Mastering State Management in Java with Enums
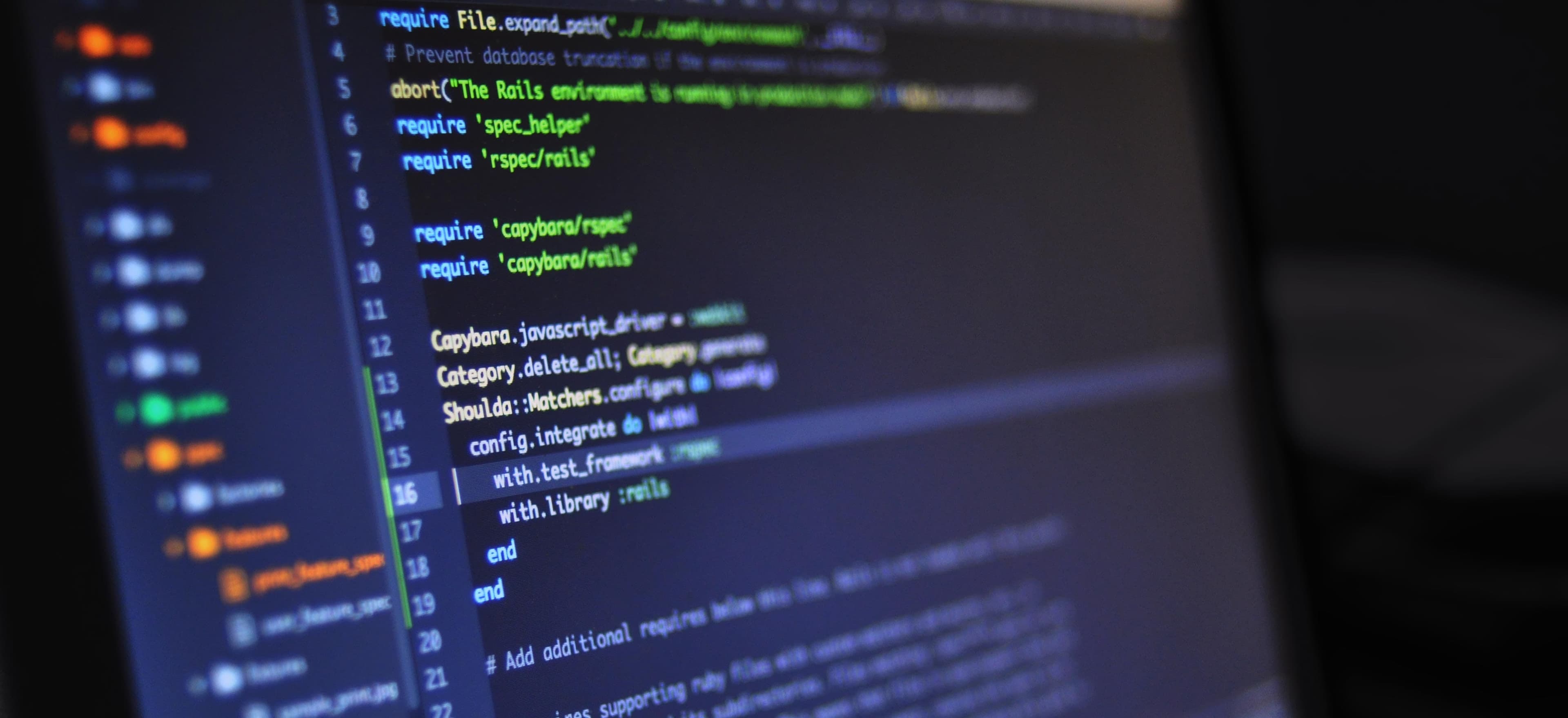
- Published on
Mastering State Management in Java with Enums
When developing applications in Java, state management is a critical component that can directly affect the maintainability and scalability of your code. One powerful tool available in Java is the enum (short for enumeration). Enums can play a vital role in managing the state of an object, offering readability, type safety, and more concise code.
In this blog post, we will explore the advantages of using enums for state management, provide practical examples, and discuss best practices to utilize enums effectively in your Java applications.
What are Enums?
In Java, enums are a special data type that enables for a variable to be a set of predefined constants. They are more than just a simple list of values; they can contain methods, constructors, and instance variables, allowing for complex behavior.
Basic Example
Let’s start with a simple example to understand how enums work:
public enum Day {
SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY
}
In this example, we created an enum called Day
representing the days of the week. Each of these constants can be used wherever a Day is needed, providing type safety and clear intent.
Why Use Enums for State Management?
- Type Safety: Enums restrict the variable to only accept values defined in the enum, reducing bugs.
- Clear Intent: Using enums makes code easier to read and understand. Instead of arbitrary integers or strings, you use meaningful names.
- Centralized State Logic: All related constants and logic are kept in a single location, improving maintainability.
- Switch-Case Statements: Enums work seamlessly with switch-case statements, improving code clarity.
Practical Application of Enums for State Management
Let us see how we can leverage enums to manage states in a real-world application. We will create a simple order processing system where orders can be in different states: NEW, PROCESSING, SHIPPED, and DELIVERED.
Step 1: Define the Enum
public enum OrderStatus {
NEW, PROCESSING, SHIPPED, DELIVERED;
public boolean isTerminal() {
return this == DELIVERED;
}
}
In this enum, we've not only defined the possible states, but we've also added a method isTerminal()
that checks if the current status is terminal (in this case, whether the order has been delivered).
Step 2: Create an Order Class
Next, we will create an Order
class to leverage our OrderStatus
enum.
public class Order {
private Long id;
private OrderStatus status;
public Order(Long id) {
this.id = id;
this.status = OrderStatus.NEW; // Initial status
}
public OrderStatus getStatus() {
return status;
}
public void processOrder() {
switch (status) {
case NEW:
status = OrderStatus.PROCESSING;
break;
case PROCESSING:
status = OrderStatus.SHIPPED;
break;
case SHIPPED:
status = OrderStatus.DELIVERED;
break;
default:
System.out.println("Order is already processed.");
}
}
}
Commentary on the Code
- Encapsulation of State: The
status
variable manages the different states of an order, making it easier to reason about the order's current state. - Switch Statement: The switch-case structure makes it clear how orders progress through states, enhancing readability.
- Safety through Enums: If you tried to assign a state to the
status
variable that isn’t defined inOrderStatus
, the compiler would throw an error, preventing potential bugs.
Step 3: Test the Order Processing System
Now, let’s test our Order
class:
public class Main {
public static void main(String[] args) {
Order order = new Order(1L);
System.out.println("Initial order status: " + order.getStatus());
order.processOrder();
System.out.println("Current order status: " + order.getStatus());
order.processOrder();
System.out.println("Current order status: " + order.getStatus());
order.processOrder();
System.out.println("Current order status: " + order.getStatus());
order.processOrder(); // Attempting to re-process
}
}
Expected Output
Initial order status: NEW
Current order status: PROCESSING
Current order status: SHIPPED
Current order status: DELIVERED
Order is already processed.
Expanding Functionality with Enums
Enums can also hold more complex behavior by attaching instance variables and methods. For example, we may want to assign a description to each order status:
public enum OrderStatus {
NEW("Order has been placed"),
PROCESSING("Order is being processed"),
SHIPPED("Order has been shipped"),
DELIVERED("Order has been delivered");
private String description;
OrderStatus(String description) {
this.description = description;
}
public String getDescription() {
return description;
}
}
Example Usage of Enhanced Enum
Now we can call getDescription()
to provide more context about the order status:
System.out.println("Order status: " + order.getStatus().getDescription());
Best Practices for Using Enums in Java
Here are some best practices when using enums in your Java applications:
- Limit Enum Size: Keep enums small and focused. If they grow too large or complex, reconsider your design.
- Use Enums for Fixed Sets: Enums are useful for a fixed set of related constants. Avoid using them for dynamic data sets.
- Implement Interfaces: Enums can implement interfaces, allowing for flexible design patterns. Consider this when working with diverse behaviors.
- Fallback Options: If applicable, provide a fallback option (like UNKNOWN) for safety.
- Documentation: Document your enums clearly to explain their purpose and usage. This aids future maintainability.
The Bottom Line
Enums are a powerful yet underutilized feature in Java. By incorporating enums into your state management strategy, you can create clearer, more maintainable code. The benefits of type safety, readability, and centralized logic make enums an excellent choice for managing state in your applications.
Additionally, with some creativity, you can extend their functionality to suit your needs further. Whether you are managing user roles, traffic lights, or even game states, enums can simplify your implementation and improve your code quality.
Remember, when done with proper practice and consideration, enums can significantly streamline how your application handles state. For more information on Java enums, you can check the official Java documentation.
Start embracing enums for state management in your next Java project, and witness how they transform your coding experience!
Checkout our other articles