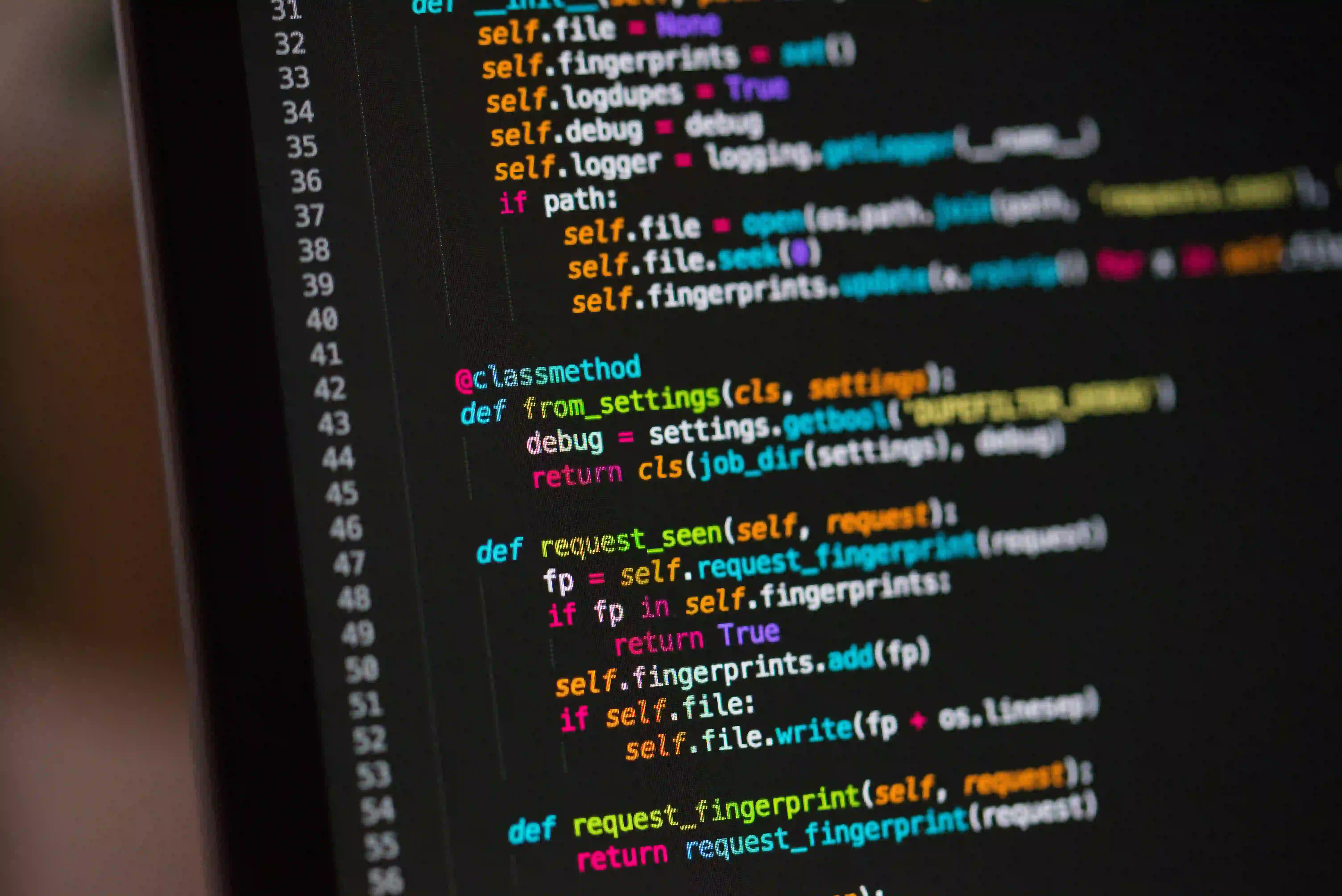
Enhancing Cybersecurity with Open Source Java Tools
In today's digital age, cybersecurity is more important than ever. With the increasing frequency and sophistication of cyber threats, it's crucial for individuals and organizations to proactively protect their digital assets. Java, as a powerful and versatile programming language, offers a myriad of open source tools that can significantly enhance cybersecurity measures. In this blog post, we'll explore some of the top open source Java tools that can be leveraged to bolster cybersecurity.
1. Apache Shiro
Apache Shiro is a powerful and easy-to-use Java security framework that performs authentication, authorization, cryptography, and session management. It provides a simple API that can be integrated into any application, whether it's a standalone Java application, a web application, or a microservice.
By using Apache Shiro for authentication and authorization, developers can ensure that only authenticated and authorized users have access to sensitive resources. This helps prevent unauthorized access and strengthens the overall security posture of the application.
Example Usage:
// Authenticating a user
Subject currentUser = SecurityUtils.getSubject();
UsernamePasswordToken token = new UsernamePasswordToken(username, password);
currentUser.login(token);
In this example, we're using Apache Shiro to authenticate a user with a username and password. The Subject
and UsernamePasswordToken
classes are provided by Apache Shiro, making the authentication process straightforward and secure.
For more information on Apache Shiro, visit the official Apache Shiro website.
2. Keycloak
Keycloak is an open source identity and access management solution that provides features such as single sign-on, identity brokering, and social login. It's built on top of the OAuth 2.0 and OpenID Connect protocols, making it a robust choice for securing applications and services.
Keycloak seamlessly integrates with Java applications, allowing developers to incorporate strong authentication and authorization capabilities without reinventing the wheel. Additionally, it supports user federation, enabling the use of existing LDAP or Active Directory servers for authentication.
Example Usage:
// Verifying a user's access token
Keycloak keycloak = Keycloak.getInstance(
"https://keycloak.example.com/auth",
"realm",
"client",
"client-secret",
"username",
"password"
);
TokenVerifier verifier = keycloak.tokenVerifier();
TokenVerifier.Result result = verifier.parse(tokenString);
if (result.isValid()) {
// Token is valid
} else {
// Token is invalid
}
In this example, we're using the Keycloak Java adapter to verify the validity of a user's access token. The Keycloak
and TokenVerifier
classes provide a straightforward way to incorporate token verification into a Java application.
To learn more about Keycloak, check out the official Keycloak documentation.
3. OWASP ESAPI
The OWASP Enterprise Security API (ESAPI) is a set of reusable security components that can be used to build secure applications. It provides functions for encoding, validation, encryption, and more, helping developers mitigate common security vulnerabilities such as injection attacks, cross-site scripting, and insecure deserialization.
By leveraging the OWASP ESAPI library, Java developers can ensure that their applications are resilient against a wide range of security threats. The library follows best practices and is continuously updated to address emerging security challenges.
Example Usage:
// Encoding user input to prevent cross-site scripting
String userInput = "<script>alert('XSS attack')</script>";
String encodedInput = ESAPI.encoder().encodeForHTML(userInput);
In this example, we're using the OWASP ESAPI library to encode user input for HTML content. By doing so, we prevent potential cross-site scripting attacks by ensuring that any HTML content entered by the user is properly encoded.
For additional information on OWASP ESAPI, visit the official OWASP ESAPI GitHub repository.
Key Takeaways
In conclusion, open source Java tools offer robust solutions for enhancing cybersecurity in applications and services. Apache Shiro, Keycloak, and OWASP ESAPI are just a few examples of the many powerful tools available to Java developers. By incorporating these tools into their projects, developers can strengthen the security posture of their applications and better protect sensitive data from cyber threats.
Cybersecurity is an ongoing concern, and it's essential to stay vigilant and proactive in safeguarding digital assets. Open source Java tools provide a cost-effective and reliable means of bolstering cybersecurity measures, ensuring that applications and services remain resilient against evolving security challenges.
Remember, cybersecurity is everyone's responsibility, and by leveraging the power of open source Java tools, developers can contribute to a safer and more secure digital ecosystem.