Mastering Server Headers: Boost Your Download Speed!
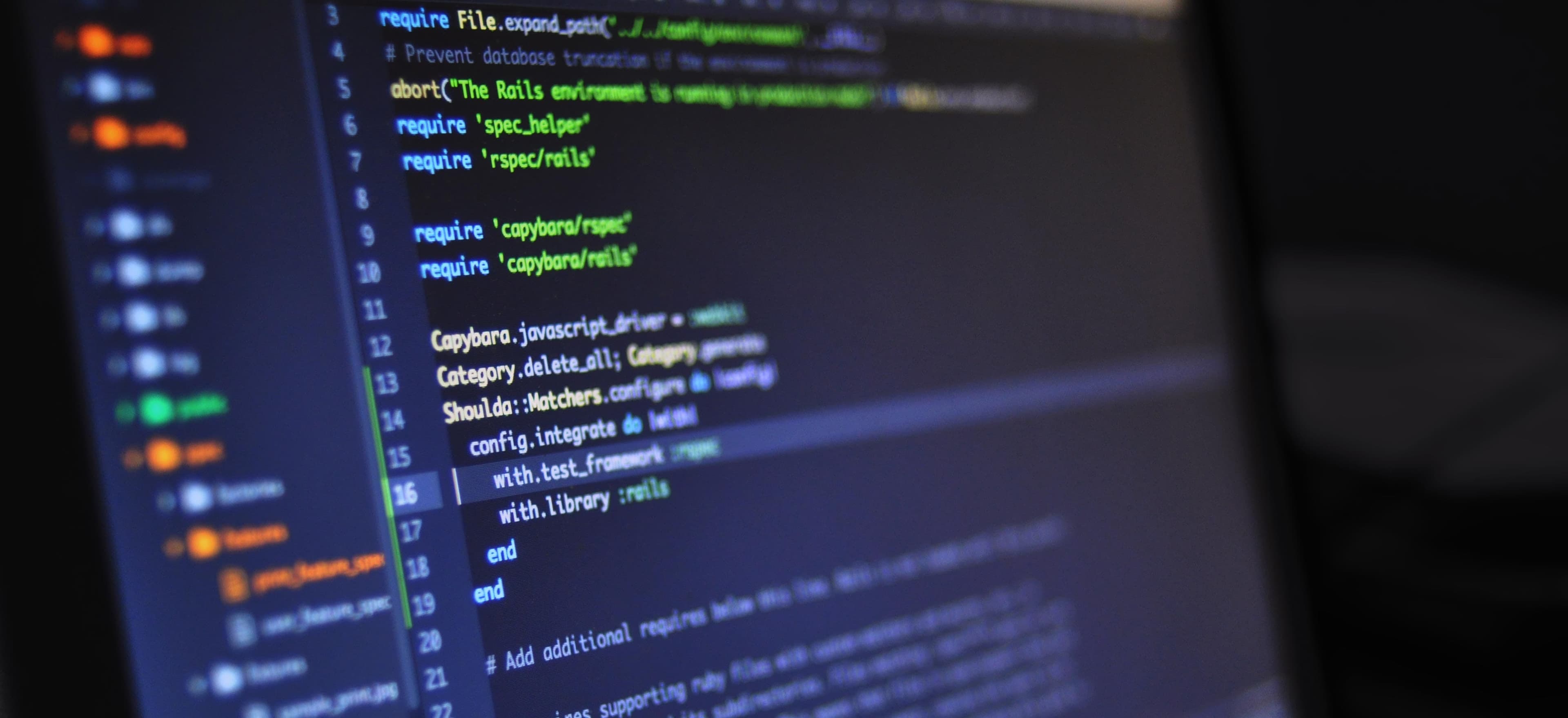
- Published on
Mastering Server Headers: Boost Your Download Speed!
In the realm of web development, every millisecond counts when it comes to user experience. Website loading speed is a critical factor that can make or break user engagement. One often overlooked aspect of optimizing website performance is leveraging server headers. In this post, we'll delve into server headers and explore how they can significantly boost your download speed. We'll also provide practical examples of using server headers in Java to optimize your web application.
Understanding Server Headers
Server headers are metadata pieces sent by the server to the client's web browser. These headers provide essential information about the server, the requested resource, and instructions for the client's browser on how to handle the response. By utilizing server headers effectively, developers can optimize various aspects of web performance, including caching, compression, security, and more.
Leveraging Server Headers for Performance Optimization
Caching with Cache-Control
Header
Caching is a pivotal technique for reducing load times and improving the overall user experience. By instructing the client's browser to cache certain resources, subsequent visits or page navigations can be expedited, since the browser can retrieve cached assets instead of re-downloading them from the server.
In Java, you can set the Cache-Control
header to enable client-side caching for specific resources. Below is a code snippet demonstrating how to set the Cache-Control
header in a Servlet:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Set cache control headers for one hour
response.setHeader("Cache-Control", "public, max-age=3600");
// Other processing and response handling
}
In this example, the Cache-Control
header is set to allow public caching with a maximum age of 3600 seconds (1 hour). By sending this header, the client's browser will cache the resource for the specified duration, leading to faster subsequent page loads.
Gzip Compression with Content-Encoding
Header
Compressing resources before transmitting them over the network can drastically reduce download times. Gzip compression, in particular, is widely supported by modern browsers and can significantly decrease the size of text-based resources such as HTML, CSS, and JavaScript files.
To enable Gzip compression for resources served by your Java application, you can set the Content-Encoding
header. Below is a sample code snippet illustrating how to apply Gzip compression to HTTP responses:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String text = "Sample text to be compressed"; // Sample uncompressed text
byte[] compressedBytes = gzipCompress(text.getBytes("UTF-8"));
response.setHeader("Content-Encoding", "gzip");
response.getOutputStream().write(compressedBytes);
// Other processing and response handling
}
In this example, the Content-Encoding
header is set to indicate Gzip compression for the response. The gzipCompress
method would be responsible for compressing the resource before it is sent back to the client.
Security Headers for Enhanced Protection
In addition to performance optimization, server headers can also be utilized to bolster security. For instance, the Strict-Transport-Security
header instructs the browser to only interact with the server over HTTPS, preventing potential security vulnerabilities like protocol downgrade attacks.
In a Java web application, you can set the Strict-Transport-Security
header to enforce secure communication. Here's a concise demonstration of adding the Strict-Transport-Security
header to your HTTP responses:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Enable strict transport security for one year
response.setHeader("Strict-Transport-Security", "max-age=31536000; includeSubDomains");
// Other processing and response handling
}
By including the Strict-Transport-Security
header with an appropriate max-age
directive, you can instruct the client's browser to interact with your web application only over HTTPS for the specified duration, thus enhancing security.
Bringing It All Together
Mastering the utilization of server headers is crucial for optimizing web performance. By incorporating techniques such as caching, compression, and security directives through server headers, you can significantly enhance the download speed and overall user experience of your Java web applications. Embracing these techniques empowers developers to deliver faster, more secure, and more efficient web experiences to their users. Start leveraging the power of server headers today and witness the tangible impact on your web application's performance!
Remember, in the fast-paced world of web development, every optimization, no matter how small, can make a substantial difference. So, don't overlook the potential of server headers in elevating your Java web application's performance.
For further reading on server headers and their impact on web performance, check out the MDN Web Docs and the HTTP/2 specifications. These resources provide in-depth insights into the vast world of HTTP headers and their potent influence on web optimization.
Checkout our other articles