Slicing Through Complexity: Mastering Guava's Strings
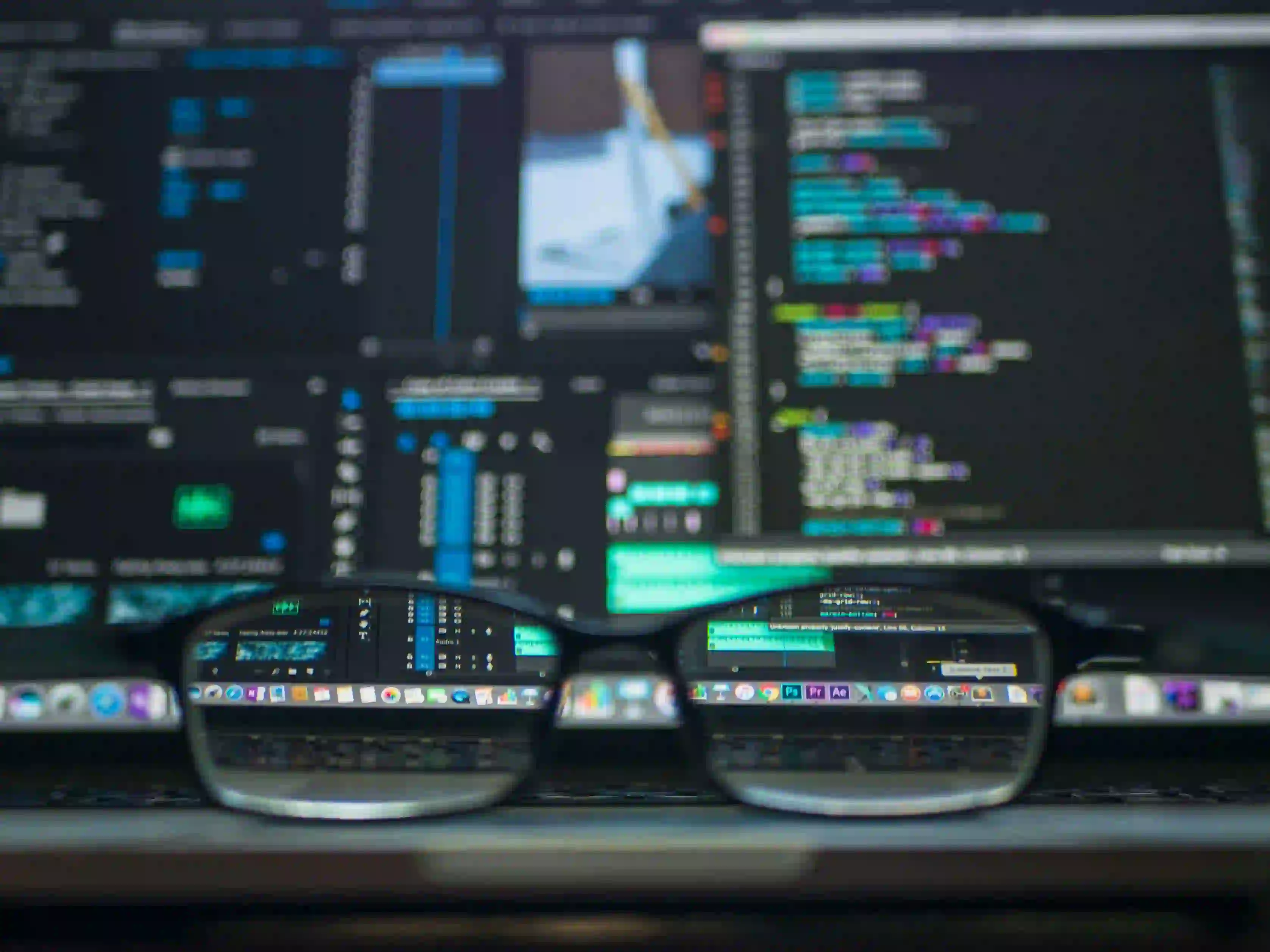
Slicing Through Complexity: Mastering Guava's Strings
Diving Into the Subject
String manipulation is a fundamental aspect of Java programming, and it plays a vital role in various applications, from data processing to user interface interactions. However, Java's native String class has limitations and complexities that can make string operations cumbersome and less intuitive. Fortunately, Google's Guava library provides a robust and elegant solution in the form of its Strings utility class. By leveraging Guava's Strings, developers can streamline string manipulations and tackle common challenges with ease.
Understanding Guava and Its Installation
Guava, developed by Google, is an open-source Java library that provides a rich set of utilities and helper functions to address various programming challenges. While it offers diverse functionalities, its Strings utility class stands out for simplifying string operations, handling null values, and providing convenient string-related methods beyond those offered by Java's native String class.
Adding Guava to Your Project
To incorporate Guava into a Java project, developers can use either Maven or Gradle as their build tools.
Maven Dependency Snippet:
<dependency>
<groupId>com.google.guava</groupId>
<artifactId>guava</artifactId>
<version>31.0.1-jre</version>
</dependency>
Gradle Dependency Snippet:
implementation 'com.google.guava:guava:31.0.1-jre'
Once the dependency has been added, developers can take advantage of the powerful features provided by Guava's Strings class. For deeper insights into Guava and its various utilities, readers can visit the Guava GitHub page and explore its official documentation.
Diving Into Guava's String Utilities
Guava's Strings class offers a plethora of methods that simplify common string manipulation tasks, including handling null values and padding strings. Let's delve into some of the most useful methods and explore practical examples of their usage.
Converting Between Null and Empty Strings
Converting null strings to empty strings and vice versa is a frequent requirement in many applications. Guava's nullToEmpty
and emptyToNull
methods provide a clean and concise way to handle such conversions.
String text = null;
String safeText = Strings.nullToEmpty(text); // Converts null to an empty string
System.out.println(safeText.equals("")); // true
In the above example, nullToEmpty
effortlessly transforms a null string into an empty string, facilitating smoother string manipulations and avoiding potential null pointer exceptions.
Checking for Null or Empty Strings
Validating strings for null or empty values is a common task in application logic. Guava's isNullOrEmpty
method simplifies this process and offers a more expressive alternative to Java's String.isEmpty()
or manual null checks.
String userName = getUserInput();
if (Strings.isNullOrEmpty(userName)) {
System.out.println("User name is required.");
}
By employing isNullOrEmpty
, developers can enhance the readability of their code and effectively handle scenarios where a user-entered username may be absent or empty.
Padding Strings for Alignment
In scenarios that involve text alignment, such as generating reports or formatting console-based outputs, padding strings becomes crucial. Guava's padStart
and padEnd
methods enable seamless string padding to ensure consistent text alignment.
String item = "Apple";
String paddedItem = Strings.padEnd(item, 10, ' ');
System.out.println(paddedItem + ": $1.99"); // Ensures alignment in a list of items
Here, padEnd
ensures that the string "Apple" is padded with spaces to achieve a total length of 10 characters, facilitating uniform text alignment in the output.
Common Use Cases and Best Practices
Guava's Strings utility class finds practical applications in various scenarios, offering a more streamlined approach to string manipulation compared to Java's native methods. Some common use cases and best practices include:
-
Log Formatting: When crafting log messages, Guava's string utilities can simplify the process of constructing informative and structured log entries, making it easier to analyze and troubleshoot issues.
-
User Input Normalization: Validating and normalizing user input strings, such as trimming leading and trailing spaces or handling null inputs, can be efficiently managed using Guava's methods, contributing to a more robust input processing logic.
-
Data Display in UIs or Reports: For displaying data in user interfaces or reports, Guava's string utilities aid in aligning and formatting text, ensuring a polished and professional presentation of information.
It's important to note that while Guava's Strings class provides powerful utilities, developers should consider the specific needs of their projects and the trade-offs associated with introducing third-party dependencies. There may be instances where Java's native String methods suffice, and incorporating Guava should be evaluated based on the overall benefits it brings to the codebase.
The Bottom Line
In conclusion, Guava's Strings utility class serves as a valuable asset for Java developers grappling with the complexities of string manipulation. By offering a comprehensive set of methods for handling null values, checking for string emptiness, and padding strings, Guava simplifies common string-related challenges and enhances code readability. Incorporating Guava into Java projects can efficiently streamline string operations and significantly improve the developer experience.
For further exploration of Guava's capabilities and other utilities beyond Strings, readers are encouraged to delve into the official documentation provided by Google. By leveraging Guava's features, developers can empower their Java applications to slice through complexity, master string manipulation, and elevate the quality of their code.